mirror of
https://gitlab.gnome.org/GNOME/gimp
synced 2024-10-23 13:01:42 +00:00
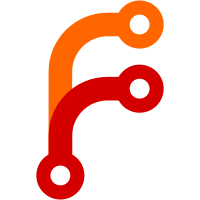
2005-12-29 Simon Budig <simon@gimp.org> * tools/pdbgen/pdb/vectors.pdb: gimp_vectors_[gs]et_locked renamed to _linked for consistency. gimp_vectors_[gs]et_tattoo, gimp_vectors_get_image: new. * app/pdb/internal_procs.c * app/pdb/vectors_cmds.c * libgimp/gimpvectors_pdb.[ch]: regenerated
569 lines
13 KiB
C
569 lines
13 KiB
C
/* LIBGIMP - The GIMP Library
|
|
* Copyright (C) 1995-2003 Peter Mattis and Spencer Kimball
|
|
*
|
|
* gimpvectors_pdb.c
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
/* NOTE: This file is autogenerated by pdbgen.pl */
|
|
|
|
#include "config.h"
|
|
|
|
#include <string.h>
|
|
|
|
#include "gimp.h"
|
|
|
|
/**
|
|
* gimp_vectors_get_strokes:
|
|
* @vectors_ID: The vectors object.
|
|
* @num_strokes: The number of strokes returned.
|
|
*
|
|
* List the strokes associated with the passed path.
|
|
*
|
|
* Returns an Array with the stroke-IDs associated with the passed
|
|
* path.
|
|
*
|
|
* Returns: List of the strokes belonging to the path.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gint *
|
|
gimp_vectors_get_strokes (gint32 vectors_ID,
|
|
gint *num_strokes)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gint *stroke_ids = NULL;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-get-strokes",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_END);
|
|
|
|
*num_strokes = 0;
|
|
|
|
if (return_vals[0].data.d_status == GIMP_PDB_SUCCESS)
|
|
{
|
|
*num_strokes = return_vals[1].data.d_int32;
|
|
stroke_ids = g_new (gint32, *num_strokes);
|
|
memcpy (stroke_ids, return_vals[2].data.d_int32array,
|
|
*num_strokes * sizeof (gint32));
|
|
}
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return stroke_ids;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_get_image:
|
|
* @vectors_ID: The vectors object.
|
|
*
|
|
* Returns the vectors objects image.
|
|
*
|
|
* Returns the vectors objects image.
|
|
*
|
|
* Returns: The vectors image.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gint32
|
|
gimp_vectors_get_image (gint32 vectors_ID)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gint32 image_ID = -1;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-get-image",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_END);
|
|
|
|
if (return_vals[0].data.d_status == GIMP_PDB_SUCCESS)
|
|
image_ID = return_vals[1].data.d_image;
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return image_ID;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_get_linked:
|
|
* @vectors_ID: The vectors object.
|
|
*
|
|
* Gets the linked state of the vectors object.
|
|
*
|
|
* Gets the linked state of the vectors object.
|
|
*
|
|
* Returns: TRUE if the path is linked, FALSE otherwise.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gboolean
|
|
gimp_vectors_get_linked (gint32 vectors_ID)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gboolean linked = FALSE;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-get-linked",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_END);
|
|
|
|
if (return_vals[0].data.d_status == GIMP_PDB_SUCCESS)
|
|
linked = return_vals[1].data.d_int32;
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return linked;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_set_linked:
|
|
* @vectors_ID: The vectors object.
|
|
* @linked: Whether the path is linked.
|
|
*
|
|
* Sets the linked state of the vectors object.
|
|
*
|
|
* Sets the linked state of the vectors object.
|
|
*
|
|
* Returns: TRUE on success.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gboolean
|
|
gimp_vectors_set_linked (gint32 vectors_ID,
|
|
gboolean linked)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gboolean success = TRUE;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-set-linked",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_INT32, linked,
|
|
GIMP_PDB_END);
|
|
|
|
success = return_vals[0].data.d_status == GIMP_PDB_SUCCESS;
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return success;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_get_visible:
|
|
* @vectors_ID: The vectors object.
|
|
*
|
|
* Gets the visibility of the vectors object.
|
|
*
|
|
* Gets the visibility of the vectors object.
