mirror of
https://gitlab.gnome.org/GNOME/gimp
synced 2024-10-21 12:02:32 +00:00
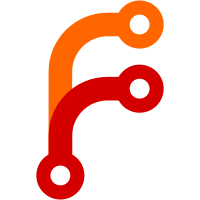
2007-04-25 Michael Natterer <mitch@gimp.org> * app/core/gimpparamspecs.[ch] (struct GimpParamSpecString) (gimp_param_spec_string): added "gboolean non_empty" to require the string being non-empty. Changed validation accordingly. Also fixed validation for static strings (we were happily freeing and modifying them before). * app/xcf/xcf.c: filenames should be non-empty. * app/pdb/gimp-pdb-compat.c: compat strings shouldn't. * tools/pdbgen/app.pl: add support for $arg->{non_empty} and changed generation of calls to gimp_param_spec_string(). * tools/pdbgen/pdb/brush_select.pdb * tools/pdbgen/pdb/edit.pdb * tools/pdbgen/pdb/vectors.pdb * tools/pdbgen/pdb/plug_in.pdb * tools/pdbgen/pdb/gradient.pdb * tools/pdbgen/pdb/palette_select.pdb * tools/pdbgen/pdb/palette.pdb * tools/pdbgen/pdb/fileops.pdb * tools/pdbgen/pdb/progress.pdb * tools/pdbgen/pdb/procedural_db.pdb * tools/pdbgen/pdb/font_select.pdb * tools/pdbgen/pdb/pattern_select.pdb * tools/pdbgen/pdb/unit.pdb * tools/pdbgen/pdb/brush.pdb * tools/pdbgen/pdb/gradient_select.pdb * tools/pdbgen/pdb/buffer.pdb: require non-empty strings for data object names, procedure names, unit strings, PDB data identifiers and buffer names. Removed some manual strlen() checks, all other places just got better error reporting for free (proper validation error instead of unspecific execution error). * app/pdb/*_cmds.c: regenerated. svn path=/trunk/; revision=22329
928 lines
40 KiB
C
928 lines
40 KiB
C
/* GIMP - The GNU Image Manipulation Program
|
|
* Copyright (C) 1995-2003 Spencer Kimball and Peter Mattis
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
/* NOTE: This file is auto-generated by pdbgen.pl. */
|
|
|
|
#include "config.h"
|
|
|
|
|
|
#include <glib-object.h>
|
|
|
|
#include "libgimpbase/gimpbase.h"
|
|
|
|
#include "pdb-types.h"
|
|
#include "gimppdb.h"
|
|
#include "gimpprocedure.h"
|
|
#include "core/gimpparamspecs.h"
|
|
|
|
#include "core/gimp-parasites.h"
|
|
#include "core/gimpdrawable.h"
|
|
#include "core/gimpimage.h"
|
|
#include "vectors/gimpvectors.h"
|
|
|
|
#include "internal_procs.h"
|
|
|
|
|
|
static GValueArray *
|
|
parasite_find_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GValueArray *return_vals;
|
|
const gchar *name;
|
|
GimpParasite *parasite = NULL;
|
|
|
|
name = g_value_get_string (&args->values[0]);
|
|
|
|
if (success)
|
|
{
|
|
parasite = gimp_parasite_copy (gimp_parasite_find (gimp, name));
|
|
|
|
if (! parasite)
|
|
success = FALSE;
|
|
}
|
|
|
|
return_vals = gimp_procedure_get_return_values (procedure, success);
|
|
|
|
if (success)
|
|
g_value_take_boxed (&return_vals->values[1], parasite);
|
|
|
|
return return_vals;
|
|
}
|
|
|
|
static GValueArray *
|
|
parasite_attach_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
const GimpParasite *parasite;
|
|
|
|
parasite = g_value_get_boxed (&args->values[0]);
|
|
|
|
if (success)
|
|
{
|
|
gimp_parasite_attach (gimp, parasite);
|
|
}
|
|
|
|
return gimp_procedure_get_return_values (procedure, success);
|
|
}
|
|
|
|
static GValueArray *
|
|
parasite_detach_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
const gchar *name;
|
|
|
|
name = g_value_get_string (&args->values[0]);
|
|
|
|
if (success)
|
|
{
|
|
gimp_parasite_detach (gimp, name);
|
|
}
|
|
|
|
return gimp_procedure_get_return_values (procedure, success);
|
|
}
|
|
|
|
static GValueArray *
|
|
parasite_list_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
GValueArray *return_vals;
|
|
gint32 num_parasites = 0;
|
|
gchar **parasites = NULL;
|
|
|
|
parasites = gimp_parasite_list (gimp, &num_parasites);
|
|
|
|
return_vals = gimp_procedure_get_return_values (procedure, TRUE);
|
|
|
|
g_value_set_int (&return_vals->values[1], num_parasites);
|
|
gimp_value_take_stringarray (&return_vals->values[2], parasites, num_parasites);
|
|
|
|
return return_vals;
