mirror of
https://gitlab.gnome.org/GNOME/gimp
synced 2024-10-21 20:12:30 +00:00
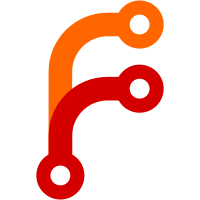
2001-07-24 Michael Natterer <mitch@gimp.org> Port to glib/gtk+ 2.0 episode I (every segfault has it's beginning) * configure.in: require glib/gtk+ >= 1.3.7, commented out the gtkxmhtml stuff. From now on, you will need glib, pango, atk and gtk+ HEAD from CVS to hack or use GIMP HEAD. Beware, it crashes randomly :) * app/core/Makefile.am * app/core/gimpmarshal.list: new file plus rules to generate gimpmarshal.[ch] from it. * app/core/* * app/tools/* * app/widgets/* * libgimpwidgets/*: started to use the glib object system. All core/ objects are still gtk objects however. All signals are created using g_signal_new(). There are many gtk+ artefacts left. Finally, we will _not_ use the gtk_signal_foo() wrappers and friends any more. * app/colormaps.c * app/devices.[ch] * app/disp_callbacks.c * app/errorconsole.c * app/file-save.[ch] * app/interface.c * app/module_db.c * app/nav_window.c * app/ops_buttons.c * app/scroll.c * app/user_install.c * app/gui/about-dialog.c * app/gui/brush-editor.c * app/gui/brushes-commands.c * app/gui/color-notebook.c * app/gui/colormap-dialog.c * app/gui/dialogs-commands.c * app/gui/dialogs-constructors.c * app/gui/file-commands.c * app/gui/file-dialog-utils.c * app/gui/file-new-dialog.c * app/gui/file-open-dialog.[ch] * app/gui/file-save-dialog.c * app/gui/gradient-editor.c * app/gui/gradients-commands.c * app/gui/image-commands.c * app/gui/info-dialog.[ch] * app/gui/layer-select.c * app/gui/layers-commands.c * app/gui/menus.c * app/gui/offset-dialog.c * app/gui/palette-editor.c * app/gui/palettes-commands.c * app/gui/patterns-commands.c * app/gui/preferences-dialog.c * app/gui/resize-dialog.[ch] * app/gui/splash.c * app/gui/tips-dialog.c * app/gui/tool-options-dialog.c * app/gui/toolbox.c * app/gui/tools-commands.c * libgimp/gimpbrushmenu.c * libgimp/gimpmenu.c * libgimp/gimppatternmenu.c * libgimp/gimpui.c * libgimpbase/gimpenv.c: tons and tons of changes like "const gchar*", switch from GdkDeviceInfo to GdkDevice (very incomplete and currently disables), lots of s/gtk_signal/g_signal/, removal/replacement of deprecated stuff, s/GtkSignalFunc/GCallback/ and lots of small changes and fixes while I was on it, zillions of warnings left... * modules/Makefile.am: disabled the water color selector temporarily (XInput issues). * plug-ins/Makefile.am * plug-ins/common/.cvsignore * plug-ins/common/Makefile.am * plug-ins/common/plugin-defs.pl: simply excluded all plug-ins which did not build (including Script-Fu). They are trivial to fix.
