mirror of
https://gitlab.gnome.org/GNOME/nautilus
synced 2024-11-05 16:04:31 +00:00
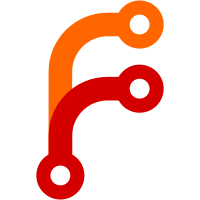
check will report if it fails instead of just aborting. Added new functions to manage a string that specifies a color or gradient, and added tests for the new functions.
508 lines
19 KiB
C
508 lines
19 KiB
C
/* -*- Mode: C; indent-tabs-mode: t; c-basic-offset: 8; tab-width: 8 -*-
|
|
|
|
gdk-extensions.c: Graphics routines to augment what's in gdk.
|
|
|
|
Copyright (C) 1999, 2000 Eazel, Inc.
|
|
|
|
The Gnome Library is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU Library General Public License as
|
|
published by the Free Software Foundation; either version 2 of the
|
|
License, or (at your option) any later version.
|
|
|
|
The Gnome Library is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Library General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Library General Public
|
|
License along with the Gnome Library; see the file COPYING.LIB. If not,
|
|
write to the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
|
|
Authors: Darin Adler <darin@eazel.com>
|
|
*/
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
#include <config.h>
|
|
#endif
|
|
|
|
#include "gdk-extensions.h"
|
|
|
|
#include "nautilus-lib-self-check-functions.h"
|
|
|
|
#include <string.h>
|
|
|
|
#define GRADIENT_BAND_SIZE 4
|
|
|
|
/**
|
|
* nautilus_fill_rectangle:
|
|
* @drawable: Target to draw into.
|
|
* @gc: Graphics context (mainly for clip).
|
|
* @rectangle: Rectangle to fill.
|
|
*
|
|
* Fill the rectangle with the foreground color.
|
|
* Convenient when you have a GdkRectangle structure.
|
|
*/
|
|
void
|
|
nautilus_fill_rectangle (GdkDrawable *drawable,
|
|
GdkGC *gc,
|
|
const GdkRectangle *rectangle)
|
|
{
|
|
gdk_draw_rectangle (drawable, gc, TRUE,
|
|
rectangle->x, rectangle->y, rectangle->width, rectangle->height);
|
|
}
|
|
|
|
/**
|
|
* nautilus_fill_rectangle_with_color:
|
|
* @drawable: Target to draw into.
|
|
* @gc: Graphics context (mainly for clip).
|
|
* @rectangle: Rectangle to fill.
|
|
* @color: Color to fill with.
|
|
*
|
|
* Fill the rectangle with a color.
|
|
* Convenient when you have a GdkRectangle structure.
|
|
*/
|
|
void
|
|
nautilus_fill_rectangle_with_color (GdkDrawable *drawable,
|
|
GdkGC *gc,
|
|
const GdkRectangle *rectangle,
|
|
const GdkColor *color)
|
|
{
|
|
GdkGCValues saved_values;
|
|
|
|
gdk_gc_get_values(gc, &saved_values);
|
|
gdk_gc_set_foreground (gc, (GdkColor *) color);
|
|
nautilus_fill_rectangle (drawable, gc, rectangle);
|
|
gdk_gc_set_foreground (gc, &saved_values.foreground);
|
|
}
|
|
|
|
/**
|
|
* nautilus_fill_rectangle_with_gradient:
|
|
* @drawable: Target to draw into.
|
|
* @gc: Graphics context (mainly for clip).
|
|
* @colormap: Map to use to allocate colors for gradient.
|
|
* @rectangle: Rectangle to draw gradient in.
|
|
* @start_color: Color for the left or top; pixel value does not matter.
|
|
* @end_color: Color for the right or bottom; pixel value does not matter.
|
|
* @horizontal: TRUE if the color changes from left to right. FALSE if from top to bottom.
|
|
*
|
|
* Fill the rectangle with a gradient.
|
|
* The color changes from start_color to end_color.
|
|
* A colormap is necessary because a gradient uses many different colors.
|
|
* This effect works best on true color displays.