|
|
}
|
|
|
|
static GValueArray *
|
|
image_parasite_find_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GValueArray *return_vals;
|
|
GimpImage *image;
|
|
const gchar *name;
|
|
GimpParasite *parasite = NULL;
|
|
|
|
image = gimp_value_get_image (&args->values[0], gimp);
|
|
name = g_value_get_string (&args->values[1]);
|
|
|
|
if (success)
|
|
{
|
|
parasite = gimp_parasite_copy (gimp_image_parasite_find (image, name));
|
|
|
|
if (! parasite)
|
|
success = FALSE;
|
|
}
|
|
|
|
return_vals = gimp_procedure_get_return_values (procedure, success);
|
|
|
|
if (success)
|
|
g_value_take_boxed (&return_vals->values[1], parasite);
|
|
|
|
return return_vals;
|
|
}
|
|
|
|
static GValueArray *
|
|
image_parasite_attach_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GimpImage *image;
|
|
const GimpParasite *parasite;
|
|
|
|
image = gimp_value_get_image (&args->values[0], gimp);
|
|
parasite = g_value_get_boxed (&args->values[1]);
|
|
|
|
if (success)
|
|
{
|
|
gimp_image_parasite_attach (image, parasite);
|
|
}
|
|
|
|
return gimp_procedure_get_return_values (procedure, success);
|
|
}
|
|
|
|
static GValueArray *
|
|
image_parasite_detach_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GimpImage *image;
|
|
const gchar *name;
|
|
|
|
image = gimp_value_get_image (&args->values[0], gimp);
|
|
name = g_value_get_string (&args->values[1]);
|
|
|
|
if (success)
|
|
{
|
|
gimp_image_parasite_detach (image, name);
|
|
}
|
|
|
|
return gimp_procedure_get_return_values (procedure, success);
|
|
}
|
|
|
|
static GValueArray *
|
|
image_parasite_list_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GValueArray *return_vals;
|
|
GimpImage *image;
|
|
gint32 num_parasites = 0;
|
|
gchar **parasites = NULL;
|
|
|
|
image = gimp_value_get_image (&args->values[0], gimp);
|
|
|
|
if (success)
|
|
{
|
|
parasites = gimp_image_parasite_list (image, &num_parasites);
|
|
}
|
|
|
|
return_vals = gimp_procedure_get_return_values (procedure, success);
|
|
|
|
if (success)
|
|
{
|
|
g_value_set_int (&return_vals->values[1], num_parasites);
|
|
gimp_value_take_stringarray (&return_vals->values[2], parasites, num_parasites);
|
|
}
|
|
|
|
return return_vals;
|
|
}
|
|
|
|
static GValueArray *
|
|
drawable_parasite_find_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GValueArray *return_vals;
|
|
GimpDrawable *drawable;
|
|
const gchar *name;
|
|
GimpParasite *parasite = NULL;
|
|
|
|
drawable = gimp_value_get_drawable (&args->values[0], gimp);
|
|
name = g_value_get_string (&args->values[1]);
|
|
|
|
if (success)
|
|
{
|
|
parasite = gimp_parasite_copy (gimp_item_parasite_find (GIMP_ITEM (drawable),
|
|
name));
|
|
|
|
if (! parasite)
|
|
success = FALSE;
|
|
}
|
|
|
|
return_vals = gimp_procedure_get_return_values (procedure, success);
|
|
|
|
if (success)
|
|
g_value_take_boxed (&return_vals->values[1], parasite);
|
|
|
|
return return_vals;
|
|
}
|
|
|
|
static GValueArray *
|
|
drawable_parasite_attach_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GimpDrawable *drawable;
|
|
const GimpParasite *parasite;
|
|
|
|
drawable = gimp_value_get_drawable (&args->values[0], gimp);
|
|
parasite = g_value_get_boxed (&args->values[1]);
|
|
|
|
if (success)
|
|
{
|
|
gimp_item_parasite_attach (GIMP_ITEM (drawable), parasite);
|
|
}
|
|
|
|
return gimp_procedure_get_return_values (procedure, success);
|
|
}
|
|
|
|
static GValueArray *
|
|
drawable_parasite_detach_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GimpDrawable *drawable;
|
|
const gchar *name;
|
|
|
|
drawable = gimp_value_get_drawable (&args->values[0], gimp);
|
|
name = g_value_get_string (&args->values[1]);
|
|
|
|
if (success)
|
|
{
|
|
gimp_item_parasite_detach (GIMP_ITEM (drawable), name);
|
|
}
|
|
|
|
return gimp_procedure_get_return_values (procedure, success);
|
|
}
|
|
|
|
static GValueArray *
|
|
drawable_parasite_list_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GValueArray *return_vals;