|
|
*
|
|
* Returns: TRUE if the path is visible, FALSE otherwise.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gboolean
|
|
gimp_vectors_get_visible (gint32 vectors_ID)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gboolean visible = FALSE;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-get-visible",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_END);
|
|
|
|
if (return_vals[0].data.d_status == GIMP_PDB_SUCCESS)
|
|
visible = return_vals[1].data.d_int32;
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return visible;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_set_visible:
|
|
* @vectors_ID: The vectors object.
|
|
* @visible: Whether the path is visible.
|
|
*
|
|
* Sets the visibility of the vectors object.
|
|
*
|
|
* Sets the visibility of the vectors object.
|
|
*
|
|
* Returns: TRUE on success.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gboolean
|
|
gimp_vectors_set_visible (gint32 vectors_ID,
|
|
gboolean visible)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gboolean success = TRUE;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-set-visible",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_INT32, visible,
|
|
GIMP_PDB_END);
|
|
|
|
success = return_vals[0].data.d_status == GIMP_PDB_SUCCESS;
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return success;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_get_name:
|
|
* @vectors_ID: The vectors object.
|
|
*
|
|
* Gets the name of the vectors object.
|
|
*
|
|
* Gets the name of the vectors object.
|
|
*
|
|
* Returns: The name of the vectors object.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gchar *
|
|
gimp_vectors_get_name (gint32 vectors_ID)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gchar *name = NULL;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-get-name",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_END);
|
|
|
|
if (return_vals[0].data.d_status == GIMP_PDB_SUCCESS)
|
|
name = g_strdup (return_vals[1].data.d_string);
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return name;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_set_name:
|
|
* @vectors_ID: The vectors object.
|
|
* @name: the new name of the path.
|
|
*
|
|
* Sets the name of the vectors object.
|
|
*
|
|
* Sets the name of the vectors object.
|
|
*
|
|
* Returns: TRUE on success.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gboolean
|
|
gimp_vectors_set_name (gint32 vectors_ID,
|
|
const gchar *name)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gboolean success = TRUE;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-set-name",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_STRING, name,
|
|
GIMP_PDB_END);
|
|
|
|
success = return_vals[0].data.d_status == GIMP_PDB_SUCCESS;
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return success;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_get_tattoo:
|
|
* @vectors_ID: The vectors object.
|
|
*
|
|
* Get the tattoo of the vectors object.
|
|
*
|
|
* Get the tattoo state of the vectors object.
|
|
*
|
|
* Returns: The vectors tattoo.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gint
|
|
gimp_vectors_get_tattoo (gint32 vectors_ID)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gint tattoo = 0;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-get-tattoo",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_END);
|
|
|
|
if (return_vals[0].data.d_status == GIMP_PDB_SUCCESS)
|
|
tattoo = return_vals[1].data.d_int32;
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return tattoo;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_set_tattoo:
|
|
* @vectors_ID: The vectors object.
|
|
* @tattoo: the new tattoo.
|
|
*
|
|
* Set the tattoo of the vectors object.
|
|
*
|
|
* Set the tattoo of the vectors object.
|
|
*
|
|
* Returns: TRUE on success.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gboolean
|
|
gimp_vectors_set_tattoo (gint32 vectors_ID,
|
|
gint tattoo)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gboolean success = TRUE;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-set-tattoo",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_INT32, tattoo,
|
|
GIMP_PDB_END);
|
|
|
|
success = return_vals[0].data.d_status == GIMP_PDB_SUCCESS;
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return success;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_stroke_get_length:
|
|
* @vectors_ID: The vectors object.
|
|
* @stroke_id: The stroke ID.
|
|
* @prescision: The prescision used for the approximation.
|
|
*
|
|
* measures the length of the given stroke.
|
|
*
|
|
* Measure the length of the given stroke.
|
|
*
|
|
* Returns: The length (in pixels) of the given stroke.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gdouble
|
|
gimp_vectors_stroke_get_length (gint32 vectors_ID,
|
|
gint stroke_id,
|
|
gdouble prescision)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gdouble length = 0;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-stroke-get-length",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_INT32, stroke_id,
|
|
GIMP_PDB_FLOAT, prescision,
|
|
GIMP_PDB_END);
|
|
|
|
if (return_vals[0].data.d_status == GIMP_PDB_SUCCESS)
|
|
length = return_vals[1].data.d_float;
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return length;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_stroke_remove:
|
|
* @vectors_ID: The vectors object.
|
|
* @stroke_id: The stroke ID.
|
|
*
|
|
* remove the stroke from a vectors object.
