mirror of
https://gitlab.gnome.org/GNOME/gimp
synced 2024-10-21 20:12:30 +00:00
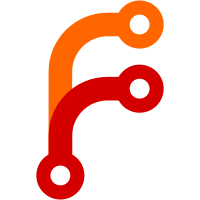
2007-04-25 Michael Natterer <mitch@gimp.org> * app/core/gimpparamspecs.[ch] (struct GimpParamSpecString) (gimp_param_spec_string): added "gboolean non_empty" to require the string being non-empty. Changed validation accordingly. Also fixed validation for static strings (we were happily freeing and modifying them before). * app/xcf/xcf.c: filenames should be non-empty. * app/pdb/gimp-pdb-compat.c: compat strings shouldn't. * tools/pdbgen/app.pl: add support for $arg->{non_empty} and changed generation of calls to gimp_param_spec_string(). * tools/pdbgen/pdb/brush_select.pdb * tools/pdbgen/pdb/edit.pdb * tools/pdbgen/pdb/vectors.pdb * tools/pdbgen/pdb/plug_in.pdb * tools/pdbgen/pdb/gradient.pdb * tools/pdbgen/pdb/palette_select.pdb * tools/pdbgen/pdb/palette.pdb * tools/pdbgen/pdb/fileops.pdb * tools/pdbgen/pdb/progress.pdb * tools/pdbgen/pdb/procedural_db.pdb * tools/pdbgen/pdb/font_select.pdb * tools/pdbgen/pdb/pattern_select.pdb * tools/pdbgen/pdb/unit.pdb * tools/pdbgen/pdb/brush.pdb * tools/pdbgen/pdb/gradient_select.pdb * tools/pdbgen/pdb/buffer.pdb: require non-empty strings for data object names, procedure names, unit strings, PDB data identifiers and buffer names. Removed some manual strlen() checks, all other places just got better error reporting for free (proper validation error instead of unspecific execution error). * app/pdb/*_cmds.c: regenerated. svn path=/trunk/; revision=22329
213 lines
9.1 KiB
C
213 lines
9.1 KiB
C
/* GIMP - The GNU Image Manipulation Program
|
|
* Copyright (C) 1995-2003 Spencer Kimball and Peter Mattis
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
/* NOTE: This file is auto-generated by pdbgen.pl. */
|
|
|
|
#include "config.h"
|
|
|
|
|
|
#include <glib-object.h>
|
|
|
|
#include "pdb-types.h"
|
|
#include "gimppdb.h"
|
|
#include "gimpprocedure.h"
|
|
#include "core/gimpparamspecs.h"
|
|
|
|
#include "core/gimp.h"
|
|
#include "core/gimpdatafactory.h"
|
|
|
|
#include "internal_procs.h"
|
|
|
|
|
|
static GValueArray *
|
|
palettes_popup_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
const gchar *palette_callback;
|
|
const gchar *popup_title;
|
|
const gchar *initial_palette;
|
|
|
|
palette_callback = g_value_get_string (&args->values[0]);
|
|
popup_title = g_value_get_string (&args->values[1]);
|
|
initial_palette = g_value_get_string (&args->values[2]);
|
|
|
|
if (success)
|
|
{
|
|
if (gimp->no_interface ||
|
|
! gimp_pdb_lookup_procedure (gimp->pdb, palette_callback) ||
|
|
! gimp_pdb_dialog_new (gimp, context, progress,
|
|
gimp->palette_factory->container,
|
|
popup_title, palette_callback, initial_palette,
|
|
NULL))
|
|
success = FALSE;
|
|
}
|
|
|
|
return gimp_procedure_get_return_values (procedure, success);
|
|
}
|
|
|
|
static GValueArray *
|
|
palettes_close_popup_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
const gchar *palette_callback;
|
|
|
|
palette_callback = g_value_get_string (&args->values[0]);
|
|
|
|
if (success)
|
|
{
|
|
if (gimp->no_interface ||
|
|
! gimp_pdb_lookup_procedure (gimp->pdb, palette_callback) ||
|
|
! gimp_pdb_dialog_close (gimp, gimp->palette_factory->container,
|
|
palette_callback))
|
|
success = FALSE;
|
|
}
|
|
|
|
return gimp_procedure_get_return_values (procedure, success);
|
|
}
|
|
|
|
static GValueArray *
|
|
palettes_set_popup_invoker (GimpProcedure *procedure,