|
|
*/
|
|
void
|
|
nautilus_fill_rectangle_with_gradient (GdkDrawable *drawable,
|
|
GdkGC *gc,
|
|
GdkColormap *colormap,
|
|
const GdkRectangle *rectangle,
|
|
const GdkColor *start_color,
|
|
const GdkColor *end_color,
|
|
gboolean horizontal)
|
|
{
|
|
GdkRectangle band_box;
|
|
gint16 *position;
|
|
guint16 *size;
|
|
gint num_bands;
|
|
guint16 last_band_size;
|
|
gdouble multiplier;
|
|
gint band;
|
|
|
|
g_return_if_fail (drawable);
|
|
g_return_if_fail (gc);
|
|
g_return_if_fail (rectangle);
|
|
g_return_if_fail (start_color);
|
|
g_return_if_fail (end_color);
|
|
g_return_if_fail (horizontal == FALSE || horizontal == TRUE);
|
|
|
|
/* Set up the band box so we can access it the same way for horizontal or vertical. */
|
|
band_box = *rectangle;
|
|
position = horizontal ? &band_box.x : &band_box.y;
|
|
size = horizontal ? &band_box.width : &band_box.height;
|
|
|
|
/* Figure out how many bands we will need. */
|
|
num_bands = (*size + GRADIENT_BAND_SIZE - 1) / GRADIENT_BAND_SIZE;
|
|
last_band_size = GRADIENT_BAND_SIZE - (GRADIENT_BAND_SIZE * num_bands - *size);
|
|
|
|
/* Change the band box to be the size of a single band. */
|
|
*size = GRADIENT_BAND_SIZE;
|
|
|
|
/* Set up a multiplier to use to interpolate the colors as we go. */
|
|
multiplier = num_bands <= 1 ? 0.0 : 1.0 / (num_bands - 1);
|
|
|
|
/* Fill each band with a separate nautilus_draw_rectangle call. */
|
|
for (band = 0; band < num_bands; band++) {
|
|
GdkColor band_color;
|
|
|
|
/* Compute a new color value for each band. */
|
|
nautilus_interpolate_color (band * multiplier, start_color, end_color, &band_color);
|
|
if (!gdk_colormap_alloc_color (colormap, &band_color, FALSE, TRUE))
|
|
g_warning ("could not allocate color for gradient");
|
|
else {
|
|
/* Last band may need to be a bit smaller to avoid writing outside the box.
|
|
* This is more efficient than changing and restoring the clip.
|
|
*/
|
|
if (band == num_bands - 1)
|
|
*size = last_band_size;
|
|
|
|
nautilus_fill_rectangle_with_color (drawable, gc, &band_box, &band_color);
|
|
}
|
|
*position += *size;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* nautilus_interpolate_color:
|
|
* @ratio: Place on line between colors to interpolate.
|
|
* @start_color: Color for one end.
|
|
* @end_color: Color for the other end
|
|
* @interpolated_color: Result.
|
|
*
|
|
* Compute a color between @start_color and @end_color in color space.
|
|
* Currently, the color space used is RGB, but a future version could
|
|
* instead do the interpolation in the best color space for expressing
|
|
* human perception.
|
|
*/
|
|
void
|
|
nautilus_interpolate_color (gdouble ratio,
|
|
const GdkColor *start_color,
|
|
const GdkColor *end_color,
|
|
GdkColor *interpolated_color)
|
|
{
|
|
g_return_if_fail (ratio >= 0.0);
|
|
g_return_if_fail (ratio <= 1.0);
|
|
g_return_if_fail (start_color);
|
|
g_return_if_fail (end_color);
|
|
g_return_if_fail (interpolated_color);
|
|
|
|
interpolated_color->red = start_color->red * (1.0 - ratio) + end_color->red * ratio;
|
|
interpolated_color->green = start_color->green * (1.0 - ratio) + end_color->green * ratio;
|
|
interpolated_color->blue = start_color->blue * (1.0 - ratio) + end_color->blue * ratio;
|
|
}
|
|
|
|
/**
|
|
* nautilus_gradient_new
|
|
* @start_color: Color for the top or left.
