mirror of
https://gitlab.gnome.org/GNOME/gimp
synced 2024-10-22 20:41:43 +00:00
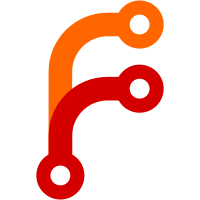
* app/composite/gimp-composite-dispatch.[ch]: retired and removed. * app/composite/Makefile.am: The 'test' target now builds and runs all the tests in TESTS Fixed typos of mismatched parenthesis in 'regenerate' target * app/composite/Makefile.am: TESTS now include ss2 and 3dnow instruction sets. * app/composite/make-gimp-composite-dispatch.py: retired and removed. * app/composite/gimp-composite.c: call the installers of all the various instruction sets supplied by x86 type processors. * app/base/base.c (base_init): Call gimp_composite_init() *before* paint_funcs_setup() (preparation for retiring app/paint-funcs/paint-funcs.c) * app/composite/gimp-composite-sse2.[ch] * app/composite/gimp-composite-3dnow.[ch] * app/composite/gimp-composite-sse2-installer.c * app/composite/gimp-composite-3dnow-installer.c: Added * app/composite/make-installer.py: generate regression test programmes that take the command line arguments --iterations and --n-pixels for the number of iterations of a set of tests, and the number of pixels to use in each test, respectively. Conditionally compile tests in order to build and run tests on machines that can actually perform the tests. Failing a regression test now causes remaining tests to be skipped and a non-zero exit code by the programme.
88 lines
2 KiB
C
88 lines
2 KiB
C
#include "config.h"
|
|
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
|
|
#include <sys/time.h>
|
|
|
|
#include <glib-object.h>
|
|
|
|
#include "base/base-types.h"
|
|
|
|
#include "gimp-composite.h"
|
|
#include "gimp-composite-regression.h"
|
|
#include "gimp-composite-util.h"
|
|
#include "gimp-composite-generic.h"
|
|
|
|
int
|
|
gimp_composite_regression(int iterations, int n_pixels)
|
|
{
|
|
GimpCompositeContext generic_ctx;
|
|
GimpCompositeContext special_ctx;
|
|
double ft0;
|
|
double ft1;
|
|
gimp_rgba8_t *rgba8D1;
|
|
gimp_rgba8_t *rgba8D2;
|
|
gimp_rgba8_t *rgba8A;
|
|
gimp_rgba8_t *rgba8B;
|
|
gimp_rgba8_t *rgba8M;
|
|
gimp_va8_t *va8A;
|
|
gimp_va8_t *va8B;
|
|
gimp_va8_t *va8M;
|
|
gimp_va8_t *va8D1;
|
|
gimp_va8_t *va8D2;
|
|
int i;
|
|
|
|
rgba8A = (gimp_rgba8_t *) calloc(sizeof(gimp_rgba8_t), n_pixels+1);
|
|
rgba8B = (gimp_rgba8_t *) calloc(sizeof(gimp_rgba8_t), n_pixels+1);
|
|
rgba8M = (gimp_rgba8_t *) calloc(sizeof(gimp_rgba8_t), n_pixels+1);
|
|
rgba8D1 = (gimp_rgba8_t *) calloc(sizeof(gimp_rgba8_t), n_pixels+1);
|
|
rgba8D2 = (gimp_rgba8_t *) calloc(sizeof(gimp_rgba8_t), n_pixels+1);
|
|
va8A = (gimp_va8_t *) calloc(sizeof(gimp_va8_t), n_pixels+1);
|
|
va8B = (gimp_va8_t *) calloc(sizeof(gimp_va8_t), n_pixels+1);
|
|
va8M = (gimp_va8_t *) calloc(sizeof(gimp_va8_t), n_pixels+1);
|
|
va8D1 = (gimp_va8_t *) calloc(sizeof(gimp_va8_t), n_pixels+1);
|
|
va8D2 = (gimp_va8_t *) calloc(sizeof(gimp_va8_t), n_pixels+1);
|
|
|
|
for (i = 0; i < n_pixels; i++) {
|
|
rgba8A[i].r = 255-i;
|
|
rgba8A[i].g = 255-i;
|
|
rgba8A[i].b = 255-i;
|
|
rgba8A[i].a = 255-i;
|
|
|
|
rgba8B[i].r = i;
|
|
rgba8B[i].g = i;
|
|
rgba8B[i].b = i;
|
|
rgba8B[i].a = i;
|
|
|
|
rgba8M[i].r = i;
|
|
rgba8M[i].g = i;
|
|
rgba8M[i].b = i;
|
|
rgba8M[i].a = i;
|
|
|
|
va8A[i].v = i;
|
|
va8A[i].a = 255-i;
|
|
va8B[i].v = i;
|
|
va8B[i].a = i;
|
|
va8M[i].v = i;
|
|
va8M[i].a = i;
|
|
}
|
|
|
|
return (0);
|
|
}
|
|
|
|
int
|
|
main(int argc, char *argv[])
|
|
{
|
|
int iterations;
|
|
int n_pixels;
|
|
|
|
srand(314159);
|
|
|
|
iterations = 1;
|
|
n_pixels = 256*256;
|
|
|
|
return (gimp_composite_regression(iterations, n_pixels));
|
|
}
|