mirror of
https://gitlab.gnome.org/GNOME/gimp
synced 2024-10-20 19:43:01 +00:00
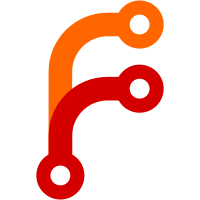
2001-07-04 Michael Natterer <mitch@gimp.org> * app/core/Makefile.am * app/core/core-types.h * app/core/gimp.[ch]: added an "application object" called Gimp. Currently, it contains the image list, the clipboard, the data factories, the procedural hashtable and the tool info list. It's the toplevel object of the core object system. Finally, creating a Gimp object will return a standalone gimp core engine instance with no other global states/variables involved. * app/app_procs.[ch]: allocate a "Gimp" instance called "the_gimp" :) Removed stuff which is now done by the "Gimp" object. Merged gimp_init() into app_init() because gimp_init() is taken now. * app/context_manager.[ch]: removed stuff done by "Gimp". * app/batch.[ch] * app/gimage.[ch] * app/xcf/xcf-load.[ch] * app/xcf/xcf.[ch] * app/core/gimpedit.[ch] * app/tools/tool_manager.[ch]: pass around an additional "Gimp" argument. * app/pdb/procedural_db.[ch]: pass a "Gimp" pointer as first parameter to all internal procedures and to all procedural_db_* functions. * app/core/gimpcontext.[ch] * app/core/gimpimage.[ch]: added a "Gimp" pointer to the structs. * app/devices.c * app/errors.c * app/file-open.c * app/file-save.c * app/gimphelp.c * app/gimpunit.c * app/image_new.c * app/main.c * app/nav_window.c * app/plug_in.c * app/base/base.c * app/core/gimpdatafactory.c * app/core/gimpimage-duplicate.c * app/core/gimpimage-mask.c * app/core/gimptoolinfo.[ch] * app/gui/brush-select.c * app/gui/convert-dialog.c * app/gui/dialogs-constructors.c * app/gui/edit-commands.c * app/gui/file-open-dialog.c * app/gui/file-save-dialog.c * app/gui/gradient-editor.c * app/gui/gradient-select.c * app/gui/gui.c * app/gui/image-commands.c * app/gui/info-window.c * app/gui/menus.c * app/gui/palette-editor.c * app/gui/palette-import-dialog.c * app/gui/palette-select.c * app/gui/paths-dialog.c * app/gui/pattern-select.c * app/gui/preferences-dialog.c * app/gui/test-commands.c * app/gui/toolbox.c * app/gui/tools-commands.c * app/tools/gimpbezierselecttool.c * app/tools/gimpbucketfilltool.c * app/tools/gimppainttool.h * app/tools/gimptexttool.c * app/tools/gimptransformtool.h * app/widgets/gimpbufferview.c * app/widgets/gimpcontainerview-utils.c * app/widgets/gimpcursor.c * app/widgets/gimpdnd.c * app/widgets/gimpimagedock.c: changed accordingly. Cleaned up lots of includes. Many files still access the global "the_gimp" variable exported by app_procs.h. * tools/pdbgen/app.pl * tools/pdbgen/pdb/brush_select.pdb * tools/pdbgen/pdb/brushes.pdb * tools/pdbgen/pdb/convert.pdb * tools/pdbgen/pdb/edit.pdb * tools/pdbgen/pdb/fileops.pdb * tools/pdbgen/pdb/gradient_select.pdb * tools/pdbgen/pdb/gradients.pdb * tools/pdbgen/pdb/image.pdb * tools/pdbgen/pdb/palette.pdb * tools/pdbgen/pdb/pattern_select.pdb * tools/pdbgen/pdb/patterns.pdb * tools/pdbgen/pdb/procedural_db.pdb: changed accordingly. Don't use "the_gimp" here because all procedures get passed a "Gimp" pointer now. * app/pdb/*: regenerated.
