mirror of
https://gitlab.gnome.org/GNOME/nautilus
synced 2024-09-20 08:11:56 +00:00
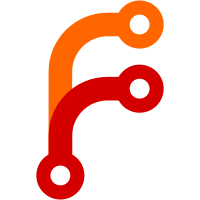
-George Fri Aug 04 14:21:51 2000 George Lebl <jirka@5z.com> * components/hardware/nautilus-hardware-view.c (setup_form_title): Check result of gnome_pixmap_file. If NULL, we can't make a new gnome-pixmap. * components/help/converters/gnome-db2html2/sect-elements.c (sect_get_infobox_logo) (sect_infobox_start_element): sect_get_infobox_logo can return a NULL so check its return and if NULL just add %s instead of <IMG ...> * components/services/vault/command-line/vault-operations.c, libnautilus-extensions/nautilus-list.c: add #include <string.h> * cut-n-paste-code/freetype/ftcalc.h, cut-n-paste-code/freetype/ftconfig.h: Well even though this is cut and paste code I NEED to add this patch for it to even compile on alpha, I'll try to get this upstream. The only changes are inside #ifdefs on platforms where long is 64 bit so it should not affect intel code at all. * libnautilus-extensions/nautilus-file-utilities.c (nautilus_format_uri_for_display): use g_strdup instead of strdup as then g_free is used and this would really confuse glib in memory checking mode. * librsvg/art_render_mask.c, librsvg/rsvg-bpath-util.c, librsvg/rsvg-ft.c: add #include <string.h> * src/Makefile.am: define NAUTILUS_PIXMAPDIR. This is neccessary for both making the tarballs and actual code sanity on switching toolbar themes etc. In GNOME 2.0 the GNOME_PATH can be sanely used to allow relocatable nautilus binary, but in 1.[02] this is the correct way to do things. * src/nautilus-complex-search-bar.c (nautilus_complex_search_bar_initialize) (load_find_them_pixmap_widget): If we can't load pixmaps don't add them. Also don't hardcode "/gnome/share" prefix and use the NAUTILUS_PIXMAPDIR define to find search.png AND make sure we can load it before using it. * src/nautilus-window-toolbars.c (find_toolbar_child) (setup_button) In toolbar_info use the NAUTILUS_PIXMAPDIR define to find pixmaps. remove get_stock_callback and get_stock_widget as those functions were an incredible hack and were likely to break. Replace with a function which searches the toolbar children and then finds correct icon widget. It also doesn't do anything if there is no icon. In setup button, use NAUTILUS_PIXMAPDIR to define pixmaps by their full name. If we cannot set the stock widget icon, then the icon is not registered and we register it with gnome-stock. Miraculously the current code worked but only worked because the eazel theme was set up in the toolbar_info, other themes with outside images would break.
197 lines
4.5 KiB
C
197 lines
4.5 KiB
C
/*
|
|
rsvg-bpath-util.c: Data structure and convenience functions for creating bezier paths.
|
|
|
|
Copyright (C) 2000 Eazel, Inc.
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public License as
|
|
published by the Free Software Foundation; either version 2 of the
|
|
License, or (at your option) any later version.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public
|
|
License along with this program; if not, write to the
|
|
Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
|
|
Author: Raph Levien <raph@artofcode.com>
|
|
*/
|
|
|
|
#include <glib.h>
|
|
#include <math.h>
|
|
#include <string.h>
|
|
#include "rsvg-bpath-util.h"
|
|
|
|
/* This is adapted from gnome-canvas-bpath-util in libgnomeprint
|
|
(originally developed as part of Gill). */
|
|
|
|
RsvgBpathDef *
|
|
rsvg_bpath_def_new (void)
|
|
{
|
|
RsvgBpathDef *bpd;
|
|
|
|
bpd = g_new (RsvgBpathDef, 1);
|
|
bpd->n_bpath = 0;
|
|
bpd->n_bpath_max = 16;
|
|
bpd->moveto_idx = -1;
|
|
