mirror of
https://gitlab.gnome.org/GNOME/gimp
synced 2024-10-20 19:43:01 +00:00
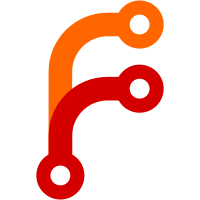
2002-09-01 Michael Natterer <mitch@gimp.org> GimpViewableDialogs everywhere, cleanup: * libgimpwidgets/gimpstock.c: added texts for the RESIZE, SCALE and CROP stock items. * app/widgets/gimpviewabledialog.c: update the title when the viewable's name changes. * app/gui/color-notebook.[ch]: added color_notebook_viewable_new() which creates a GimpViewableDialog. * app/widgets/gimpgradienteditor.[ch] * app/gui/colormap-editor-commands.c * app/gui/file-new-dialog.c * app/gui/gradient-editor-commands.c * app/gui/palette-editor-commands.c * app/undo_history.c: use GimpViewableDialogs and the new color_notebook constructor. * app/gui/convert-dialog.c: #include "widgets/gimpviewabledialog.h" * app/gui/image-commands.c * app/gui/info-dialog.c * app/gui/resize-dialog.c: minor cleanups. * app/gui/info-window.c: cleaned up the whole thing, esp. the "Extended" page. Added HSV color display to the color picker frame. Set the icons as frame titles, stuff... * app/tools/gimpimagemaptool.[ch]: removed "shell_title", "shell_name" and "stock_id" from the GimpImageMapTool struct because they can be obtained from the tool's GimpToolInfo object. * app/tools/gimpbrightnesscontrasttool.c * app/tools/gimpcolorbalancetool.c * app/tools/gimpcurvestool.c * app/tools/gimphuesaturationtool.c * app/tools/gimplevelstool.c * app/tools/gimpposterizetool.c * app/tools/gimpthresholdtool.c: changed accordingly. * app/tools/gimphistogramtool.c: same here: take values from tool->tool_info instead of hardcoding them. * app/tools/gimpcroptool.[ch]: removed the static crop dialog variables and added them to the GimpCropTool struct. Feels safer and makes the callback code much simpler. Use stock items for the dialog's "Resize" and "Crop" buttons. * app/tools/gimpmeasuretool.c * app/tools/gimprotatetool.c: for consistency don't name the tools "Blah Tool", also the dialog titles need to match the menu entries. Unrelated: * libgimpwidgets/gimpwidgets.c: the recently changed, gtk-doc comment was correct, as gtk-doc takes the parameter names from the header, not the .c file. * app/tools/gimptransformtool.c: set the transform tool's state to TRANSFORM_CREATING after changing displays, so the initial matrix components are saved correctly for the "Reset" function.
131 lines
4.3 KiB
C
131 lines
4.3 KiB
C
/* The GIMP -- an image manipulation program
|
|
* Copyright (C) 1995 Spencer Kimball and Peter Mattis
|
|
*
|
|
* Gradient editor module copyight (C) 1996-1997 Federico Mena Quintero
|
|
* federico@nuclecu.unam.mx
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef __GIMP_GRADIENT_EDITOR_H__
|
|
#define __GIMP_GRADIENT_EDITOR_H__
|
|
|
|
|
|
#include "gimpdataeditor.h"
|
|
|
|
#include "gui/gui-types.h" /* FIXME */
|
|
|
|
|
|
#define GRAD_NUM_COLORS 10
|
|
|
|
|
|
typedef enum
|
|
{
|
|
GRAD_DRAG_NONE = 0,
|
|
GRAD_DRAG_LEFT,
|
|
GRAD_DRAG_MIDDLE,
|
|
GRAD_DRAG_ALL
|
|
} GradientEditorDragMode;
|
|
|