148 lines
4.6 KiB
C
148 lines
4.6 KiB
C
/* The GIMP -- an image manipulation program
|
|
* Copyright (C) 1995 Spencer Kimball and Peter Mattis
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef __PROCEDURAL_DB_H__
|
|
#define __PROCEDURAL_DB_H__
|
|
|
|
|
|
struct _Argument
|
|
{
|
|
GimpPDBArgType arg_type; /* argument type */
|
|
|
|
union _ArgValue
|
|
{
|
|
gint32 pdb_int; /* Integer type */
|
|
gdouble pdb_float; /* Floating point type */
|
|
gpointer pdb_pointer; /* Pointer type */
|
|
GimpRGB pdb_color; /* Color type */
|
|
} value;
|
|
};
|
|
|
|
|
|
/* Argument marshalling procedures */
|
|
typedef Argument * (* ArgMarshal) (Gimp *gimp,
|
|
Argument *args);
|
|
|
|
|
|
/* Execution types */
|
|
typedef struct _IntExec IntExec;
|
|
typedef struct _PlugInExec PlugInExec;
|
|
typedef struct _ExtExec ExtExec;
|
|
typedef struct _TempExec TempExec;
|
|
typedef struct _NetExec NetExec;
|
|
|
|
|
|
struct _IntExec
|
|
{
|
|
ArgMarshal marshal_func; /* Function called to marshal arguments */
|
|
};
|
|
|
|
struct _PlugInExec
|
|
{
|
|
gchar *filename; /* Where is the executable on disk? */
|
|
};
|
|
|
|
struct _ExtExec
|
|
{
|
|
gchar *filename; /* Where is the executable on disk? */
|
|
};
|
|
|
|
struct _TempExec
|
|
{
|
|
void *plug_in; /* Plug-in that registered this temp proc */
|
|
};
|
|
|
|
struct _NetExec
|
|
{
|
|
gchar *host; /* Host responsible for procedure execution */
|
|
gint32 port; /* Port on host to send data to */
|
|
};
|
|
|
|
|
|
/* Structure for a procedure argument */
|
|
|
|
struct _ProcArg
|
|
{
|
|
GimpPDBArgType arg_type; /* Argument type (int, char, char *, etc) */
|
|
gchar *name; /* Argument name */
|
|
gchar *description; /* Argument description */
|
|
};
|
|
|
|
|
|
/* Structure for a procedure */
|
|
|
|
struct _ProcRecord
|
|
{
|
|
/* Procedure information */
|
|
gchar *name; /* Procedure name */
|
|
gchar *blurb; /* Short procedure description */
|
|
gchar *help; /* Detailed help instructions */
|
|
gchar *author; /* Author field */
|
|
gchar *copyright; /* Copyright field */
|
|
gchar *date; /* Date field */
|
|
|
|
/* Procedure type */
|
|
GimpPDBProcType proc_type; /* Type of procedure--Internal, Plug-In, Extension */
|
|
|
|
/* Input arguments */
|
|
gint32 num_args; /* Number of procedure arguments */
|
|
ProcArg *args; /* Array of procedure arguments */
|
|
|
|
/* Output values */
|
|
gint32 num_values; /* Number of return values */
|
|
ProcArg *values; /* Array of return values */
|
|
|
|
/* Method of procedure execution */
|
|
union _ExecMethod
|
|
{
|
|
IntExec internal; /* Execution information for internal procs */
|
|
PlugInExec plug_in; /* ..................... for plug-ins */
|
|
ExtExec extension; /* ..................... for extensions */
|
|
TempExec temporary; /* ..................... for temp procs */
|
|
} exec_method;
|
|
};
|
|
|
|
|
|
/* Functions */
|
|
void procedural_db_init (Gimp *gimp);
|
|
void procedural_db_free (Gimp *gimp);
|
|
void procedural_db_register (Gimp *gimp,
|
|
ProcRecord *procedure);
|
|
void procedural_db_unregister (Gimp *gimp,
|
|
const gchar *name);
|
|
ProcRecord * procedural_db_lookup (Gimp *gimp,
|
|
const gchar *name);
|
|
Argument * procedural_db_execute (Gimp *gimp,
|
|
const gchar *name,
|
|
Argument *args);
|
|
Argument * procedural_db_run_proc (Gimp *gimp,
|
|
const gchar *name,
|
|
gint *nreturn_vals,
|
|
...);
|
|
Argument * procedural_db_return_args (ProcRecord *procedure,
|
|
gboolean success);
|
|
void procedural_db_destroy_args (Argument *args,
|
|
gint nargs);
|
|
|
|
/* "type" should really be a GimpPDBArgType, but we can cope with
|
|
* out-of-range values.
|
|
*/
|
|
const gchar * pdb_type_name (gint type); /* really exists in _cmds.c file */
|
|
|
|
#endif /* __PROCEDURAL_DB_H__ */
|