bpd->bpath = g_new (ArtBpath, bpd->n_bpath_max);
|
|
bpd->ref_count = 1;
|
|
|
|
return bpd;
|
|
}
|
|
|
|
RsvgBpathDef *
|
|
rsvg_bpath_def_new_from (ArtBpath *path)
|
|
{
|
|
RsvgBpathDef *bpd;
|
|
int i;
|
|
|
|
g_return_val_if_fail (path != NULL, NULL);
|
|
|
|
for (i = 0; path[i].code != ART_END; i++)
|
|
;
|
|
if (i <= 0)
|
|
return rsvg_bpath_def_new ();
|
|
|
|
bpd = g_new (RsvgBpathDef, 1);
|
|
|
|
bpd->n_bpath = i;
|
|
bpd->n_bpath_max = i;
|
|
bpd->moveto_idx = -1;
|
|
bpd->ref_count = 1;
|
|
bpd->bpath = g_new (ArtBpath, i);
|
|
|
|
memcpy (bpd->bpath, path, i * sizeof (ArtBpath));
|
|
return bpd;
|
|
}
|
|
|
|
RsvgBpathDef *
|
|
rsvg_bpath_def_ref (RsvgBpathDef *bpd)
|
|
{
|
|
g_return_val_if_fail (bpd != NULL, NULL);
|
|
|
|
bpd->ref_count += 1;
|
|
return bpd;
|
|
}
|
|
|
|
void
|
|
rsvg_bpath_def_free (RsvgBpathDef *bpd)
|
|
{
|
|
g_return_if_fail (bpd != NULL);
|
|
|
|
bpd->ref_count -= 1;
|
|
if (bpd->ref_count == 0)
|
|
{
|
|
g_free (bpd->bpath);
|
|
g_free (bpd);
|
|
}
|
|
}
|
|
|
|
void
|
|
rsvg_bpath_def_moveto (RsvgBpathDef *bpd, double x, double y)
|
|
{
|
|
ArtBpath *bpath;
|
|
int n_bpath;
|
|
|
|
g_return_if_fail (bpd != NULL);
|
|
|
|
n_bpath = bpd->n_bpath++;
|
|
|
|
if (n_bpath == bpd->n_bpath_max)
|
|
bpd->bpath = g_realloc (bpd->bpath,
|
|
(bpd->n_bpath_max <<= 1) * sizeof (ArtBpath));
|
|
bpath = bpd->bpath;
|
|
bpath[n_bpath].code = ART_MOVETO_OPEN;
|
|
bpath[n_bpath].x3 = x;
|
|
bpath[n_bpath].y3 = y;
|
|
bpd->moveto_idx = n_bpath;
|
|
}
|
|
|
|
void
|
|
rsvg_bpath_def_lineto (RsvgBpathDef *bpd, double x, double y)
|
|
{
|
|
ArtBpath *bpath;
|
|
int n_bpath;
|
|
|
|
g_return_if_fail (bpd != NULL);
|
|
g_return_if_fail (bpd->moveto_idx >= 0);
|
|
|
|
n_bpath = bpd->n_bpath++;
|
|
|
|
if (n_bpath == bpd->n_bpath_max)
|
|
bpd->bpath = g_realloc (bpd->bpath,
|
|
(bpd->n_bpath_max <<= 1) * sizeof (ArtBpath));
|
|
bpath = bpd->bpath;
|
|
bpath[n_bpath].code = ART_LINETO;
|
|
bpath[n_bpath].x3 = x;
|
|
bpath[n_bpath].y3 = y;
|
|
}
|
|
|
|
void
|
|
rsvg_bpath_def_curveto (RsvgBpathDef *bpd, double x1, double y1, double x2, double y2, double x3, double y3)
|
|
{
|
|
ArtBpath *bpath;
|
|
int n_bpath;
|
|
|
|
g_return_if_fail (bpd != NULL);
|
|
g_return_if_fail (bpd->moveto_idx >= 0);
|
|
|
|
n_bpath = bpd->n_bpath++;
|
|
|
|
if (n_bpath == bpd->n_bpath_max)
|
|
bpd->bpath = g_realloc (bpd->bpath,
|
|
(bpd->n_bpath_max <<= 1) * sizeof (ArtBpath));
|
|
bpath = bpd->bpath;
|
|
bpath[n_bpath].code = ART_CURVETO;
|
|
bpath[n_bpath].x1 = x1;
|
|
bpath[n_bpath].y1 = y1;
|
|
bpath[n_bpath].x2 = x2;
|
|
bpath[n_bpath].y2 = y2;
|
|
bpath[n_bpath].x3 = x3;
|
|
bpath[n_bpath].y3 = y3;
|
|
}
|
|
|
|
void
|
|
rsvg_bpath_def_closepath (RsvgBpathDef *bpd)
|
|
{
|
|
ArtBpath *bpath;
|
|
int n_bpath;
|
|
|
|
g_return_if_fail (bpd != NULL);
|
|
g_return_if_fail (bpd->moveto_idx >= 0);
|
|
g_return_if_fail (bpd->n_bpath > 0);
|
|
|
|
bpath = bpd->bpath;
|
|
n_bpath = bpd->n_bpath;
|
|
|
|
/* Add closing vector if we need it. */
|
|
if (bpath[n_bpath - 1].x3 != bpath[bpd->moveto_idx].x3 ||
|
|
bpath[n_bpath - 1].y3 != bpath[bpd->moveto_idx].y3)
|
|
{
|
|
rsvg_bpath_def_lineto (bpd, bpath[bpd->moveto_idx].x3,
|
|
bpath[bpd->moveto_idx].y3);
|
|
bpath = bpd->bpath;
|
|
}
|
|
bpath[bpd->moveto_idx].code = ART_MOVETO;
|
|
bpd->moveto_idx = -1;
|
|
}
|
|
|
|
void
|
|
rsvg_bpath_def_art_finish (RsvgBpathDef *bpd)
|
|
{
|
|
int n_bpath;
|
|
|
|
g_return_if_fail (bpd != NULL);
|
|
|
|
n_bpath = bpd->n_bpath++;
|
|
|
|
if (n_bpath == bpd->n_bpath_max)
|
|
bpd->bpath = g_realloc (bpd->bpath,
|
|
(bpd->n_bpath_max <<= 1) * sizeof (ArtBpath));
|
|
bpd->bpath[n_bpath].code = ART_END;
|
|
}
|