|
|
* @end_color: Color for the bottom or right.
|
|
* @is_horizontal: Direction of the gradient.
|
|
*
|
|
* Create a string that combines the start and end colors along
|
|
* with the direction of the gradient in a standard format.
|
|
*/
|
|
char *
|
|
nautilus_gradient_new (const char *start_color,
|
|
const char *end_color,
|
|
gboolean is_horizontal)
|
|
{
|
|
g_return_val_if_fail (start_color != NULL, g_strdup (""));
|
|
g_return_val_if_fail (end_color != NULL, g_strdup (""));
|
|
g_return_val_if_fail (is_horizontal == FALSE || is_horizontal == TRUE, g_strdup (""));
|
|
|
|
/* Handle the special case where the start and end colors are identical.
|
|
Handle the special case where the end color is an empty string.
|
|
*/
|
|
if (strcmp(start_color, end_color) == 0 || end_color[0] == '\0')
|
|
return g_strdup (start_color);
|
|
|
|
/* Handle the special case where the start color is an empty string. */
|
|
if (start_color[0] == '\0')
|
|
return g_strdup (end_color);
|
|
|
|
/* Handle the general case. */
|
|
return g_strconcat (start_color, "-", end_color, is_horizontal ? ":h" : NULL, NULL);
|
|
}
|
|
|
|
/**
|
|
* nautilus_gradient_is_gradient
|
|
* @gradient_spec: A gradient spec. string.
|
|
*
|
|
* Return true if the spec. specifies a gradient instead of a solid color.
|
|
*/
|
|
gboolean
|
|
nautilus_gradient_is_gradient (const char *gradient_spec)
|
|
{
|
|
g_return_val_if_fail (gradient_spec != NULL, FALSE);
|
|
|
|
return strchr (gradient_spec, '-') != NULL;
|
|
}
|
|
|
|
static char *
|
|
nautilus_gradient_strip_trailing_direction_if_any (const char *gradient_spec)
|
|
{
|
|
size_t length;
|
|
|
|
g_return_val_if_fail (gradient_spec != NULL, g_strdup (""));
|
|
|
|
length = strlen (gradient_spec);
|
|
if (length >= 2 && gradient_spec[length - 2] == ':'
|
|
&& (gradient_spec[length - 1] == 'v' || gradient_spec[length - 1] == 'h'))
|
|
length -= 2;
|
|
|
|
return g_strndup (gradient_spec, length);
|
|
}
|
|
|
|
/**
|
|
* nautilus_gradient_get_start_color_spec
|
|
* @gradient_spec: A gradient spec. string.
|
|
*
|
|
* Return the start color.
|
|
* This may be the entire gradient_spec if it's a solid color.
|
|
*/
|
|
char *
|
|
nautilus_gradient_get_start_color_spec (const char *gradient_spec)
|
|
{
|
|
const char *separator;
|
|
|
|
g_return_val_if_fail (gradient_spec != NULL, g_strdup (""));
|
|
|
|
separator = strchr (gradient_spec, '-');
|
|
if (separator == NULL)
|
|
return nautilus_gradient_strip_trailing_direction_if_any (gradient_spec);
|
|
|
|
return g_strndup (gradient_spec, separator - gradient_spec);
|
|
}
|
|
|
|
/**
|
|
* nautilus_gradient_get_end_color_spec
|
|
* @gradient_spec: A gradient spec. string.
|
|
*
|
|
* Return the end color.
|
|
* This may be the entire gradient_spec if it's a solid color.
