mirror of
https://gitlab.gnome.org/GNOME/gimp
synced 2024-10-19 22:34:37 +00:00
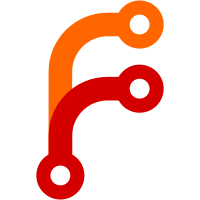
2002-09-10 Michael Natterer <mitch@gimp.org> Some PDB cleanup: * tools/pdbgen/pdb/procedural_db.pdb: removed the get_data() and set_data() implementations and the global "data_list" variable. Cleaned up the dump() stuff (pass the FILE pointer around instead of having a global variable for it). Fixed output_string() so it does not crash on NULL strings. * app/core/gimp.[ch]: added gimp->procedural_db_data_list. * app/pdb/procedural_db.[ch]: added procedural_db_[set|get]_data(). Don't leak data identifiers when overwriting an already existing entry. Added g_return_if_fail() stuff to all public functions. * tools/pdbgen/pdb/channel.pdb * tools/pdbgen/pdb/drawable.pdb * tools/pdbgen/pdb/layer.pdb * tools/pdbgen/pdb/parasite.pdb: tweaked some helper functions to take parameters which make them aware of the real type of the objects they handle (e.g. the PDB function gimp_layer_set_name() matches the core function gimp_object_get_name()). * app/pdb/pdb_glue.h: removed ugly CPP-level workarounds for the issue mentioned above. * app/pdb/channel_cmds.c * app/pdb/drawable_cmds.c * app/pdb/layer_cmds.c * app/pdb/parasite_cmds.c * app/pdb/procedural_db_cmds.c: regenerated.
556 lines
15 KiB
Plaintext
556 lines
15 KiB
Plaintext
# The GIMP -- an image manipulation program
|
|
# Copyright (C) 1995 Spencer Kimball and Peter Mattis
|
|
|
|
# This program is free software; you can redistribute it and/or modify
|
|
# it under the terms of the GNU General Public License as published by
|
|
# the Free Software Foundation; either version 2 of the License, or
|
|
# (at your option) any later version.
|
|
|
|
# This program is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
|
|
# You should have received a copy of the GNU General Public License
|
|
# along with this program; if not, write to the Free Software
|
|
# Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
|
|
# "Perlized" from C source by Manish Singh <yosh@gimp.org>
|
|
|
|
sub drawable_arg () {{
|
|
name => 'drawable',
|
|
type => 'drawable',
|
|
desc => 'The drawable',
|
|
}}
|
|
|
|
sub drawable_coord_args () {
|
|
@inargs = ( &drawable_arg );
|
|
|
|
foreach (qw(x y)) {
|
|
push @inargs, { name => "${_}_coord", type => '0 <= int32',
|
|
desc => "The $_ coordinate", alias => $_ }
|
|
}
|
|
}
|
|
|
|
sub pixel_arg () {{
|
|
name => 'pixel',
|
|
type => 'int8array',
|
|
desc => 'The pixel value',
|
|
array => { name => 'num_channels', type => 'int32',
|
|
desc => 'The number of channels for the pixel',
|
|
no_success => 1 }
|
|
}}
|
|
|
|
sub drawable_prop_proc {
|
|
my ($return, $name, $type, $func, $desc) = @_;
|
|
|
|
$blurb = "Returns $return.";
|
|
|
|
&std_pdb_misc;
|
|
|
|
@inargs = ( &drawable_arg );
|
|
|
|
@outargs = (
|
|
{ name => $name, type => $type, desc => $desc,
|
|
alias => "gimp_drawable_$func (drawable)", no_declare => 1 }
|
|
);
|
|
}
|
|
|
|
sub drawable_type_proc {
|
|
my ($desc, $type, $func) = @_;
|
|
|
|
$help = <<HELP;
|
|
This procedure returns non-zero if the specified drawable is of type
|
|
{ $type }.