|
|
GimpDrawable *drawable;
|
|
gint32 num_parasites = 0;
|
|
gchar **parasites = NULL;
|
|
|
|
drawable = gimp_value_get_drawable (&args->values[0], gimp);
|
|
|
|
if (success)
|
|
{
|
|
parasites = gimp_item_parasite_list (GIMP_ITEM (drawable), &num_parasites);
|
|
}
|
|
|
|
return_vals = gimp_procedure_get_return_values (procedure, success);
|
|
|
|
if (success)
|
|
{
|
|
g_value_set_int (&return_vals->values[1], num_parasites);
|
|
gimp_value_take_stringarray (&return_vals->values[2], parasites, num_parasites);
|
|
}
|
|
|
|
return return_vals;
|
|
}
|
|
|
|
static GValueArray *
|
|
vectors_parasite_find_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GValueArray *return_vals;
|
|
GimpVectors *vectors;
|
|
const gchar *name;
|
|
GimpParasite *parasite = NULL;
|
|
|
|
vectors = gimp_value_get_vectors (&args->values[0], gimp);
|
|
name = g_value_get_string (&args->values[1]);
|
|
|
|
if (success)
|
|
{
|
|
parasite = gimp_parasite_copy (gimp_item_parasite_find (GIMP_ITEM (vectors),
|
|
name));
|
|
|
|
if (! parasite)
|
|
success = FALSE;
|
|
}
|
|
|
|
return_vals = gimp_procedure_get_return_values (procedure, success);
|
|
|
|
if (success)
|
|
g_value_take_boxed (&return_vals->values[1], parasite);
|
|
|
|
return return_vals;
|
|
}
|
|
|
|
static GValueArray *
|
|
vectors_parasite_attach_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GimpVectors *vectors;
|
|
const GimpParasite *parasite;
|
|
|
|
vectors = gimp_value_get_vectors (&args->values[0], gimp);
|
|
parasite = g_value_get_boxed (&args->values[1]);
|
|
|
|
if (success)
|
|
{
|
|
gimp_item_parasite_attach (GIMP_ITEM (vectors), parasite);
|
|
}
|
|
|
|
return gimp_procedure_get_return_values (procedure, success);
|
|
}
|
|
|
|
static GValueArray *
|
|
vectors_parasite_detach_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GimpVectors *vectors;
|
|
const gchar *name;
|
|
|
|
vectors = gimp_value_get_vectors (&args->values[0], gimp);
|
|
name = g_value_get_string (&args->values[1]);
|
|
|
|
if (success)
|
|
{
|
|
gimp_item_parasite_detach (GIMP_ITEM (vectors), name);
|
|
}
|
|
|
|
return gimp_procedure_get_return_values (procedure, success);
|
|
}
|
|
|
|
static GValueArray *
|
|
vectors_parasite_list_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
GValueArray *return_vals;
|
|
GimpVectors *vectors;
|
|
gint32 num_parasites = 0;
|
|
gchar **parasites = NULL;
|
|
|
|
vectors = gimp_value_get_vectors (&args->values[0], gimp);
|
|
|
|
if (success)
|
|
{
|
|
parasites = gimp_item_parasite_list (GIMP_ITEM (vectors), &num_parasites);
|
|
}
|
|
|
|
return_vals = gimp_procedure_get_return_values (procedure, success);
|
|
|
|
if (success)
|
|
{
|
|
g_value_set_int (&return_vals->values[1], num_parasites);
|
|
gimp_value_take_stringarray (&return_vals->values[2], parasites, num_parasites);
|
|
}
|
|
|
|
return return_vals;
|
|
}
|
|
|
|
void
|
|
register_parasite_procs (GimpPDB *pdb)
|
|
{
|
|
GimpProcedure *procedure;
|
|
|
|
/*
|
|
* gimp-parasite-find
|
|
*/
|
|
procedure = gimp_procedure_new (parasite_find_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-parasite-find");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-parasite-find",
|
|
"Look up a global parasite.",
|
|
"Finds and returns the global parasite that was previously attached.",
|
|
"Jay Cox",
|
|
"Jay Cox",
|
|
"1998",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("name",
|
|
"name",
|
|
"The name of the parasite to find",
|
|
FALSE, FALSE, FALSE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_return_value (procedure,
|
|
gimp_param_spec_parasite ("parasite",
|
|
"parasite",
|
|
"The found parasite",
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-parasite-attach
|
|
*/
|
|
procedure = gimp_procedure_new (parasite_attach_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-parasite-attach");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-parasite-attach",
|
|
"Add a global parasite.",
|
|