|
|
*/
|
|
char *
|
|
nautilus_gradient_get_end_color_spec (const char *gradient_spec)
|
|
{
|
|
const char *separator;
|
|
|
|
g_return_val_if_fail (gradient_spec != NULL, g_strdup (""));
|
|
|
|
separator = strchr (gradient_spec, '-');
|
|
return nautilus_gradient_strip_trailing_direction_if_any
|
|
(separator != NULL ? separator + 1 : gradient_spec);
|
|
}
|
|
|
|
/* Do the work shared by all the set_color_spec functions below. */
|
|
static char *
|
|
nautilus_gradient_set_edge_color (const char *gradient_spec,
|
|
const char *edge_color,
|
|
gboolean is_horizontal,
|
|
gboolean change_end)
|
|
{
|
|
char *opposite_color;
|
|
char *result;
|
|
|
|
g_return_val_if_fail (gradient_spec != NULL, g_strdup (""));
|
|
g_return_val_if_fail (edge_color != NULL, g_strdup (gradient_spec));
|
|
|
|
/* Get the color from the existing gradient spec. for the opposite
|
|
edge. This will parse away all the stuff we don't want from the
|
|
old gradient spec.
|
|
*/
|
|
opposite_color = change_end
|
|
? nautilus_gradient_get_start_color_spec (gradient_spec)
|
|
: nautilus_gradient_get_end_color_spec (gradient_spec);
|
|
|
|
/* Create a new gradient spec. The nautilus_gradient_new function handles
|
|
some special cases, so we don't have to bother with them here.
|
|
*/
|
|
result = nautilus_gradient_new (change_end ? opposite_color : edge_color,
|
|
change_end ? edge_color : opposite_color,
|
|
is_horizontal);
|
|
|
|
g_free (opposite_color);
|
|
|
|
return result;
|
|
}
|
|
|
|
/**
|
|
* nautilus_gradient_set_left_color_spec
|
|
* @gradient_spec: A gradient spec. string.
|
|
* @left_color: Color spec. to replace left color with.
|
|
*
|
|
* Changes the left color to what's passed in.
|
|
* This creates a horizontal gradient.
|
|
*/
|
|
char *
|
|
nautilus_gradient_set_left_color_spec (const char *gradient_spec,
|
|
const char *left_color)
|
|
{
|
|
return nautilus_gradient_set_edge_color (gradient_spec, left_color, TRUE, FALSE);
|
|
}
|
|
|
|
/**
|
|
* nautilus_gradient_set_top_color_spec
|
|
* @gradient_spec: A gradient spec. string.
|
|
* @top_color: Color spec. to replace top color with.
|
|
*
|
|
* Changes the top color to what's passed in.
|
|
* This creates a vertical gradient.
|
|
*/
|
|
char *
|
|
nautilus_gradient_set_top_color_spec (const char *gradient_spec,
|
|
const char *top_color)
|
|
{
|
|
return nautilus_gradient_set_edge_color (gradient_spec, top_color, FALSE, FALSE);
|
|
}
|
|
|
|
/**
|
|
* nautilus_gradient_set_right_color_spec
|
|
* @gradient_spec: A gradient spec. string.
|
|
* @right_color: Color spec. to replace right color with.
|
|
*
|
|
* Changes the right color to what's passed in.
|
|
* This creates a horizontal gradient.
|
|
*/
|
|
char *
|
|
nautilus_gradient_set_right_color_spec (const char *gradient_spec,
|
|
const char *right_color)
|
|
{
|
|
return nautilus_gradient_set_edge_color (gradient_spec, right_color, TRUE, TRUE);
|
|
}
|
|
|
|
/**
|
|
* nautilus_gradient_set_bottom_color_spec
|
|
* @gradient_spec: A gradient spec. string.
|
|
* @bottom_color: Color spec. to replace bottom color with.
|
|
*
|
|
* Changes the bottom color to what's passed in.
|
|
* This creates a vertical gradient.
