mirror of
https://gitlab.gnome.org/GNOME/gimp
synced 2024-10-20 08:37:21 +00:00
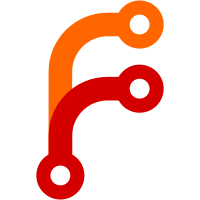
Sun May 9 16:23:47 BST 1999 Adam D. Moss <adam@gimp.org> * app/tile.c * app/tile.h * app/tile_manager.c * app/tile_pvt.h * app/paint_funcs.c: Added Tile Row Hinting to the GIMP tile structure. Tiles now have cheap per-row hints indicating whether each row is all-transparent, all-opaque, a mixture, or other properties. These hints are automatically invalidated when the tile is checked in as dirty, and are re-evaluated on demand. Currently only the layer compositing routines take advantage of these hints, though there is opportunity to use them to advantage in numerous other places. The whole layer compositing process is typically 2x-4x faster now, especially on subsequent renders of data which has already had its hints calculated. See tile.h for the explicit TileRowHint query/set interface. The procedure to re-evaluate tile hints currently resides in paint_funcs.c but may be exposed to other parts of the core if necessary. This is experimental. Please report mis-rendering problems.
83 lines
2.5 KiB
C
83 lines
2.5 KiB
C
#ifndef __TILE_PVT_H__
|
|
#define __TILE_PVT_H__
|
|
|
|
#ifdef USE_PTHREADS
|
|
#include <pthread.h>
|
|
#endif
|
|
|
|
|
|
#include <sys/types.h>
|
|
#include <glib.h>
|
|
|
|
#include "config.h"
|
|
#include "tile.h"
|
|
|
|
typedef struct _TileLink TileLink;
|
|
|
|
struct _TileLink
|
|
{
|
|
TileLink *next;
|
|
int tile_num; /* the number of this tile within the drawable */
|
|
void *tm; /* A pointer to the tile manager for this tile.
|
|
* We need this in order to call the tile managers
|
|
* validate proc whenever the tile is referenced yet
|
|
* invalid.
|
|
*/
|
|
};
|
|
|
|
struct _Tile
|
|
{
|
|
short ref_count; /* reference count. when the reference count is
|
|
* non-zero then the "data" for this tile must
|
|
* be valid. when the reference count for a tile
|
|
* is 0 then the "data" for this tile must be
|
|
* NULL.
|
|
*/
|
|
short write_count; /* write count: number of references that are
|
|
for write access */
|
|
short share_count; /* share count: number of tile managers that
|
|
hold this tile */
|
|
guint dirty : 1; /* is the tile dirty? has it been modified? */
|
|
guint valid : 1; /* is the tile valid? */
|
|
|
|
/* An array of hints for rendering purposes */
|
|
TileRowHint rowhint[TILE_HEIGHT];
|
|
|
|
guchar *data; /* the data for the tile. this may be NULL in which
|
|
* case the tile data is on disk.
|
|
*/
|
|
|
|
int ewidth; /* the effective width of the tile */
|
|
int eheight; /* the effective height of the tile */
|
|
/* a tile's effective width and height may be smaller
|
|
* (but not larger) than TILE_WIDTH and TILE_HEIGHT.
|
|
* this is to handle edge tiles of a drawable.
|
|
*/
|
|
int bpp; /* the bytes per pixel (1, 2, 3 or 4) */
|
|
int swap_num; /* the index into the file table of the file to be used
|
|
* for swapping. swap_num 1 is always the global swap file.
|
|
*/
|
|
off_t swap_offset; /* the offset within the swap file of the tile data.
|
|
* if the tile data is in memory this will be set to -1.
|
|
*/
|
|
TileLink *tlink;
|
|
|
|
Tile *next;
|
|
Tile *prev; /* List pointers for the tile cache lists */
|
|
void *listhead; /* Pointer to the head of the list this tile is on */
|
|
#ifdef USE_PTHREADS
|
|
pthread_mutex_t mutex;
|
|
#endif
|
|
};
|
|
|
|
#ifdef USE_PTHREADS
|
|
#define TILE_MUTEX_LOCK(tile) pthread_mutex_lock(&((tile)->mutex))
|
|
#define TILE_MUTEX_UNLOCK(tile) pthread_mutex_unlock(&((tile)->mutex))
|
|
#else
|
|
#define TILE_MUTEX_LOCK(tile) /* nothing */
|
|
#define TILE_MUTEX_UNLOCK(tile) /* nothing */
|
|
#endif
|
|
|
|
|
|
#endif /* __TILE_PVT_H__ */
|