|
|
HELP
|
|
|
|
&drawable_prop_proc("whether the drawable is $desc type", $func, 'boolean',
|
|
$func, "non-zero if the drawable is $desc type");
|
|
}
|
|
|
|
sub drawable_is_proc {
|
|
my ($desc, $check) = @_;
|
|
|
|
my $type = $desc;
|
|
$type =~ s/ /_/g;
|
|
|
|
$help = <<HELP;
|
|
This procedure returns non-zero if the specified drawable is a $desc.
|
|
HELP
|
|
|
|
&drawable_prop_proc("whether the drawable is a $desc",
|
|
$type, 'boolean', $type,
|
|
"Non-zero if the drawable is a $desc");
|
|
|
|
$outargs[0]->{alias} = $check . ' (drawable) ? TRUE : FALSE';
|
|
}
|
|
|
|
sub drawable_merge_shadow {
|
|
$blurb = 'Merge the shadow buffer with the specified drawable.';
|
|
|
|
$help = <<'HELP';
|
|
This procedure combines the contents of the image's shadow buffer (for
|
|
temporary processing) with the specified drawable. The "undo" parameter
|
|
specifies whether to add an undo step for the operation. Requesting no undo is
|
|
useful for such applications as 'auto-apply'.
|
|
HELP
|
|
|
|
&std_pdb_misc;
|
|
|
|
@inargs = (
|
|
&drawable_arg,
|
|
{ name => 'undo', type => 'boolean',
|
|
desc => 'Push merge to undo stack?' }
|
|
);
|
|
|
|
%invoke = ( code => 'gimp_drawable_merge_shadow (drawable, undo);' );
|
|
}
|
|
|
|
sub drawable_fill {
|
|
$blurb = 'Fill the drawable with the specified fill mode.';
|
|
|
|
$help = <<'HELP';
|
|
This procedure fills the drawable with the fill mode. If the fill mode is
|
|
foreground the current foreground color is used. If the fill mode is
|
|
background, the current background color is used. If the fill type is white,
|
|
then white is used. Transparent fill only affects layers with an alpha channel,
|
|
in which case the alpha channel is set to transparent. If the drawable has no
|
|
alpha channel, it is filled to white. No fill leaves the drawable's contents
|
|
undefined. This procedure is unlike the bucket fill tool because it fills
|
|
regardless of a selection
|
|
HELP
|
|
|
|
&std_pdb_misc;
|
|
|
|
@inargs = (
|
|
&drawable_arg,
|
|
{ name => 'fill_type', type => 'enum GimpFillType',
|
|
desc => 'The type of fill: %%desc%%' }
|
|
);
|
|
|
|
%invoke = ( code => 'gimp_drawable_fill_by_type (drawable, gimp_get_current_context (gimp), (GimpFillType) fill_type);' );
|
|
}
|
|
|
|
sub drawable_update {
|
|
$blurb = 'Update the specified region of the drawable.';
|
|
|
|
$help = <<'HELP';
|
|
This procedure updates the specified region of the drawable. The (x, y)
|
|
coordinate pair is relative to the drawable's origin, not to the image origin.
|
|
Therefore, the entire drawable can be updated with: {x->0, y->0, w->width,
|
|
h->height }.
|
|
HELP
|
|
|
|
&std_pdb_misc;
|
|
|
|
@inargs = (
|
|
&drawable_arg,
|
|
{ name => 'x', type => 'int32',
|
|
desc => 'x coordinate of upper left corner of update region' },
|
|
{ name => 'y', type => 'int32',
|
|
desc => 'y coordinate of upper left corner of update region' },
|
|
{ name => 'width', type => 'int32',
|
|
desc => 'Width of update region' },
|
|
{ name => 'height', type => 'int32',
|
|
desc => 'Height of update region' }
|
|
);
|
|
|
|
%invoke = ( code => 'gimp_drawable_update (drawable, x, y, width, height);' );
|
|
}
|
|
|
|
sub drawable_mask_bounds {
|
|
$blurb = <<'BLURB';
|
|
Find the bounding box of the current selection in relation to the specified
|
|
drawable.