|
typedef enum
|
|
{
|
|
GRAD_UPDATE_GRADIENT = 1 << 0,
|
|
GRAD_UPDATE_PREVIEW = 1 << 1,
|
|
GRAD_UPDATE_CONTROL = 1 << 2,
|
|
GRAD_RESET_CONTROL = 1 << 3
|
|
} GradientEditorUpdateMask;
|
|
|
|
|
|
#define GIMP_TYPE_GRADIENT_EDITOR (gimp_gradient_editor_get_type ())
|
|
#define GIMP_GRADIENT_EDITOR(obj) (G_TYPE_CHECK_INSTANCE_CAST ((obj), GIMP_TYPE_GRADIENT_EDITOR, GimpGradientEditor))
|
|
#define GIMP_GRADIENT_EDITOR_CLASS(klass) (G_TYPE_CHECK_CLASS_CAST ((klass), GIMP_TYPE_GRADIENT_EDITOR, GimpGradientEditorClass))
|
|
#define GIMP_IS_GRADIENT_EDITOR(obj) (G_TYPE_CHECK_INSTANCE_TYPE ((obj), GIMP_TYPE_GRADIENT_EDITOR))
|
|
#define GIMP_IS_GRADIENT_EDITOR_CLASS(klass) (G_TYPE_CHECK_CLASS_TYPE ((klass), GIMP_TYPE_GRADIENT_EDITOR))
|
|
#define GIMP_GRADIENT_EDITOR_GET_CLASS(obj) (G_TYPE_INSTANCE_GET_CLASS ((obj), GIMP_TYPE_GRADIENT_EDITOR, GimpGradientEditorClass))
|
|
|
|
|
|
typedef struct _GimpGradientEditorClass GimpGradientEditorClass;
|
|
|
|
struct _GimpGradientEditor
|
|
{
|
|
GimpDataEditor parent_instance;
|
|
|
|
GtkWidget *hint_label1;
|
|
GtkWidget *hint_label2;
|
|
GtkWidget *hint_label3;
|
|
GtkWidget *scrollbar;
|
|
GtkWidget *preview;
|
|
GtkWidget *control;
|
|
|
|
/* Zoom and scrollbar */
|
|
guint zoom_factor;
|
|
GtkObject *scroll_data;
|
|
|
|
/* Instant update */
|
|
gboolean instant_update;
|
|
|
|
/* Color notebook */
|
|
ColorNotebook *color_notebook;
|
|
|
|
/* Gradient preview */
|
|
guchar *preview_rows[2]; /* For caching redraw info */
|
|
gint preview_last_x;
|
|
gboolean preview_button_down;
|
|
|
|
/* Gradient control */
|
|
GdkPixmap *control_pixmap;
|
|
GimpGradientSegment *control_drag_segment; /* Segment which is being dragged */
|
|
GimpGradientSegment *control_sel_l; /* Left segment of selection */
|
|
GimpGradientSegment *control_sel_r; /* Right segment of selection */
|
|
GradientEditorDragMode control_drag_mode; /* What is being dragged? */
|
|
guint32 control_click_time; /* Time when mouse was pressed */
|
|
gboolean control_compress; /* Compressing/expanding handles */
|
|
gint control_last_x; /* Last mouse position when dragging */
|
|
gdouble control_last_gx; /* Last position (wrt gradient) when dragging */
|
|
gdouble control_orig_pos; /* Original click position when dragging */
|
|
|
|
/* Split uniformly dialog */
|
|
gint split_parts;
|
|
|
|
/* Replicate dialog */
|
|
gint replicate_times;
|
|
|
|
/* Saved colors */
|
|
GimpRGB saved_colors[GRAD_NUM_COLORS];
|
|
|
|
/* Color dialogs */
|
|
GimpGradientSegment *left_saved_segments;
|
|
gboolean left_saved_dirty;
|
|
|
|
GimpGradientSegment *right_saved_segments;
|
|
gboolean right_saved_dirty;
|
|
};
|
|
|
|
struct _GimpGradientEditorClass
|
|
{
|
|
GimpDataEditorClass parent_class;
|
|
};
|
|
|
|
|
|
GType gimp_gradient_editor_get_type (void) G_GNUC_CONST;
|
|
|
|
GimpDataEditor * gimp_gradient_editor_new (Gimp *gimp);
|
|
|
|
void gimp_gradient_editor_update (GimpGradientEditor *editor,
|
|
GradientEditorUpdateMask flags);
|
|
|
|
|
|
#endif /* __GIMP_GRADIENT_EDITOR_H__ */
|