|
|
*
|
|
* Remove the stroke from a vectors object.
|
|
*
|
|
* Returns: TRUE on success.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gboolean
|
|
gimp_vectors_stroke_remove (gint32 vectors_ID,
|
|
gint stroke_id)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gboolean success = TRUE;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-stroke-remove",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_INT32, stroke_id,
|
|
GIMP_PDB_END);
|
|
|
|
success = return_vals[0].data.d_status == GIMP_PDB_SUCCESS;
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return success;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_stroke_translate:
|
|
* @vectors_ID: The vectors object.
|
|
* @stroke_id: The stroke ID.
|
|
* @off_x: Offset in x direction.
|
|
* @off_y: Offset in y direction.
|
|
*
|
|
* translate the given stroke.
|
|
*
|
|
* Translate the given stroke.
|
|
*
|
|
* Returns: TRUE on success.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gboolean
|
|
gimp_vectors_stroke_translate (gint32 vectors_ID,
|
|
gint stroke_id,
|
|
gint off_x,
|
|
gint off_y)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gboolean success = TRUE;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-stroke-translate",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_INT32, stroke_id,
|
|
GIMP_PDB_INT32, off_x,
|
|
GIMP_PDB_INT32, off_y,
|
|
GIMP_PDB_END);
|
|
|
|
success = return_vals[0].data.d_status == GIMP_PDB_SUCCESS;
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return success;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_stroke_scale:
|
|
* @vectors_ID: The vectors object.
|
|
* @stroke_id: The stroke ID.
|
|
* @scale_x: Scale factor in x direction.
|
|
* @scale_y: Scale factor in y direction.
|
|
*
|
|
* scales the given stroke.
|
|
*
|
|
* Scale the given stroke.
|
|
*
|
|
* Returns: TRUE on success.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gboolean
|
|
gimp_vectors_stroke_scale (gint32 vectors_ID,
|
|
gint stroke_id,
|
|
gdouble scale_x,
|
|
gdouble scale_y)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gboolean success = TRUE;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-stroke-scale",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_INT32, stroke_id,
|
|
GIMP_PDB_FLOAT, scale_x,
|
|
GIMP_PDB_FLOAT, scale_y,
|
|
GIMP_PDB_END);
|
|
|
|
success = return_vals[0].data.d_status == GIMP_PDB_SUCCESS;
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return success;
|
|
}
|
|
|
|
/**
|
|
* gimp_vectors_stroke_interpolate:
|
|
* @vectors_ID: The vectors object.
|
|
* @stroke_id: The stroke ID.
|
|
* @prescision: The prescision used for the approximation.
|
|
* @num_coords: The number of floats returned.
|
|
* @coords: List of the coords along the path (x0, y0, x1, y1, ...).
|
|
*
|
|
* returns polygonal approximation of the stroke.
|
|
*
|
|
* returns polygonal approximation of the stroke.
|
|
*
|
|
* Returns: List of the strokes belonging to the path.
|
|
*
|
|
* Since: GIMP 2.4
|
|
*/
|
|
gboolean
|
|
gimp_vectors_stroke_interpolate (gint32 vectors_ID,
|
|
gint stroke_id,
|
|
gdouble prescision,
|
|
gint *num_coords,
|
|
gdouble **coords)
|
|
{
|
|
GimpParam *return_vals;
|
|
gint nreturn_vals;
|
|
gboolean closed = FALSE;
|
|
|
|
return_vals = gimp_run_procedure ("gimp-vectors-stroke-interpolate",
|
|
&nreturn_vals,
|
|
GIMP_PDB_PATH, vectors_ID,
|
|
GIMP_PDB_INT32, stroke_id,
|
|
GIMP_PDB_FLOAT, prescision,
|
|
GIMP_PDB_END);
|
|
|
|
*num_coords = 0;
|
|
|
|
if (return_vals[0].data.d_status == GIMP_PDB_SUCCESS)
|
|
{
|
|
closed = return_vals[1].data.d_int32;
|
|
*num_coords = return_vals[2].data.d_int32;
|
|
*coords = g_new (gdouble, *num_coords);
|
|
memcpy (*coords, return_vals[3].data.d_floatarray,
|
|
*num_coords * sizeof (gdouble));
|
|
}
|
|
|
|
gimp_destroy_params (return_vals, nreturn_vals);
|
|
|
|
return closed;
|
|
}
|