|
|
BLURB
|
|
|
|
$help = <<'HELP';
|
|
This procedure returns the whether there is a selection. If there is one, the
|
|
upper left and lower righthand corners of its bounding box are returned. These
|
|
coordinates are specified relative to the drawable's origin, and bounded by
|
|
the drawable's extents.
|
|
HELP
|
|
|
|
&std_pdb_misc;
|
|
|
|
@inargs = ( &drawable_arg );
|
|
|
|
@outargs = (
|
|
{ name => 'non_empty', type => 'boolean', init => 1,
|
|
desc => 'TRUE if there is a selection' },
|
|
);
|
|
|
|
my $pos = 1;
|
|
foreach $where ('upper left', 'lower right') {
|
|
foreach (qw(x y)) {
|
|
push @outargs, { name => "$_$pos", type => 'int32',
|
|
desc => "$_ coordinate of the $where corner of
|
|
selection bounds" }
|
|
}
|
|
$pos++;
|
|
}
|
|
|
|
%invoke = (
|
|
code => <<'CODE'
|
|
non_empty = gimp_drawable_mask_bounds (drawable, &x1, &y1, &x2, &y2);
|
|
CODE
|
|
);
|
|
}
|
|
|
|
sub drawable_image {
|
|
$blurb = "Returns the drawable's image.";
|
|
|
|
$help = "This procedure returns the drawable's image.";
|
|
|
|
&std_pdb_misc;
|
|
|
|
@inargs = ( &drawable_arg );
|
|
|
|
@outargs = ( &std_image_arg );
|
|
$outargs[0]->{desc} = "The drawable's image";
|
|
$outargs[0]->{init} = 1;
|
|
|
|
%invoke = (
|
|
code => 'success = (gimage = gimp_item_get_image (GIMP_ITEM (drawable))) != NULL;'
|
|
);
|
|
}
|
|
|
|
sub drawable_type {
|
|
$help = "This procedure returns the drawable's type.";
|
|
|
|
&drawable_prop_proc("the drawable's type", 'type', 'enum GimpImageType',
|
|
'type', "The drawable's type: { %%desc%% }");
|
|
}
|
|
|
|
sub drawable_has_alpha {
|
|
$help = <<'HELP';
|
|
This procedure returns whether the specified drawable has an alpha channel.
|
|
This can only be true for layers, and the associated type will be one of:
|
|
{ RGBA , GRAYA, INDEXEDA }.
|
|
HELP
|
|
|
|
&drawable_prop_proc('non-zero if the drawable has an alpha channel',
|
|
'has_alpha', 'boolean', 'has_alpha',
|
|
'Does the drawable have an alpha channel?');
|
|
}
|
|
|
|
sub drawable_type_with_alpha {
|
|
$help = <<'HELP';
|
|
This procedure returns the drawable's type if an alpha channel were added. If
|
|
the type is currently Gray, for instance, the returned type would be GrayA. If
|
|
the drawable already has an alpha channel, the drawable's type is simply
|
|
returned.
|
|
HELP
|
|
|
|
&drawable_prop_proc("the drawable's type with alpha", 'type_with_alpha',
|
|
'enum GimpImageType (no RGB_GIMAGE, GRAY_GIMAGE,
|
|
INDEXED_GIMAGE)', 'type_with_alpha',
|
|
"The drawable's type with alpha: { %%desc%% }");
|
|
}
|
|
|
|
sub drawable_is_rgb {
|
|
&drawable_type_proc('an RGB', 'RGB, RGBA', 'is_rgb');
|
|
}
|
|
|
|
sub drawable_is_gray {
|
|
&drawable_type_proc('a grayscale', 'Gray, GrayA', 'is_gray');
|
|
}
|
|
|
|
sub drawable_is_indexed {
|
|
&drawable_type_proc('an indexed', 'Indexed, IndexedA', 'is_indexed');
|
|
}
|
|
|
|
sub drawable_bytes {
|
|
$help = <<'HELP';
|
|
This procedure returns the number of bytes per pixel (or the number of
|
|
channels) for the specified drawable.
