mirror of
https://gitlab.gnome.org/GNOME/gimp
synced 2024-10-21 03:52:33 +00:00
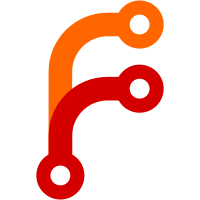
2001-05-15 Michael Natterer <mitch@gimp.org> * configure.in: new directory app/base/ * app/Makefile.am * app/boundary.[ch] * app/brush_scale.[ch] * app/gimpchecks.h * app/gimplut.[ch] * app/pixel_processor.[ch] * app/pixel_region.[ch] * app/pixel_surround.[ch] * app/temp_buf.[ch] * app/tile.[ch] * app/tile_cache.[ch] * app/tile_manager.[ch] * app/tile_manager_pvt.h * app/tile_pvt.h * app/tile_swap.[ch]: moved to base/ * app/base/Makefile.am * app/base/base-types.h * app/base/*: new directory for the sub-object pixel maniplation and storage stuff. Does not include Gtk+ or anything outside base/. Did some cleanup in all files. * app/appenums.h * app/apptypes.h * app/core/gimpimage.h: removed types which are now in base/base-types.h. * app/base/base-config.[ch] * app/gimprc.[ch]: put the config variables for base/ to their own file so base/ doesn not have to include gimprc.h (does not yet work, i.e. the variables are un-configurable right now) * app/main.c: set a log handler for "Gimp-Base". * app/paint-funcs/Makefile.am * app/paint-funcs/paint-funcs.[ch]: removed the color hash which maps RGB to color indices because it's a totally standalone system which has nothing to do with the paint-funcs and introduced a GimpImage dependency. paint-funcs/ should be considered on the same sub-object (glib-only) level as base/, only in a different directory. * app/core/Makefile.am * app/core/gimpimage-colorhash.[ch]: put the color hash here. * app/gimage.c: don't invalidate the color hash here... * app/core/gimpimage.c: ... but in the colormap_changed() default inplementation. Initialize the hash in class_init(). * tools/pdbgen/Makefile.am: scan app/base/base-types.h for enums. * tools/pdbgen/enums.pl: regenerated. * app/[lots] * app/core/[of] * app/gui/[files] * app/pdb/[all] * app/tools/[over] * app/widgets/[the] * tools/pdbgen/pdb/[place]: changed #includes accordingly. And use base_config->value instead of the stuff from gimprc.h.
233 lines
6 KiB
Plaintext
233 lines
6 KiB
Plaintext
# The GIMP -- an image manipulation program
|
|
# Copyright (C) 1995 Spencer Kimball and Peter Mattis
|
|
|
|
# This program is free software; you can redistribute it and/or modify
|
|
# it under the terms of the GNU General Public License as published by
|
|
# the Free Software Foundation; either version 2 of the License, or
|
|
# (at your option) any later version.
|
|
|
|
# This program is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
|
|
# You should have received a copy of the GNU General Public License
|
|
# along with this program; if not, write to the Free Software
|
|
# Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
|
|
# "Perlized" from C source by Manish Singh <yosh@gimp.org>
|
|
|
|
sub pattern_arg () {{
|
|
name => 'name',
|
|
type => 'string',
|
|
desc => 'The pattern name'
|
|
}}
|
|
|
|
sub dim_args () {
|
|
my @args;
|
|
foreach (qw(width height)) {
|
|
push @args, { name => $_, type => 'int32', desc => "The pattern $_" };
|
|
}
|
|
@args;
|
|
}
|
|
|
|
sub pattern_outargs {
|
|
foreach (@outargs) {
|
|
my $alias;
|
|
if ($_->{type} eq 'string') {
|
|
$alias = "GIMP_OBJECT (pattern)->$_->{name}";
|
|
} else {
|
|
$alias = "pattern->$_->{name}";
|
|
}
|
|
$alias = "g_strdup ($alias)" if $_->{type} eq 'string';
|
|
$alias =~ s/pattern/pattern->mask/ if $_->{name} =~ /width|height/;
|
|
$_->{alias} = $alias;
|
|
$_->{no_declare} = 1;
|
|
}
|
|
}
|
|
|
|
# The defs
|
|
|
|
sub patterns_get_pattern {
|
|
$blurb = 'Retrieve information about the currently active pattern.';
|
|
|
|
$help = <<'HELP';
|
|
This procedure retrieves information about the currently active pattern. This
|
|
includes the pattern name, and the pattern extents (width and height). All
|
|
clone and bucket-fill operations with patterns will use this pattern to control
|
|
the application of paint to the image.
|
|
HELP
|
|
|
|
&std_pdb_misc;
|
|
|
|
@outargs = (
|
|
&pattern_arg,
|
|
&dim_args,
|
|
);
|
|
|
|
&pattern_outargs;
|
|
|
|
%invoke = (
|
|
vars => [ 'GimpPattern *pattern' ],
|
|
code => 'success = (pattern = gimp_context_get_pattern (NULL)) != NULL;'
|
|
);
|
|
}
|
|
|
|
sub patterns_set_pattern {
|
|
$blurb = 'Set the specified pattern as the active pattern.';
|
|
|
|
$help = <<'HELP';
|
|
This procedure allows the active pattern mask to be set by specifying its name.
