mirror of
https://gitlab.gnome.org/GNOME/nautilus
synced 2024-11-05 16:04:31 +00:00
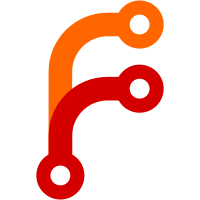
Fixes bugs #314, #324, and #325. * components/help/hyperbola.goad: Corrected repo_ids -> repo_id, reported by Richard Hult. * src/nautilus.goad: Added missing = character, reported by Richard Hult. * libnautilus/gnome-icon-container-grid.c: * libnautilus/gnome-icon-container-grid.h: * libnautilus/gnome-icon-container.c: * libnautilus/gnome-icon-container-private.h: Made the icon grid handle negative coordinates. Removed most of the icon grid code. Reimplemented the arrow keys. They should work again. * libnautilus/nautilus-gnome-extensions.c: * libnautilus/nautilus-gnome-extensions.h: (nautilus_art_irect_contains_irect): Added another helper function. * libnautilus/nautilus-icons-view-icon-item.c: * libnautilus/nautilus-icons-view-icon-item.h: * libnautilus/gnome-icon-container.c: * libnautilus/gnome-icon-container-private.h: Added user data to each canvas item and got rid of the hash table that maps canvas items to icons. * RENAMING: Tweaks.
180 lines
4.9 KiB
C
180 lines
4.9 KiB
C
/* -*- Mode: C; indent-tabs-mode: t; c-basic-offset: 8; tab-width: 8 -*- */
|
|
|
|
/* nautilus-gnome-extensions.c - implementation of new functions that operate on
|
|
gnome classes. Perhaps some of these should be
|
|
rolled into gnome someday.
|
|
|
|
Copyright (C) 1999, 2000 Eazel, Inc.
|
|
|
|
The Gnome Library is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU Library General Public License as
|
|
published by the Free Software Foundation; either version 2 of the
|
|
License, or (at your option) any later version.
|
|
|
|
The Gnome Library is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Library General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Library General Public
|
|
License along with the Gnome Library; see the file COPYING.LIB. If not,
|
|
write to the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
|
|
Authors: Darin Adler <darin@eazel.com>
|
|
*/
|
|
|
|
#include <config.h>
|
|
#include "nautilus-gnome-extensions.h"
|
|
|
|
void
|
|
nautilus_gnome_canvas_world_to_window_rectangle (GnomeCanvas *canvas,
|
|
const ArtDRect *world_rect,
|
|
ArtIRect *window_rect)
|
|
{
|
|
double x0, y0, x1, y1;
|
|
|
|
g_return_if_fail (GNOME_IS_CANVAS (canvas));
|
|
g_return_if_fail (world_rect != NULL);
|
|
g_return_if_fail (window_rect != NULL);
|
|
|
|
gnome_canvas_world_to_window (canvas,
|
|
world_rect->x0,
|
|
world_rect->y0,
|
|
&x0, &y0);
|
|
gnome_canvas_world_to_window (canvas,
|
|
world_rect->x1,
|
|
world_rect->y1,
|
|
&x1, &y1);
|
|
|
|
window_rect->x0 = x0;
|
|
window_rect->y0 = y0;
|
|
window_rect->x1 = x1;
|
|
window_rect->y1 = y1;
|
|
}
|
|
|
|
void
|
|
nautilus_gnome_canvas_world_to_canvas_rectangle (GnomeCanvas *canvas,
|
|
const ArtDRect *world_rect,
|
|
ArtIRect *canvas_rect)
|
|
{
|
|
g_return_if_fail (GNOME_IS_CANVAS (canvas));
|
|
g_return_if_fail (world_rect != NULL);
|
|
g_return_if_fail (canvas_rect != NULL);
|
|
|
|
gnome_canvas_w2c (canvas,