|
|
HELP
|
|
|
|
&drawable_prop_proc('the bytes per pixel', 'bytes', 'int32', 'bytes',
|
|
'Bytes per pixel');
|
|
}
|
|
|
|
sub drawable_width {
|
|
$help = "This procedure returns the specified drawable's width in pixels.";
|
|
|
|
&drawable_prop_proc('the width of the drawable', 'width', 'int32',
|
|
'width', 'Width of drawable');
|
|
}
|
|
|
|
sub drawable_height {
|
|
$help = "This procedure returns the specified drawable's height in pixels.";
|
|
|
|
&drawable_prop_proc('the height of the drawable', 'height', 'int32',
|
|
'height', 'Height of drawable');
|
|
}
|
|
|
|
sub drawable_offsets {
|
|
$blurb = 'Returns the offsets for the drawable.';
|
|
|
|
$help = <<'HELP';
|
|
This procedure returns the specified drawable's offsets. This only makes sense
|
|
if the drawable is a layer since channels are anchored. The offsets of a
|
|
channel will be returned as 0.
|
|
HELP
|
|
|
|
&std_pdb_misc;
|
|
|
|
@inargs = ( &drawable_arg );
|
|
|
|
foreach (qw(x y)) {
|
|
push @outargs, { name => "offset_$_", type => 'int32',
|
|
desc => "$_ offset of drawable", void_ret => 1 }
|
|
}
|
|
|
|
%invoke = ( code => 'gimp_drawable_offsets (drawable, &offset_x, &offset_y);' );
|
|
}
|
|
|
|
sub drawable_is_layer {
|
|
&drawable_is_proc('layer', 'GIMP_IS_LAYER');
|
|
}
|
|
|
|
sub drawable_is_layer_mask {
|
|
&drawable_is_proc('layer mask', 'GIMP_IS_LAYER_MASK');
|
|
}
|
|
|
|
sub drawable_is_channel {
|
|
&drawable_is_proc('channel', 'GIMP_IS_CHANNEL');
|
|
}
|
|
|
|
sub drawable_get_pixel {
|
|
$blurb = 'Gets the value of the pixel at the specified coordinates.';
|
|
|
|
$help = <<'HELP';
|
|
This procedure gets the pixel value at the specified coordinates. The
|
|
'num_channels' argument must always be equal to the bytes-per-pixel value for
|
|
the specified drawable.
|
|
HELP
|
|
|
|
&std_pdb_misc;
|
|
$date = '1997';
|
|
|
|
&drawable_coord_args();
|
|
|
|
@outargs = ( &pixel_arg );
|
|
$outargs[0]->{init} = $outargs[0]->{array}->{init} = 1;
|
|
|
|
%invoke = (
|
|
vars => [ 'guint8 *p', 'gint b', 'Tile *tile' ],
|
|
code => <<'CODE'
|
|
{
|
|
if (x < gimp_drawable_width (drawable) && y < gimp_drawable_height (drawable))
|
|
{
|
|
num_channels = gimp_drawable_bytes (drawable);
|
|
pixel = g_new (guint8, num_channels);
|
|
|
|
tile = tile_manager_get_tile (gimp_drawable_data (drawable), x, y,
|
|
TRUE, TRUE);
|
|
|
|
x %= TILE_WIDTH;
|
|
y %= TILE_HEIGHT;
|
|
|
|
p = tile_data_pointer (tile, x, y);
|
|
for (b = 0; b < num_channels; b++)
|
|
pixel[b] = p[b];
|
|
|
|
tile_release (tile, FALSE);
|
|
}
|
|
else
|
|
success = FALSE;
|
|
}
|
|
CODE
|
|
);
|
|
}
|
|
|
|
sub drawable_set_pixel {
|
|
$blurb = 'Sets the value of the pixel at the specified coordinates.';
|
|
|
|
$help = <<'HELP';
|
|
This procedure sets the pixel value at the specified coordinates. The
|
|
'num_channels' argument must always be equal to the bytes-per-pixel value for
|
|
the spec ified drawable.