"This procedure attaches a global parasite. It has no return values.",
|
|
"Jay Cox",
|
|
"Jay Cox",
|
|
"1998",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_parasite ("parasite",
|
|
"parasite",
|
|
"The parasite to attach",
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-parasite-detach
|
|
*/
|
|
procedure = gimp_procedure_new (parasite_detach_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-parasite-detach");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-parasite-detach",
|
|
"Removes a global parasite.",
|
|
"This procedure detaches a global parasite from. It has no return values.",
|
|
"Jay Cox",
|
|
"Jay Cox",
|
|
"1998",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("name",
|
|
"name",
|
|
"The name of the parasite to detach.",
|
|
FALSE, FALSE, FALSE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-parasite-list
|
|
*/
|
|
procedure = gimp_procedure_new (parasite_list_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-parasite-list");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-parasite-list",
|
|
"List all parasites.",
|
|
"Returns a list of all currently attached global parasites.",
|
|
"Marc Lehmann",
|
|
"Marc Lehmann",
|
|
"1999",
|
|
NULL);
|
|
gimp_procedure_add_return_value (procedure,
|
|
gimp_param_spec_int32 ("num-parasites",
|
|
"num parasites",
|
|
"The number of attached parasites",
|
|
0, G_MAXINT32, 0,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_return_value (procedure,
|
|
gimp_param_spec_string_array ("parasites",
|
|
"parasites",
|
|
"The names of currently attached parasites",
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-image-parasite-find
|
|
*/
|
|
procedure = gimp_procedure_new (image_parasite_find_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-image-parasite-find");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-image-parasite-find",
|
|
"Look up a parasite in an image",
|
|
"Finds and returns the parasite that was previously attached to an image.",
|
|
"Jay Cox",
|
|
"Jay Cox",
|
|
"1998",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_image_id ("image",
|
|
"image",
|
|
"The image",
|
|
pdb->gimp, FALSE,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("name",
|
|
"name",
|
|
"The name of the parasite to find",
|
|
FALSE, FALSE, FALSE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_return_value (procedure,
|
|
gimp_param_spec_parasite ("parasite",
|
|
"parasite",
|
|
"The found parasite",
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-image-parasite-attach
|
|
*/
|
|
procedure = gimp_procedure_new (image_parasite_attach_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-image-parasite-attach");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-image-parasite-attach",
|
|
"Add a parasite to an image.",
|
|
"This procedure attaches a parasite to an image. It has no return values.",
|
|
"Jay Cox",
|
|
"Jay Cox",
|
|
"1998",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_image_id ("image",
|
|
"image",
|
|
"The image",
|
|
pdb->gimp, FALSE,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_parasite ("parasite",
|
|
"parasite",
|
|
"The parasite to attach to an image",
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-image-parasite-detach
|
|
*/
|
|
procedure = gimp_procedure_new (image_parasite_detach_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-image-parasite-detach");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-image-parasite-detach",
|
|
"Removes a parasite from an image.",
|
|
"This procedure detaches a parasite from an image. It has no return values.",
|
|
"Jay Cox",
|
|
"Jay Cox",
|
|
"1998",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_image_id ("image",
|
|
"image",
|
|
"The image",
|
|
pdb->gimp, FALSE,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("name",
|
|
"name",
|
|
"The name of the parasite to detach from an image.",
|
|
FALSE, FALSE, FALSE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-image-parasite-list