|
|
The name is simply a string which corresponds to one of the names of the
|
|
installed patterns. If there is no matching pattern found, this procedure will
|
|
return an error. Otherwise, the specified pattern becomes active and will be
|
|
used in all subsequent paint operations.
|
|
HELP
|
|
|
|
&std_pdb_misc;
|
|
|
|
@inargs = ( &pattern_arg );
|
|
|
|
%invoke = (
|
|
vars => [ 'GimpPattern *pattern', 'GList *list' ],
|
|
code => <<'CODE'
|
|
{
|
|
success = FALSE;
|
|
|
|
for (list = GIMP_LIST (global_pattern_factory->container)->list;
|
|
list;
|
|
list = g_list_next (list))
|
|
{
|
|
pattern = (GimpPattern *) list->data;
|
|
|
|
if (! strcmp (GIMP_OBJECT (pattern)->name, name))
|
|
{
|
|
gimp_context_set_pattern (NULL, pattern);
|
|
success = TRUE;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
CODE
|
|
);
|
|
}
|
|
|
|
sub patterns_list {
|
|
$blurb = 'Retrieve a complete listing of the available patterns.';
|
|
|
|
$help = <<'HELP';
|
|
This procedure returns a complete listing of available GIMP patterns. Each name
|
|
returned can be used as input to the 'gimp_patterns_set_pattern'.
|
|
HELP
|
|
|
|
&std_pdb_misc;
|
|
|
|
@outargs = (
|
|
{ name => 'pattern_list', type => 'stringarray',
|
|
desc => 'The list of pattern names',
|
|
alias => 'patterns',
|
|
array => { name => 'num_patterns',
|
|
desc => 'The number of patterns in the pattern list',
|
|
alias => 'global_pattern_factory->container->num_children', no_declare => 1 } }
|
|
);
|
|
|
|
%invoke = (
|
|
vars => [ 'GList *list = NULL', 'gint i = 0' ],
|
|
code => <<'CODE'
|
|
{
|
|
patterns = g_new (gchar *, global_pattern_factory->container->num_children);
|
|
|
|
success = ((list = GIMP_LIST (global_pattern_factory->container)->list) != NULL);
|
|
|
|
while (list)
|
|
{
|
|
patterns[i++] = g_strdup (GIMP_OBJECT (list->data)->name);
|
|
list = list->next;
|
|
}
|
|
}
|
|
CODE
|
|
);
|
|
}
|
|
|
|
sub patterns_get_pattern_data {
|
|
$blurb = <<'BLURB';
|
|
Retrieve information about the currently active pattern (including data).
|
|
BLURB
|
|
|
|
$help = <<'HELP';
|
|
This procedure retrieves information about the currently active pattern. This
|
|
includes the pattern name, and the pattern extents (width and height). It also
|
|
returns the pattern data.
|
|
HELP
|
|
|
|
$author = $copyright = 'Andy Thomas';
|
|
$date = '1998';
|
|
|
|
@inargs = ( &pattern_arg );
|
|
$inargs[0]->{desc} = 'the pattern name ("" means current active pattern)';
|
|
|
|
@outargs = (
|
|
&pattern_arg,
|
|
&dim_args,
|
|
);
|
|
|
|
&pattern_outargs;
|
|
|
|
push @outargs, { name => 'mask_bpp', type => 'int32', init => 1,
|
|
desc => 'Pattern bytes per pixel',
|
|
alias => 'pattern->mask->bytes', no_declare => 1 },
|
|
{ name => 'mask_data', type => 'int8array', init => 1,
|
|
desc => 'The pattern mask data',
|
|
array => { name => 'length', init => 1,
|
|
desc => 'Length of pattern mask data' } };
|
|
|
|
%invoke = (
|
|
headers => [ qw(<string.h>) ],
|
|
vars => [ 'GimpPattern *pattern = NULL' ],
|
|
code => <<'CODE'
|
|
{
|
|
if (strlen (name))
|
|
{
|
|
GList *list;
|
|
|
|
success = FALSE;
|
|
|
|
for (list = GIMP_LIST (global_pattern_factory->container)->list;
|
|
list;
|
|
list = g_list_next (list))
|
|
{
|
|
pattern = (GimpPattern *) list->data;
|
|
|
|
if (!strcmp (GIMP_OBJECT (pattern)->name, name))
|
|
{
|
|
success = TRUE;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
else
|
|
success = (pattern = gimp_context_get_pattern (NULL)) != NULL;
|
|
|
|
if (success)
|
|
{
|
|
length = pattern->mask->height * pattern->mask->width *
|
|
pattern->mask->bytes;
|
|
mask_data = g_new (guint8, length);
|
|
g_memmove (mask_data, temp_buf_data (pattern->mask), length);
|
|
}
|
|
}
|
|
CODE
|
|
);
|
|
}
|
|
|
|
@headers = qw("context_manager.h" "core/gimpcontext.h" "core/gimpdatafactory.h"
|
|
"core/gimplist.h" "core/gimppattern.h" "base/temp-buf.h");
|
|
|
|
@procs = qw(patterns_get_pattern patterns_set_pattern patterns_list
|
|
patterns_get_pattern_data);
|
|
%exports = (app => [@procs], lib => [$procs[3]]);
|
|
|
|
$desc = 'Patterns';
|
|
|
|
1;
|