|
|
Gimp *gimp,
|
|
GimpContext *context,
|
|
GimpProgress *progress,
|
|
const GValueArray *args)
|
|
{
|
|
gboolean success = TRUE;
|
|
const gchar *palette_callback;
|
|
const gchar *palette_name;
|
|
|
|
palette_callback = g_value_get_string (&args->values[0]);
|
|
palette_name = g_value_get_string (&args->values[1]);
|
|
|
|
if (success)
|
|
{
|
|
if (gimp->no_interface ||
|
|
! gimp_pdb_lookup_procedure (gimp->pdb, palette_callback) ||
|
|
! gimp_pdb_dialog_set (gimp, gimp->palette_factory->container,
|
|
palette_callback, palette_name,
|
|
NULL))
|
|
success = FALSE;
|
|
}
|
|
|
|
return gimp_procedure_get_return_values (procedure, success);
|
|
}
|
|
|
|
void
|
|
register_palette_select_procs (GimpPDB *pdb)
|
|
{
|
|
GimpProcedure *procedure;
|
|
|
|
/*
|
|
* gimp-palettes-popup
|
|
*/
|
|
procedure = gimp_procedure_new (palettes_popup_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-palettes-popup");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-palettes-popup",
|
|
"Invokes the Gimp palette selection.",
|
|
"This procedure opens the palette selection dialog.",
|
|
"Michael Natterer <mitch@gimp.org>",
|
|
"Michael Natterer",
|
|
"2002",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("palette-callback",
|
|
"palette callback",
|
|
"The callback PDB proc to call when palette selection is made",
|
|
FALSE, FALSE, TRUE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("popup-title",
|
|
"popup title",
|
|
"Title of the palette selection dialog",
|
|
FALSE, FALSE, FALSE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("initial-palette",
|
|
"initial palette",
|
|
"The name of the palette to set as the first selected",
|
|
FALSE, TRUE, FALSE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-palettes-close-popup
|
|
*/
|
|
procedure = gimp_procedure_new (palettes_close_popup_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-palettes-close-popup");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-palettes-close-popup",
|
|
"Close the palette selection dialog.",
|
|
"This procedure closes an opened palette selection dialog.",
|
|
"Michael Natterer <mitch@gimp.org>",
|
|
"Michael Natterer",
|
|
"2002",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("palette-callback",
|
|
"palette callback",
|
|
"The name of the callback registered for this pop-up",
|
|
FALSE, FALSE, TRUE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
|
|
/*
|
|
* gimp-palettes-set-popup
|
|
*/
|
|
procedure = gimp_procedure_new (palettes_set_popup_invoker);
|
|
gimp_object_set_static_name (GIMP_OBJECT (procedure), "gimp-palettes-set-popup");
|
|
gimp_procedure_set_static_strings (procedure,
|
|
"gimp-palettes-set-popup",
|
|
"Sets the current palette in a palette selection dialog.",
|
|
"Sets the current palette in a palette selection dialog.",
|
|
"Michael Natterer <mitch@gimp.org>",
|
|
"Michael Natterer",
|
|
"2002",
|
|
NULL);
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("palette-callback",
|
|
"palette callback",
|
|
"The name of the callback registered for this pop-up",
|
|
FALSE, FALSE, TRUE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_procedure_add_argument (procedure,
|
|
gimp_param_spec_string ("palette-name",
|
|
"palette name",
|
|
"The name of the palette to set as selected",
|
|
FALSE, FALSE, FALSE,
|
|
NULL,
|
|
GIMP_PARAM_READWRITE));
|
|
gimp_pdb_register_procedure (pdb, procedure);
|
|
g_object_unref (procedure);
|
|
}
|