mirror of
https://gitlab.gnome.org/GNOME/nautilus
synced 2024-11-05 16:04:31 +00:00
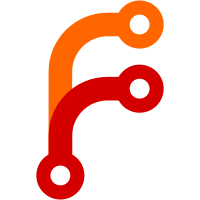
* README: Tell everyone that we are back on bonobo HEAD. * configure.in: Make sure Nautilus doesn't try to compile with the old bonobo. * components/adapter/nautilus-adapter-embed-strategy.c: (nautilus_adapter_embed_strategy_get): * components/adapter/nautilus-adapter-load-strategy.c: (nautilus_adapter_load_strategy_get): * components/adapter/nautilus-adapter-progressive-load-strategy.c: (nautilus_adapter_progressive_load_strategy_load_location): * components/history/nautilus-history-view.c: (history_view_frame_call_begin): * libnautilus/nautilus-undo.c: (set_up_bonobo_control): * libnautilus/nautilus-view.c: (view_frame_call_begin): * libnautilus/nautilus-zoomable.c: (nautilus_zoomable_ensure_zoomable_frame): Fix all the callers of query_interface in CORBA to call it queryInterface (I used the Bonobo script to do it). * src/nautilus-window.h: * src/nautilus-window.c: (nautilus_window_constructed), (nautilus_window_set_arg), (nautilus_window_get_arg), (nautilus_window_set_content_view_widget): Update for BonoboWin -> BonoboWindow change. Once again, the change was done by the Bonobo script (but I had to undo the spaces it ate after BONOBO_WIN). * components/services/install/idl/trilobite-eazel-install.idl: * components/services/time/idl/trilobite-eazel-time-service.idl: * components/services/trilobite/idl/trilobite-service.idl: * components/services/trilobite/sample/idl/sample-service.idl: * libnautilus-adapter/nautilus-adapter-factory.idl: * libnautilus/nautilus-distributed-undo.idl: * libnautilus/nautilus-view-component.idl: Since IDL dependencies don't work, I touched every IDL file to make the switchover smoother. This only works if people update before they try to build and fail, otherwise they need to do: find -name '*.xml' | xargs touch (Maciej and I want to add dependency support to orbit-idl to make this problem go away long term). * src/nautilus-desktop-window.c: (realize): The script updated this comment to, so I let it. * user-guide/C/Makefile.am: Took out the botched change I made to this file. Mathieu did the correct one.
103 lines
3.2 KiB
Text
103 lines
3.2 KiB
Text
/* -*- Mode: IDL; tab-width: 8; indent-tabs-mode: 8; c-basic-offset: 8 -*- */
|
|
|
|
/* nautilus-undo.idl - Interface for view components that
|
|
* support undo, used internally by the
|
|
* undo support classes.
|
|
*
|
|
* Copyright (C) 2000 Eazel, Inc.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Library General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Library General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Library General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef NAUTILUS_UNDO_IDL_INCLUDED
|
|
#define NAUTILUS_UNDO_IDL_INCLUDED
|
|
|
|
#include <Bonobo.idl>
|
|
|
|
module Nautilus {
|
|
|
|
module Undo {
|
|
|
|
/* The specifications for a menu item. */
|
|
struct MenuItem {
|
|
string label;
|
|
string hint;
|
|
};
|
|
|
|
/* A single undoable operation is represented by a
|
|
* transaction. This is the interface a transaction
|
|
* must supply for use by an undo manager.
|
|
*/
|
|
interface Transaction : ::Bonobo::Unknown {
|
|
/* These menu items are used to display undo
|
|
* or redo menu items for this transaction.
|
|
* The operation name is for lists of undoable
|
|
* operations that are listed somewhere other
|
|
* than an undo or redo object.
|
|
*/
|
|
readonly attribute MenuItem undo_menu_item;
|
|
readonly attribute MenuItem redo_menu_item;
|
|
readonly attribute string operation_name;
|
|
|
|
/* Here's how you actually perform an undo.
|
|
* Once it's performed, calling this again is
|
|
* safe; it's guaranteed to do nothing.
|
|
*/
|
|
void undo ();
|
|
};
|
|
|
|
/* An undo manager deals with a list of transactions
|
|
* for a particular application or window. This is the
|
|
* interface of the manager from the transaction's
|
|
* point of view only.
|
|
*/
|
|
interface Manager : ::Bonobo::Unknown {
|
|
/* Add a new transaction. This is normally called
|
|
* by the code that creates the transaction.
|
|
*/
|
|
void append (in Transaction transaction);
|
|
|
|
/* Forget a transaction. This is typically called
|
|
* when the operation that the transaction does
|
|
* undo for no longer makes sense.
|
|
*/
|
|
void forget (in Transaction transaction);
|
|
|
|
/* Sometimes an undo has to be "forced" from the
|
|
* client side when it recognizes an undo key
|
|
* equivalent.
|
|
*/
|
|
void undo ();
|
|
|
|
/* FIXME bugzilla.eazel.com 1292:
|
|
* We may need additional interface so the
|
|
* client can include an appropriate undo item in
|
|
* a contextual menu.
|
|
*/
|
|
};
|
|
|
|
/* To locate the appropriate manager, a view component
|
|
* can query for this interface on its Bonobo control
|
|
* frame. This is done automatically by functions in
|
|
* the undo manager.
|
|
*/
|
|
interface Context : ::Bonobo::Unknown {
|
|
readonly attribute Manager undo_manager;
|
|
};
|
|
};
|
|
};
|
|
|
|
#endif /* NAUTILUS_UNDO_IDL_INCLUDED */
|