215 lines
6.5 KiB
C
215 lines
6.5 KiB
C
/* GTK - The GIMP Toolkit
|
|
* Copyright (C) 1995-1997 Peter Mattis, Spencer Kimball and Josh MacDonald
|
|
*
|
|
* GtkHWrapBox: Horizontal wrapping box widget
|
|
* Copyright (C) 1999 Tim Janik
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Library General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Library General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Library General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#include <gtk/gtk.h>
|
|
|
|
#include "gimpconstrainedhwrapbox.h"
|
|
|
|
|
|
static void gimp_constrained_hwrap_box_class_init (GimpConstrainedHWrapBoxClass *klass);
|
|
static void gimp_constrained_hwrap_box_init (GimpConstrainedHWrapBox *hwbox);
|
|
static void gimp_constrained_hwrap_box_size_request (GtkWidget *widget,
|
|
GtkRequisition *requisition);
|
|
static void gimp_constrained_hwrap_box_size_allocate (GtkWidget *widget,
|
|
GtkAllocation *allocation);
|
|
|
|
|
|
static GtkHWrapBoxClass *parent_class = NULL;
|
|
|
|
|
|
GtkType
|
|
gimp_constrained_hwrap_box_get_type (void)
|
|
{
|
|
static GtkType constrained_hwrap_box_type = 0;
|
|
|
|
if (! constrained_hwrap_box_type)
|
|
{
|
|
static const GtkTypeInfo constrained_hwrap_box_info =
|
|
{
|
|
"GimpConstrainedHWrapBox",
|
|
sizeof (GimpConstrainedHWrapBox),
|
|
sizeof (GimpConstrainedHWrapBoxClass),
|
|
(GtkClassInitFunc) gimp_constrained_hwrap_box_class_init,
|
|
(GtkObjectInitFunc) gimp_constrained_hwrap_box_init,
|
|
/* reserved_1 */ NULL,
|
|
/* reserved_2 */ NULL,
|
|
(GtkClassInitFunc) NULL,
|
|
};
|
|
|
|
constrained_hwrap_box_type = gtk_type_unique (GTK_TYPE_HWRAP_BOX,
|
|
&constrained_hwrap_box_info);
|
|
}
|
|
|
|
return constrained_hwrap_box_type;
|
|
}
|
|
|
|
static void
|
|
gimp_constrained_hwrap_box_class_init (GimpConstrainedHWrapBoxClass *class)
|
|
{
|
|
GtkObjectClass *object_class;
|
|
GtkWidgetClass *widget_class;
|
|
|
|
object_class = GTK_OBJECT_CLASS (class);
|
|
widget_class = GTK_WIDGET_CLASS (class);
|
|
|
|
parent_class = gtk_type_class (GTK_TYPE_HWRAP_BOX);
|
|
|
|
widget_class->size_request = gimp_constrained_hwrap_box_size_request;
|
|
widget_class->size_allocate = gimp_constrained_hwrap_box_size_allocate;
|
|
}
|
|
|
|
static void
|
|
gimp_constrained_hwrap_box_init (GimpConstrainedHWrapBox *hwbox)
|
|
{
|
|
hwbox->rows = 1;
|
|
hwbox->columns = 1;
|
|
}
|
|
|
|
GtkWidget*
|
|
gimp_constrained_hwrap_box_new (gboolean homogeneous)
|
|
{
|
|
GimpConstrainedHWrapBox *hwbox;
|
|
|
|
hwbox = GIMP_CONSTRAINED_HWRAP_BOX (gtk_widget_new (GIMP_TYPE_CONSTRAINED_HWRAP_BOX, NULL));
|
|
|
|
GTK_WRAP_BOX (hwbox)->homogeneous = homogeneous ? TRUE : FALSE;
|
|
|
|
return GTK_WIDGET (hwbox);
|
|
}
|
|
|
|
static void
|
|
gimp_constrained_hwrap_box_size_request (GtkWidget *widget,
|
|
GtkRequisition *requisition)
|
|
{
|
|
GtkWrapBox *wbox = GTK_WRAP_BOX (widget);
|
|
|
|
g_return_if_fail (requisition != NULL);
|
|
|
|
if (widget->parent &&
|
|
GTK_IS_VIEWPORT (widget->parent) &&
|
|
widget->parent->parent &&
|
|