|
|
HELP
|
|
|
|
&std_pdb_misc;
|
|
$date = '1997';
|
|
|
|
&drawable_coord_args;
|
|
push @inargs, &pixel_arg;
|
|
|
|
%invoke = (
|
|
vars => [ 'guint8 *p', 'gint b', 'Tile *tile' ],
|
|
code => <<'CODE'
|
|
{
|
|
if (x < gimp_drawable_width (drawable) &&
|
|
y < gimp_drawable_height (drawable) &&
|
|
num_channels == gimp_drawable_bytes (drawable))
|
|
{
|
|
tile = tile_manager_get_tile (gimp_drawable_data (drawable), x, y,
|
|
TRUE, TRUE);
|
|
|
|
x %= TILE_WIDTH;
|
|
y %= TILE_HEIGHT;
|
|
|
|
p = tile_data_pointer (tile, x, y);
|
|
for (b = 0; b < num_channels; b++)
|
|
*p++ = *pixel++;
|
|
|
|
tile_release (tile, TRUE);
|
|
}
|
|
else
|
|
success = FALSE;
|
|
}
|
|
CODE
|
|
);
|
|
}
|
|
|
|
sub drawable_set_image {
|
|
$blurb = 'Set image where drawable belongs to.';
|
|
|
|
$help = <<'HELP';
|
|
Set the image the drawable should be a part of (Use this before adding a
|
|
drawable to another image).
|
|
HELP
|
|
|
|
&std_pdb_misc;
|
|
|
|
@inargs = (
|
|
&drawable_arg,
|
|
&std_image_arg
|
|
);
|
|
|
|
%invoke = ( code => 'gimp_item_set_image (GIMP_ITEM (drawable), gimage);' );
|
|
}
|
|
|
|
|
|
sub dim_args () {
|
|
my @args;
|
|
foreach (qw(width height bpp)) {
|
|
push @args, { name => $_, type => 'int32', desc => "The previews $_", init => 1 };
|
|
}
|
|
@args;
|
|
}
|
|
|
|
sub drawable_thumbnail {
|
|
$blurb = 'Get a thumbnail of a drawable.';
|
|
|
|
$help = <<'HELP';
|
|
This function gets data from which a thumbnail of a drawable preview can be
|
|
created. Maximum x or y dimension is 128 pixels. The pixels are returned
|
|
in the RGB[A] format. The bpp return value gives the number of bytes in
|
|
the image. The alpha channel also returned if the drawable has one.