|
|
*/
|
|
procedure = gimp_procedure_new (image_parasite_list_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-image-parasite-list");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-image-parasite-list",
|
|
"List all parasites.",
|
|
"Returns a list of all currently attached parasites.",
|
|
"Marc Lehmann",
|
|
"Marc Lehmann",
|
|
"1999",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_image_id ("image",
|
|
"image",
|
|
"The image",
|
|
pdb->gimp, FALSE,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_return_value (procedure,
|
|
gimp_param_spec_int32 ("num-parasites",
|
|
"num parasites",
|
|
"The number of attached parasites",
|
|
0, G_MAXINT32, 0,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_return_value (procedure,
|
|
gimp_param_spec_string_array ("parasites",
|
|
"parasites",
|
|
"The names of currently attached parasites",
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-drawable-parasite-find
|
|
*/
|
|
procedure = gimp_procedure_new (drawable_parasite_find_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-drawable-parasite-find");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-drawable-parasite-find",
|
|
"Look up a parasite in a drawable",
|
|
"Finds and returns the parasite that was previously attached to a drawable.",
|
|
"Jay Cox",
|
|
"Jay Cox",
|
|
"1998",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_drawable_id ("drawable",
|
|
"drawable",
|
|
"The drawable",
|
|
pdb->gimp, FALSE,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("name",
|
|
"name",
|
|
"The name of the parasite to find",
|
|
FALSE, FALSE, FALSE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_return_value (procedure,
|
|
gimp_param_spec_parasite ("parasite",
|
|
"parasite",
|
|
"The found parasite",
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-drawable-parasite-attach
|
|
*/
|
|
procedure = gimp_procedure_new (drawable_parasite_attach_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-drawable-parasite-attach");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-drawable-parasite-attach",
|
|
"Add a parasite to a drawable.",
|
|
"This procedure attaches a parasite to a drawable. It has no return values.",
|
|
"Jay Cox",
|
|
"Jay Cox",
|
|
"1998",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_drawable_id ("drawable",
|
|
"drawable",
|
|
"The drawable",
|
|
pdb->gimp, FALSE,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_parasite ("parasite",
|
|
"parasite",
|
|
"The parasite to attach to a drawable",
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-drawable-parasite-detach
|
|
*/
|
|
procedure = gimp_procedure_new (drawable_parasite_detach_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-drawable-parasite-detach");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-drawable-parasite-detach",
|
|
"Removes a parasite from a drawable.",
|
|
"This procedure detaches a parasite from a drawable. It has no return values.",
|
|
"Jay Cox",
|
|
"Jay Cox",
|
|
"1998",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_drawable_id ("drawable",
|
|
"drawable",
|
|
"The drawable",
|
|
pdb->gimp, FALSE,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("name",
|
|
"name",
|
|
"The name of the parasite to detach from a drawable.",
|
|
FALSE, FALSE, FALSE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-drawable-parasite-list
|
|
*/
|
|
procedure = gimp_procedure_new (drawable_parasite_list_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-drawable-parasite-list");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-drawable-parasite-list",
|
|
"List all parasites.",
|
|
"Returns a list of all currently attached parasites.",
|
|
"Marc Lehmann",
|
|
"Marc Lehmann",
|
|
"1999",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_drawable_id ("drawable",
|
|
"drawable",
|
|
"The drawable",
|
|
pdb->gimp, FALSE,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_return_value (procedure,