|
|
world_rect->x0,
|
|
world_rect->y0,
|
|
&canvas_rect->x0,
|
|
&canvas_rect->y0);
|
|
gnome_canvas_w2c (canvas,
|
|
world_rect->x1,
|
|
world_rect->y1,
|
|
&canvas_rect->x1,
|
|
&canvas_rect->y1);
|
|
}
|
|
|
|
gboolean
|
|
nautilus_art_irect_contains_irect (const ArtIRect *outer_rect,
|
|
const ArtIRect *inner_rect)
|
|
{
|
|
g_return_val_if_fail (outer_rect != NULL, FALSE);
|
|
g_return_val_if_fail (inner_rect != NULL, FALSE);
|
|
|
|
return outer_rect->x0 <= inner_rect->x0
|
|
&& outer_rect->y0 <= inner_rect->y0
|
|
&& outer_rect->x1 >= inner_rect->x1
|
|
&& outer_rect->y1 >= inner_rect->y1;
|
|
}
|
|
|
|
gboolean
|
|
nautilus_art_irect_hits_irect (const ArtIRect *rect_a,
|
|
const ArtIRect *rect_b)
|
|
{
|
|
ArtIRect intersection;
|
|
|
|
g_return_val_if_fail (rect_a != NULL, FALSE);
|
|
g_return_val_if_fail (rect_b != NULL, FALSE);
|
|
|
|
art_irect_intersect (&intersection, rect_a, rect_b);
|
|
return !art_irect_empty (&intersection);
|
|
}
|
|
|
|
gboolean
|
|
nautilus_art_irect_equal (const ArtIRect *rect_a,
|
|
const ArtIRect *rect_b)
|
|
{
|
|
g_return_val_if_fail (rect_a != NULL, FALSE);
|
|
g_return_val_if_fail (rect_b != NULL, FALSE);
|
|
|
|
return rect_a->x0 == rect_b->x0
|
|
&& rect_a->y0 == rect_b->y0
|
|
&& rect_a->x1 == rect_b->x1
|
|
&& rect_a->y1 == rect_b->y1;
|
|
}
|
|
|
|
gboolean
|
|
nautilus_art_drect_equal (const ArtDRect *rect_a,
|
|
const ArtDRect *rect_b)
|
|
{
|
|
g_return_val_if_fail (rect_a != NULL, FALSE);
|
|
g_return_val_if_fail (rect_b != NULL, FALSE);
|
|
|
|
return rect_a->x0 == rect_b->x0
|
|
&& rect_a->y0 == rect_b->y0
|
|
&& rect_a->x1 == rect_b->x1
|
|
&& rect_a->y1 == rect_b->y1;
|
|
}
|
|
|
|
void
|
|
nautilus_gnome_canvas_item_get_current_canvas_bounds (GnomeCanvasItem *item,
|
|
ArtIRect *bounds)
|
|
{
|
|
g_return_if_fail (GNOME_IS_CANVAS_ITEM (item));
|
|
g_return_if_fail (bounds != NULL);
|
|
|
|
bounds->x0 = item->x1;
|
|
bounds->y0 = item->y1;
|
|
bounds->x1 = item->x2;
|
|
bounds->y1 = item->y2;
|
|
}
|
|
|
|
void
|
|
nautilus_gnome_canvas_item_request_redraw (GnomeCanvasItem *item)
|
|
{
|
|
g_return_if_fail (GNOME_IS_CANVAS_ITEM (item));
|
|
|
|
gnome_canvas_request_redraw (item->canvas,
|
|
item->x1, item->y1,
|
|
item->x2, item->y2);
|
|
}
|
|
|
|
void
|
|
nautilus_gnome_canvas_request_redraw_rectangle (GnomeCanvas *canvas,
|
|
const ArtIRect *canvas_rectangle)
|
|
{
|
|
g_return_if_fail (GNOME_IS_CANVAS (canvas));
|
|
|
|
gnome_canvas_request_redraw (canvas,
|
|
canvas_rectangle->x0, canvas_rectangle->y0,
|
|
canvas_rectangle->x1, canvas_rectangle->y1);
|
|
}
|
|
|
|
void
|
|
nautilus_gnome_canvas_item_get_world_bounds (GnomeCanvasItem *item,
|
|
ArtDRect *world_bounds)
|
|
{
|
|
gnome_canvas_item_get_bounds (item,
|
|
&world_bounds->x0,
|
|
&world_bounds->y0,
|
|
&world_bounds->x1,
|
|
&world_bounds->y1);
|
|
if (item->parent != NULL) {
|
|
gnome_canvas_item_i2w (item->parent,
|
|
&world_bounds->x0,
|
|
&world_bounds->y0);
|
|
gnome_canvas_item_i2w (item->parent,
|
|
&world_bounds->x1,
|
|
&world_bounds->y1);
|
|
}
|
|
}
|