|
|
HELP
|
|
|
|
$author = $copyright = 'Andy Thomas';
|
|
$date = '1999';
|
|
|
|
@inargs = (
|
|
&drawable_arg,
|
|
{ name => 'width', type => '0 < int32',
|
|
desc => 'The thumbnail width',
|
|
alias => 'req_width' },
|
|
{ name => 'height', type => '0 < int32',
|
|
desc => 'The thumbnail height',
|
|
alias => 'req_height' }
|
|
);
|
|
|
|
@outargs = (
|
|
&dim_args,
|
|
{ name => 'thumbnail_data', type => 'int8array',
|
|
desc => 'The thumbnail data', init => 1, wrap => 1,
|
|
array => { name => 'thumbnail_data_count',
|
|
desc => 'The number of pixels in thumbnail data',
|
|
alias => 'num_pixels', init => 1 } }
|
|
);
|
|
$outargs[0]->{void_ret} = 1;
|
|
|
|
%invoke = (
|
|
headers => [ qw(<string.h> "core/gimplayer.h") ],
|
|
code => <<'CODE'
|
|
{
|
|
TempBuf * buf;
|
|
gint dwidth, dheight;
|
|
|
|
if (req_width <= 128 && req_height <= 128)
|
|
{
|
|
/* Adjust the width/height ratio */
|
|
dwidth = gimp_drawable_width (GIMP_DRAWABLE (drawable));
|
|
dheight = gimp_drawable_height (GIMP_DRAWABLE (drawable));
|
|
|
|
if (dwidth > dheight)
|
|
req_height = (req_width * dheight) / dwidth;
|
|
else
|
|
req_width = (req_height * dwidth) / dheight;
|
|
|
|
buf = gimp_viewable_get_preview (GIMP_VIEWABLE (drawable),
|
|
req_width, req_height);
|
|
|
|
num_pixels = buf->height * buf->width * buf->bytes;
|
|
thumbnail_data = g_new (guint8, num_pixels);
|
|
g_memmove (thumbnail_data, temp_buf_data (buf), num_pixels);
|
|
width = buf->width;
|
|
height = buf->height;
|
|
bpp = buf->bytes;
|
|
}
|
|
}
|
|
CODE
|
|
);
|
|
}
|
|
|
|
sub drawable_offset {
|
|
$blurb = <<'BLURB';
|
|
Offset the drawable by the specified amounts in the X and Y directions
|
|
BLURB
|
|
|
|
$help = <<'HELP';
|
|
This procedure offsets the specified drawable by the amounts specified by
|
|
'offset_x' and 'offset_y'. If 'wrap_around' is set to TRUE, then portions of
|
|
the drawable which are offset out of bounds are wrapped around. Alternatively,
|
|
the undefined regions of the drawable can be filled with transparency or the
|
|
background color, as specified by the 'fill_type' parameter.
|
|
HELP
|
|
|
|
&std_pdb_misc;
|
|
$date = '1997';
|
|
|
|
@inargs = (
|
|
{ name => 'drawable', type => 'drawable',
|
|
desc => 'The drawable to offset' },
|
|
{ name => 'wrap_around', type => 'boolean',
|
|
desc => 'wrap image around or fill vacated regions' },
|
|
{ name => 'fill_type', type => 'enum GimpOffsetType',
|
|
desc => 'fill vacated regions of drawable with background or
|
|
transparent: %%desc%%' },
|
|
{ name => 'offset_x', type => 'int32',
|
|
desc => 'offset by this amount in X direction' },
|
|
{ name => 'offset_y', type => 'int32',
|
|
desc => 'offset by this amount in Y direction' }
|
|
);
|
|
|
|
%invoke = (
|
|
code => <<'CODE'
|
|
{
|
|
gimp_drawable_offset (drawable, wrap_around, fill_type, offset_x, offset_y);
|
|
}
|
|
CODE
|
|
);
|
|
}
|
|
|
|
@headers = qw("core/gimp.h" "core/gimplayermask.h" "core/gimpchannel.h"
|
|
"core/gimpdrawable.h" "core/gimpdrawable-offset.h"
|
|
"base/tile.h" "base/tile-manager.h" "base/temp-buf.h");
|
|
|
|
@procs = qw(drawable_merge_shadow drawable_fill drawable_update
|
|
drawable_mask_bounds drawable_image drawable_type
|
|
drawable_has_alpha drawable_type_with_alpha drawable_is_rgb
|
|
drawable_is_gray drawable_is_indexed drawable_bytes drawable_width
|
|
drawable_height drawable_offsets drawable_is_layer
|
|
drawable_is_layer_mask drawable_is_channel drawable_get_pixel
|
|
drawable_set_pixel drawable_set_image drawable_thumbnail
|
|
drawable_offset);
|
|
%exports = (app => [@procs], lib => [@procs[0..17,21..22]]);
|
|
|
|
$desc = 'Drawable procedures';
|
|
|
|
1;
|