|
|
*/
|
|
char *
|
|
nautilus_gradient_set_bottom_color_spec (const char *gradient_spec,
|
|
const char *bottom_color)
|
|
{
|
|
return nautilus_gradient_set_edge_color (gradient_spec, bottom_color, FALSE, TRUE);
|
|
}
|
|
|
|
#if ! defined (NAUTILUS_OMIT_SELF_CHECK)
|
|
|
|
#include <stdio.h>
|
|
|
|
static GdkColor
|
|
nautilus_self_check_interpolate (gdouble ratio,
|
|
gushort r1, gushort g1, gushort b1,
|
|
gushort r2, gushort g2, gushort b2)
|
|
{
|
|
GdkColor start_color;
|
|
GdkColor end_color;
|
|
GdkColor interpolated_color;
|
|
|
|
start_color.red = r1;
|
|
start_color.green = g1;
|
|
start_color.blue = b1;
|
|
|
|
end_color.red = r2;
|
|
end_color.green = g2;
|
|
end_color.blue = b2;
|
|
|
|
nautilus_interpolate_color (ratio, &start_color, &end_color, &interpolated_color);
|
|
|
|
return interpolated_color;
|
|
}
|
|
|
|
void
|
|
nautilus_self_check_gdk_extensions (void)
|
|
{
|
|
/* nautilus_interpolate_color */
|
|
NAUTILUS_CHECK_INTEGER_RESULT (nautilus_self_check_interpolate (0.0, 0, 0, 0, 0, 0, 0).red, 0);
|
|
NAUTILUS_CHECK_INTEGER_RESULT (nautilus_self_check_interpolate (0.0, 0, 0, 0, 0, 0, 0).green, 0);
|
|
NAUTILUS_CHECK_INTEGER_RESULT (nautilus_self_check_interpolate (0.0, 0, 0, 0, 0, 0, 0).blue, 0);
|
|
NAUTILUS_CHECK_INTEGER_RESULT (nautilus_self_check_interpolate (0.0, 0, 0, 0, 0xFFFF, 0xFFFF, 0xFFFF).red, 0);
|
|
NAUTILUS_CHECK_INTEGER_RESULT (nautilus_self_check_interpolate (0.0, 0, 0, 0, 0xFFFF, 0xFFFF, 0xFFFF).green, 0);
|
|
NAUTILUS_CHECK_INTEGER_RESULT (nautilus_self_check_interpolate (0.0, 0, 0, 0, 0xFFFF, 0xFFFF, 0xFFFF).blue, 0);
|
|
NAUTILUS_CHECK_INTEGER_RESULT (nautilus_self_check_interpolate (0.5, 0, 0, 0, 0xFFFF, 0xFFFF, 0xFFFF).red, 0x7FFF);
|
|
NAUTILUS_CHECK_INTEGER_RESULT (nautilus_self_check_interpolate (0.5, 0, 0, 0, 0xFFFF, 0xFFFF, 0xFFFF).green, 0x7FFF);
|
|
NAUTILUS_CHECK_INTEGER_RESULT (nautilus_self_check_interpolate (0.5, 0, 0, 0, 0xFFFF, 0xFFFF, 0xFFFF).blue, 0x7FFF);
|
|
NAUTILUS_CHECK_INTEGER_RESULT (nautilus_self_check_interpolate (1.0, 0, 0, 0, 0xFFFF, 0xFFFF, 0xFFFF).red, 0xFFFF);
|
|
NAUTILUS_CHECK_INTEGER_RESULT (nautilus_self_check_interpolate (1.0, 0, 0, 0, 0xFFFF, 0xFFFF, 0xFFFF).green, 0xFFFF);
|
|
NAUTILUS_CHECK_INTEGER_RESULT (nautilus_self_check_interpolate (1.0, 0, 0, 0, 0xFFFF, 0xFFFF, 0xFFFF).blue, 0xFFFF);
|
|
|
|
/* nautilus_fill_rectangle */
|
|
/* Make a GdkImage and fill it, maybe? */
|
|
|
|
/* nautilus_fill_rectangle_with_color */
|
|
|
|
/* nautilus_fill_rectangle_with_gradient */
|
|
|
|
/* nautilus_gradient_new */
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_new ("", "", FALSE), "");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_new ("a", "b", FALSE), "a-b");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_new ("a", "b", TRUE), "a-b:h");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_new ("a", "a", FALSE), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_new ("a", "a", TRUE), "a");
|
|
|
|
/* nautilus_gradient_is_gradient */
|
|
NAUTILUS_CHECK_BOOLEAN_RESULT (nautilus_gradient_is_gradient (""), FALSE);
|
|
NAUTILUS_CHECK_BOOLEAN_RESULT (nautilus_gradient_is_gradient ("-"), TRUE);
|
|
NAUTILUS_CHECK_BOOLEAN_RESULT (nautilus_gradient_is_gradient ("a"), FALSE);
|
|
NAUTILUS_CHECK_BOOLEAN_RESULT (nautilus_gradient_is_gradient ("a-b"), TRUE);
|
|
NAUTILUS_CHECK_BOOLEAN_RESULT (nautilus_gradient_is_gradient ("a-b:h"), TRUE);
|
|
|
|
/* nautilus_gradient_get_start_color_spec */
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_start_color_spec (""), "");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_start_color_spec ("-"), "");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_start_color_spec ("a"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_start_color_spec ("a-b"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_start_color_spec ("a-"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_start_color_spec ("-b"), "");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_start_color_spec ("a:h"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_start_color_spec ("a:v"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_start_color_spec ("a:c"), "a:c");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_start_color_spec ("a:-b"), "a:");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_start_color_spec ("a:-b:v"), "a:");
