mirror of
https://gitlab.gnome.org/GNOME/gimp
synced 2024-10-20 19:43:01 +00:00
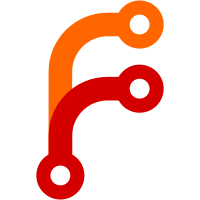
* app/*.[ch]: Actually use the enum types GimpImageType, GimpImageBaseType, LayerModeEffects, PaintApplicationMode, BrushApplicationMode, GimpFillType and ConvertPaletteType, instead of just int or gint. Hopefully I catched most of the places where these should be used. Add an enum ConvolutionType, suffix the too general constants NORMAL, ABSOLUTE and NEGATIVE with _CONVOL. Use NORMAL_MODE instead of NORMAL in some places (this was what was intended). Fix some minor gccisms. * app/apptypes.h: New file. This file contains the above enumeration types, and some opaque struct typedefs. It was necessary to collect these in one header that doesn't include other headers, because when we started using the above mentioned types in the headers, all hell broke loose because of the spaghetti-like cross-inclusion mess between headers. (An example: Header A includes header B, which includes header C which includes A. B uses a type defined in A. This is not defined, because A hasn't defined it yet at the point where it includes B, and A included from B of course is skipped as we already are reading A.)
149 lines
5.1 KiB
C
149 lines
5.1 KiB
C
/* The GIMP -- an image manipulation program
|
|
* Copyright (C) 1995 Spencer Kimball and Peter Mattis
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
*/
|
|
#ifndef __CHANNEL_H__
|
|
#define __CHANNEL_H__
|
|
|
|
#include "apptypes.h"
|
|
#include "drawable.h"
|
|
#include "boundary.h"
|
|
#include "temp_buf.h"
|
|
#include "tile_manager.h"
|
|
#include "gimpimageF.h"
|
|
|
|
/* OPERATIONS */
|
|
|
|
typedef enum
|
|
{
|
|
ADD,
|
|
SUB,
|
|
REPLACE,
|
|
INTERSECT
|
|
} ChannelOps;
|
|
|
|
/* Half way point where a region is no longer visible in a selection */
|
|
#define HALF_WAY 127
|
|
|
|
/* structure declarations */
|
|
|
|
#define GIMP_TYPE_CHANNEL (gimp_channel_get_type ())
|
|
#define GIMP_CHANNEL(obj) (GTK_CHECK_CAST ((obj), GIMP_TYPE_CHANNEL, GimpChannel))
|
|
#define GIMP_CHANNEL_CLASS(klass) (GTK_CHECK_CLASS_CAST ((klass), GIMP_TYPE_CHANNEL, GimpChannelClass))
|
|
#define GIMP_IS_CHANNEL(obj) (GTK_CHECK_TYPE ((obj), GIMP_TYPE_CHANNEL))
|
|
#define GIMP_IS_CHANNEL_CLASS(klass) (GTK_CHECK_CLASS_TYPE ((klass), GIMP_TYPE_CHANNEL))
|
|
|
|
|
|
GtkType gimp_channel_get_type (void);
|
|
|
|
/* Special undo type */
|
|
typedef struct _channel_undo ChannelUndo;
|
|
|
|
struct _channel_undo
|
|
{
|
|
Channel * channel; /* the actual channel */
|
|
int prev_position; /* former position in list */
|
|
Channel * prev_channel; /* previous active channel */
|
|
int undo_type; /* is this a new channel undo */
|
|
/* or a remove channel undo? */
|
|
};
|
|
|
|
/* Special undo type */
|
|
typedef struct _mask_undo MaskUndo;
|
|
|
|
struct _mask_undo
|
|
{
|
|
TileManager * tiles; /* the actual mask */
|
|
int x, y; /* offsets */
|
|
};
|
|
|
|
|
|
/* function declarations */
|
|
|
|
Channel * channel_new (GimpImage*, int, int, char *, int, unsigned char *);
|
|
Channel * channel_copy (Channel *);
|
|
Channel * channel_ref (Channel *);
|
|
void channel_unref (Channel *);
|
|
|
|
char * channel_get_name (Channel *);
|
|
void channel_set_name (Channel *, char *);
|
|
|
|
int channel_get_opacity (Channel *);
|
|
void channel_set_opacity (Channel *, int );
|
|
|
|
char * channel_get_color (Channel *);
|
|
void channel_set_color (Channel *, gchar *);
|
|
|
|
Channel * channel_get_ID (int);
|
|
void channel_delete (Channel *);
|
|
void channel_scale (Channel *, int, int);
|
|
void channel_resize (Channel *, int, int, int, int);
|
|
void channel_update (Channel *);
|
|
|
|
/* access functions */
|
|
|
|
unsigned char * channel_data (Channel *);
|
|
int channel_toggle_visibility (Channel *);
|
|
TempBuf * channel_preview (Channel *, int, int);
|
|
|
|
void channel_invalidate_previews (GimpImage*);
|
|
Tattoo channel_get_tattoo(const Channel *);
|
|
|
|
|
|
/* selection mask functions */
|
|
|
|
Channel * channel_new_mask (GimpImage*, int, int);
|
|
int channel_boundary (Channel *, BoundSeg **, BoundSeg **,
|
|
int *, int *, int, int, int, int);
|
|
int channel_bounds (Channel *, int *, int *, int *, int *);
|
|
int channel_value (Channel *, int, int);
|
|
int channel_is_empty (Channel *);
|
|
void channel_add_segment (Channel *, int, int, int, int);
|
|
void channel_sub_segment (Channel *, int, int, int, int);
|
|
void channel_inter_segment (Channel *, int, int, int, int);
|
|
void channel_combine_rect (Channel *, int, int, int, int, int);
|
|
void channel_combine_ellipse (Channel *, int, int, int, int, int, int);
|
|
void channel_combine_mask (Channel *, Channel *, int, int, int);
|
|
void channel_feather (Channel *, Channel *,
|
|
double, double,
|
|
int, int, int);
|
|
void channel_push_undo (Channel *);
|
|
void channel_clear (Channel *);
|
|
void channel_invert (Channel *);
|
|
void channel_sharpen (Channel *);
|
|
void channel_all (Channel *);
|
|
|
|
void channel_border (Channel * channel,
|
|
int radius_x,
|
|
int radius_y);
|
|
void channel_grow (Channel * channel,
|
|
int radius_x,
|
|
int radius_y);
|
|
void channel_shrink (Channel * channel,
|
|
int radius_x,
|
|
int radius_y,
|
|
int edge_lock);
|
|
|
|
void channel_translate (Channel *, int, int);
|
|
void channel_load (Channel *, Channel *);
|
|
void channel_invalidate_bounds (Channel *);
|
|
|
|
extern int channel_get_count;
|
|
|
|
#define drawable_channel GIMP_IS_CHANNEL
|
|
|
|
#endif /* __CHANNEL_H__ */
|