|
|
gimp_param_spec_int32 ("num-parasites",
|
|
"num parasites",
|
|
"The number of attached parasites",
|
|
0, G_MAXINT32, 0,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_return_value (procedure,
|
|
gimp_param_spec_string_array ("parasites",
|
|
"parasites",
|
|
"The names of currently attached parasites",
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-vectors-parasite-find
|
|
*/
|
|
procedure = gimp_procedure_new (vectors_parasite_find_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-vectors-parasite-find");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-vectors-parasite-find",
|
|
"Look up a parasite in a vectors object",
|
|
"Finds and returns the parasite that was previously attached to a vectors object.",
|
|
"Michael Natterer <mitch@gimp.org>",
|
|
"Michael Natterer",
|
|
"2006",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_vectors_id ("vectors",
|
|
"vectors",
|
|
"The vectors object",
|
|
pdb->gimp, FALSE,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("name",
|
|
"name",
|
|
"The name of the parasite to find",
|
|
FALSE, FALSE, FALSE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_return_value (procedure,
|
|
gimp_param_spec_parasite ("parasite",
|
|
"parasite",
|
|
"The found parasite",
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-vectors-parasite-attach
|
|
*/
|
|
procedure = gimp_procedure_new (vectors_parasite_attach_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-vectors-parasite-attach");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-vectors-parasite-attach",
|
|
"Add a parasite to a vectors object",
|
|
"This procedure attaches a parasite to a vectors object. It has no return values.",
|
|
"Michael Natterer <mitch@gimp.org>",
|
|
"Michael Natterer",
|
|
"2006",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_vectors_id ("vectors",
|
|
"vectors",
|
|
"The vectors object",
|
|
pdb->gimp, FALSE,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_parasite ("parasite",
|
|
"parasite",
|
|
"The parasite to attach to a vectors object",
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-vectors-parasite-detach
|
|
*/
|
|
procedure = gimp_procedure_new (vectors_parasite_detach_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-vectors-parasite-detach");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-vectors-parasite-detach",
|
|
"Removes a parasite from a vectors object",
|
|
"This procedure detaches a parasite from a vectors object. It has no return values.",
|
|
"Michael Natterer <mitch@gimp.org>",
|
|
"Michael Natterer",
|
|
"2006",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_vectors_id ("vectors",
|
|
"vectors",
|
|
"The vectors object",
|
|
pdb->gimp, FALSE,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("name",
|
|
"name",
|
|
"The name of the parasite to detach from a vectors object.",
|
|
FALSE, FALSE, FALSE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-vectors-parasite-list
|
|
*/
|
|
procedure = gimp_procedure_new (vectors_parasite_list_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-vectors-parasite-list");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-vectors-parasite-list",
|
|
"List all parasites.",
|
|
"Returns a list of all currently attached parasites.",
|
|
"Michael Natterer <mitch@gimp.org>",
|
|
"Michael Natterer",
|
|
"2006",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_vectors_id ("vectors",
|
|
"vectors",
|
|
"The vectors object",
|
|
pdb->gimp, FALSE,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_return_value (procedure,
|
|
gimp_param_spec_int32 ("num-parasites",
|
|
"num parasites",
|
|
"The number of attached parasites",
|
|
0, G_MAXINT32, 0,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_return_value (procedure,
|
|
gimp_param_spec_string_array ("parasites",
|
|
"parasites",
|
|
"The names of currently attached parasites",
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
}
|