mirror of
https://gitlab.gnome.org/GNOME/gimp
synced 2024-10-21 20:12:30 +00:00
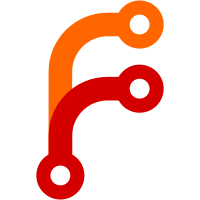
2005-07-25 Michael Natterer <mitch@gimp.org> Some DND fixes / cleanup: * app/widgets/widgets-enums.h: renamed GIMP_DND_TYPE_TOOL to GIMP_DND_TYPE_TOOL_INFO. * app/widgets/gimpselectiondata.[ch]: s/tool/tool_info/g. Moved private functions to the end of the file. Include GIMP's PID in all GtkSelectionData strings which are used to pass around stuff by reference. For things which are referenced by name, also encode the object's address in the GtkSelectionData so having a brush called "Standard" or a named buffer called "Global Buffer" will work together with DND. * app/widgets/gimpdnd.[ch]: s/tool/tool_info/g. Renamed gimp_dnd_get_data_data() to gimp_dnd_get_object_data() since it's not limited to GimpData objects. Follow above selection data API changes. Cleanup. * libgimp/gimpbrushmenu.c * libgimp/gimpdrawablecombobox.c * libgimp/gimpfontselectbutton.c * libgimp/gimpgradientmenu.c * libgimp/gimpimagecombobox.c * libgimp/gimppalettemenu.c * libgimp/gimppatternmenu.c: follow GtkSelectionData format change and check the dropped things' PID against the return value of gimp_getpid().
260 lines
12 KiB
C
260 lines
12 KiB
C
/* The GIMP -- an image manipulation program
|
|
* Copyright (C) 1995-1997 Spencer Kimball and Peter Mattis
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef __GIMP_DND_H__
|
|
#define __GIMP_DND_H__
|
|
|
|
|
|
#define GIMP_TARGET_URI_LIST \
|
|
{ "text/uri-list", 0, GIMP_DND_TYPE_URI_LIST }
|
|
|
|
#define GIMP_TARGET_TEXT_PLAIN \
|
|
{ "text/plain", 0, GIMP_DND_TYPE_TEXT_PLAIN }
|
|
|
|
#define GIMP_TARGET_NETSCAPE_URL \
|
|
{ "_NETSCAPE_URL", 0, GIMP_DND_TYPE_NETSCAPE_URL }
|
|
|
|
#define GIMP_TARGET_XDS \
|
|
{ "XdndDirectSave0", 0, GIMP_DND_TYPE_XDS }
|
|
|
|
#define GIMP_TARGET_COLOR \
|
|
{ "application/x-color", 0, GIMP_DND_TYPE_COLOR }
|
|
|
|
#define GIMP_TARGET_SVG \
|
|
{ "image/svg", 0, GIMP_DND_TYPE_SVG }
|
|
|
|
#define GIMP_TARGET_SVG_XML \
|
|
{ "image/svg+xml", 0, GIMP_DND_TYPE_SVG_XML }
|
|
|
|
/* just here for documentation purposes, the actual list of targets
|
|
* is created dynamically from available GdkPixbuf loaders
|
|
*/
|
|
#define GIMP_TARGET_PIXBUF \
|
|
{ NULL, 0, GIMP_DND_TYPE_PIXBUF }
|
|
|
|
#define GIMP_TARGET_IMAGE \
|
|
{ "application/x-gimp-image-id", GTK_TARGET_SAME_APP, GIMP_DND_TYPE_IMAGE }
|
|
|
|
#define GIMP_TARGET_COMPONENT \
|
|
{ "application/x-gimp-component", GTK_TARGET_SAME_APP, GIMP_DND_TYPE_COMPONENT }
|
|
|
|
#define GIMP_TARGET_LAYER \
|
|
{ "application/x-gimp-layer-id", GTK_TARGET_SAME_APP, GIMP_DND_TYPE_LAYER }
|
|
|
|
#define GIMP_TARGET_CHANNEL \
|
|
{ "application/x-gimp-channel-id", GTK_TARGET_SAME_APP, GIMP_DND_TYPE_CHANNEL }
|
|
|
|
#define GIMP_TARGET_LAYER_MASK \
|
|
{ "application/x-gimp-layer-mask-id", GTK_TARGET_SAME_APP, GIMP_DND_TYPE_LAYER_MASK }
|
|
|
|
#define GIMP_TARGET_VECTORS \
|
|