|
|
|
|
/* nautilus_gradient_get_end_color_spec */
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_end_color_spec (""), "");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_end_color_spec ("-"), "");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_end_color_spec ("a"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_end_color_spec ("a-b"), "b");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_end_color_spec ("a-"), "");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_end_color_spec ("-b"), "b");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_end_color_spec ("a:h"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_end_color_spec ("a:v"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_end_color_spec ("a:c"), "a:c");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_end_color_spec ("a:-b"), "b");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_get_end_color_spec ("a:-b:v"), "b");
|
|
|
|
/* nautilus_gradient_set_left_color_spec */
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_left_color_spec ("", ""), "");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_left_color_spec ("", "a"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_left_color_spec ("a", ""), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_left_color_spec ("a", "a"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_left_color_spec ("a", "b"), "b-a:h");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_left_color_spec ("a-c:v", "b"), "b-c:h");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_left_color_spec ("a-c:v", "c"), "c");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_left_color_spec ("a:-b:v", "d"), "d-b:h");
|
|
|
|
/* nautilus_gradient_set_top_color_spec */
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_top_color_spec ("", ""), "");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_top_color_spec ("", "a"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_top_color_spec ("a", ""), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_top_color_spec ("a", "a"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_top_color_spec ("a", "b"), "b-a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_top_color_spec ("a-c:v", "b"), "b-c");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_top_color_spec ("a-c:v", "c"), "c");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_top_color_spec ("a:-b:h", "d"), "d-b");
|
|
|
|
/* nautilus_gradient_set_right_color_spec */
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_right_color_spec ("", ""), "");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_right_color_spec ("", "a"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_right_color_spec ("a", ""), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_right_color_spec ("a", "a"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_right_color_spec ("a", "b"), "a-b:h");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_right_color_spec ("a-c:v", "b"), "a-b:h");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_right_color_spec ("a-c:v", "c"), "a-c:h");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_right_color_spec ("a:-b:v", "d"), "a:-d:h");
|
|
|
|
/* nautilus_gradient_set_bottom_color_spec */
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_bottom_color_spec ("", ""), "");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_bottom_color_spec ("", "a"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_bottom_color_spec ("a", ""), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_bottom_color_spec ("a", "a"), "a");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_bottom_color_spec ("a", "b"), "a-b");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_bottom_color_spec ("a-c:v", "b"), "a-b");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_bottom_color_spec ("a-c:v", "c"), "a-c");
|
|
NAUTILUS_CHECK_STRING_RESULT (nautilus_gradient_set_bottom_color_spec ("a:-b:h", "d"), "a:-d");
|
|
}
|
|
|
|
#endif /* ! NAUTILUS_OMIT_SELF_CHECK */
|