GTK_IS_SCROLLED_WINDOW (widget->parent->parent) &&
|
|
wbox->children &&
|
|
wbox->children->widget)
|
|
{
|
|
GtkWidget *scrolled_win;
|
|
gint child_width;
|
|
gint child_height;
|
|
gint viewport_width;
|
|
gint columns;
|
|
gint rows;
|
|
|
|
scrolled_win = widget->parent->parent;
|
|
|
|
child_width = wbox->children->widget->requisition.width;
|
|
child_height = wbox->children->widget->requisition.height;
|
|
|
|
viewport_width =
|
|
(scrolled_win->allocation.width -
|
|
GTK_SCROLLED_WINDOW (scrolled_win)->vscrollbar->allocation.width -
|
|
GTK_SCROLLED_WINDOW_GET_CLASS (scrolled_win)->scrollbar_spacing -
|
|
scrolled_win->style->xthickness * 2);
|
|
|
|
columns =
|
|
(viewport_width + wbox->hspacing) / (child_width + wbox->hspacing);
|
|
|
|
columns = MAX (1, columns);
|
|
|
|
requisition->width = (child_width + wbox->hspacing) * columns;
|
|
|
|
rows = wbox->n_children / columns;
|
|
|
|
if (rows * columns < wbox->n_children)
|
|
rows++;
|
|
|
|
requisition->height = (child_height + wbox->vspacing) * rows;
|
|
|
|
GIMP_CONSTRAINED_HWRAP_BOX (wbox)->columns = columns;
|
|
GIMP_CONSTRAINED_HWRAP_BOX (wbox)->rows = rows;
|
|
}
|
|
else if (GTK_WIDGET_CLASS (parent_class)->size_request)
|
|
{
|
|
GTK_WIDGET_CLASS (parent_class)->size_request (widget, requisition);
|
|
|
|
GIMP_CONSTRAINED_HWRAP_BOX (wbox)->columns = 1;
|
|
GIMP_CONSTRAINED_HWRAP_BOX (wbox)->rows = 1;
|
|
}
|
|
}
|
|
|
|
static void
|
|
gimp_constrained_hwrap_box_size_allocate (GtkWidget *widget,
|
|
GtkAllocation *allocation)
|
|
{
|
|
GtkWrapBox *wbox = GTK_WRAP_BOX (widget);
|
|
|
|
g_return_if_fail (allocation != NULL);
|
|
|
|
if (widget->parent &&
|
|
GTK_IS_VIEWPORT (widget->parent) &&
|
|
widget->parent->parent &&
|
|
GTK_IS_SCROLLED_WINDOW (widget->parent->parent) &&
|
|
wbox->children &&
|
|
wbox->children->widget)
|
|
{
|
|
GtkWidget *scrolled_win;
|
|
gint child_width;
|
|
gint child_height;
|
|
gint viewport_width;
|
|
gint columns;
|
|
gint rows;
|
|
|
|
scrolled_win = widget->parent->parent;
|
|
|
|
child_width = wbox->children->widget->requisition.width;
|
|
child_height = wbox->children->widget->requisition.height;
|
|
|
|
viewport_width =
|
|
(scrolled_win->allocation.width -
|
|
GTK_SCROLLED_WINDOW (scrolled_win)->vscrollbar->allocation.width -
|
|
GTK_SCROLLED_WINDOW_GET_CLASS (scrolled_win)->scrollbar_spacing -
|
|
scrolled_win->style->xthickness * 2);
|
|
|
|
allocation->width = viewport_width;
|
|
|
|
columns =
|
|
(viewport_width + wbox->hspacing) / (child_width + wbox->hspacing);
|
|
|
|
columns = MAX (1, columns);
|
|
|
|
rows = wbox->n_children / columns;
|
|
|
|
if (rows * columns < wbox->n_children)
|
|
rows++;
|
|
|
|
GIMP_CONSTRAINED_HWRAP_BOX (wbox)->columns = columns;
|
|
GIMP_CONSTRAINED_HWRAP_BOX (wbox)->rows = rows;
|
|
}
|
|
else
|
|
{
|
|
GIMP_CONSTRAINED_HWRAP_BOX (wbox)->columns = 1;
|
|
GIMP_CONSTRAINED_HWRAP_BOX (wbox)->rows = 1;
|
|
}
|
|
|
|
if (GTK_WIDGET_CLASS (parent_class)->size_allocate)
|
|
GTK_WIDGET_CLASS (parent_class)->size_allocate (widget, allocation);
|
|
}
|