{ "application/x-gimp-vectors-id", GTK_TARGET_SAME_APP, GIMP_DND_TYPE_VECTORS }
|
|
|
|
#define GIMP_TARGET_BRUSH \
|
|
{ "application/x-gimp-brush-name", 0, GIMP_DND_TYPE_BRUSH }
|
|
|
|
#define GIMP_TARGET_PATTERN \
|
|
{ "application/x-gimp-pattern-name", 0, GIMP_DND_TYPE_PATTERN }
|
|
|
|
#define GIMP_TARGET_GRADIENT \
|
|
{ "application/x-gimp-gradient-name", 0, GIMP_DND_TYPE_GRADIENT }
|
|
|
|
#define GIMP_TARGET_PALETTE \
|
|
{ "application/x-gimp-palette-name", 0, GIMP_DND_TYPE_PALETTE }
|
|
|
|
#define GIMP_TARGET_FONT \
|
|
{ "application/x-gimp-font-name", 0, GIMP_DND_TYPE_FONT }
|
|
|
|
#define GIMP_TARGET_BUFFER \
|
|
{ "application/x-gimp-buffer-name", GTK_TARGET_SAME_APP, GIMP_DND_TYPE_BUFFER }
|
|
|
|
#define GIMP_TARGET_IMAGEFILE \
|
|
{ "application/x-gimp-imagefile-name", GTK_TARGET_SAME_APP, GIMP_DND_TYPE_IMAGEFILE }
|
|
|
|
#define GIMP_TARGET_TEMPLATE \
|
|
{ "application/x-gimp-template-name", GTK_TARGET_SAME_APP, GIMP_DND_TYPE_TEMPLATE }
|
|
|
|
#define GIMP_TARGET_TOOL_INFO \
|
|
{ "application/x-gimp-tool-info-name", GTK_TARGET_SAME_APP, GIMP_DND_TYPE_TOOL_INFO }
|
|
|
|
#define GIMP_TARGET_DIALOG \
|
|
{ "application/x-gimp-dialog", GTK_TARGET_SAME_APP, GIMP_DND_TYPE_DIALOG }
|
|
|
|
|
|
/* dnd initialization */
|
|
|
|
void gimp_dnd_init (Gimp *gimp);
|
|
|
|
|
|
/* uri list dnd functions */
|
|
|
|
typedef GList * (* GimpDndDragUriListFunc) (GtkWidget *widget,
|
|
gpointer data);
|
|
typedef void (* GimpDndDropUriListFunc) (GtkWidget *widget,
|
|
gint x,
|
|
gint y,
|
|
GList *uri_list,
|
|
gpointer data);
|
|
|
|
void gimp_dnd_uri_list_source_add (GtkWidget *widget,
|
|
GimpDndDragUriListFunc get_uri_list_func,
|
|
gpointer data);
|
|
void gimp_dnd_uri_list_source_remove (GtkWidget *widget);
|
|
|
|
void gimp_dnd_uri_list_dest_add (GtkWidget *widget,
|
|
GimpDndDropUriListFunc set_uri_list_func,
|
|
gpointer data);
|
|
void gimp_dnd_uri_list_dest_remove (GtkWidget *widget);
|
|
|
|
|
|
/* color dnd functions */
|
|
|
|
typedef void (* GimpDndDragColorFunc) (GtkWidget *widget,
|
|
GimpRGB *color,
|
|
gpointer data);
|
|
typedef void (* GimpDndDropColorFunc) (GtkWidget *widget,
|
|
gint x,
|
|
gint y,
|
|
const GimpRGB *color,
|
|
gpointer data);
|
|
|
|
void gimp_dnd_color_source_add (GtkWidget *widget,
|
|
GimpDndDragColorFunc get_color_func,
|
|
gpointer data);
|
|
void gimp_dnd_color_source_remove (GtkWidget *widget);
|
|
|
|
void gimp_dnd_color_dest_add (GtkWidget *widget,
|
|
GimpDndDropColorFunc set_color_func,
|
|
gpointer data);
|
|
void gimp_dnd_color_dest_remove (GtkWidget *widget);
|
|
|
|
|
|
/* stream dnd functions */
|
|
|
|
typedef guchar * (* GimpDndDragStreamFunc) (GtkWidget *widget,
|
|
gsize *stream_len,
|
|
gpointer data);
|
|
typedef void (* GimpDndDropStreamFunc) (GtkWidget *widget,
|
|
gint x,
|
|
gint y,
|
|
const guchar *stream,
|
|
gsize stream_len,
|
|
gpointer data);
|
|
|
|
void gimp_dnd_svg_source_add (GtkWidget *widget,
|
|
GimpDndDragStreamFunc get_svg_func,
|
|
gpointer data);
|
|
void gimp_dnd_svg_source_remove (GtkWidget *widget);
|
|
|
|
void gimp_dnd_svg_dest_add (GtkWidget *widget,
|
|
GimpDndDropStreamFunc set_svg_func,
|
|
gpointer data);
|
|
void gimp_dnd_svg_dest_remove (GtkWidget *widget);
|
|
|
|
|
|
/* pixbuf dnd functions */
|
|
|
|
typedef GdkPixbuf * (* GimpDndDragPixbufFunc) (GtkWidget *widget,
|
|
gpointer data);
|
|
typedef void (* GimpDndDropPixbufFunc) (GtkWidget *widget,
|
|
gint x,
|
|
gint y,
|
|
GdkPixbuf *pixbuf,
|
|
gpointer data);
|
|
|
|
void gimp_dnd_pixbuf_source_add (GtkWidget *widget,
|
|
GimpDndDragPixbufFunc get_pixbuf_func,
|
|
gpointer data);
|
|
void gimp_dnd_pixbuf_source_remove (GtkWidget *widget);
|
|
|
|
void gimp_dnd_pixbuf_dest_add (GtkWidget *widget,
|
|
GimpDndDropPixbufFunc set_pixbuf_func,
|
|
gpointer data);
|
|
void gimp_dnd_pixbuf_dest_remove (GtkWidget *widget);
|
|
|
|
|
|
/* component dnd functions */
|
|
|
|
typedef GimpImage * (* GimpDndDragComponentFunc) (GtkWidget *widget,
|
|
GimpChannelType *channel,
|
|
gpointer data);
|
|
typedef void (* GimpDndDropComponentFunc) (GtkWidget *widget,
|
|
gint x,
|
|
gint y,
|
|
GimpImage *image,
|
|
GimpChannelType channel,
|
|
gpointer data);
|
|
|
|
void gimp_dnd_component_source_add (GtkWidget *widget,
|
|
GimpDndDragComponentFunc get_comp_func,
|
|
gpointer data);
|
|
void gimp_dnd_component_source_remove (GtkWidget *widget);
|
|
|
|
void gimp_dnd_component_dest_add (GtkWidget *widget,
|
|
GimpDndDropComponentFunc set_comp_func,
|
|
gpointer data);
|
|
void gimp_dnd_component_dest_remove (GtkWidget *widget);
|
|
|
|
|
|
/* GimpViewable (by GType) dnd functions */
|
|
|
|
typedef GimpViewable * (* GimpDndDragViewableFunc) (GtkWidget *widget,
|
|
gpointer data);
|
|
typedef void (* GimpDndDropViewableFunc) (GtkWidget *widget,
|
|
gint x,
|
|
gint y,
|
|
GimpViewable *viewable,
|
|
gpointer data);
|
|
|
|
|
|
gboolean gimp_dnd_drag_source_set_by_type (GtkWidget *widget,
|
|
GdkModifierType start_button_mask,
|
|
GType type,
|
|
GdkDragAction actions);
|
|
gboolean gimp_dnd_viewable_source_add (GtkWidget *widget,
|
|
GType type,
|
|
GimpDndDragViewableFunc get_viewable_func,
|
|
gpointer data);
|
|
gboolean gimp_dnd_viewable_source_remove (GtkWidget *widget,
|
|
GType type);
|
|
|
|
gboolean gimp_dnd_drag_dest_set_by_type (GtkWidget *widget,
|
|
GtkDestDefaults flags,
|
|
GType type,
|
|
GdkDragAction actions);
|
|
|
|
gboolean gimp_dnd_viewable_dest_add (GtkWidget *widget,
|
|
GType type,
|
|
GimpDndDropViewableFunc set_viewable_func,
|
|
gpointer data);
|
|
gboolean gimp_dnd_viewable_dest_remove (GtkWidget *widget,
|
|
GType type);
|
|
|
|
GimpViewable * gimp_dnd_get_drag_data (GtkWidget *widget);
|
|
|
|
|
|
/* Direct Save Protocol (XDS) */
|
|
|
|
void gimp_dnd_xds_source_add (GtkWidget *widget,
|
|
GimpDndDragViewableFunc get_image_func,
|
|
gpointer data);
|
|
void gimp_dnd_xds_source_remove (GtkWidget *widget);
|
|
|
|
|
|
#endif /* __GIMP_DND_H__ */
|