mirror of
https://gitlab.gnome.org/GNOME/nautilus
synced 2024-11-05 16:04:31 +00:00
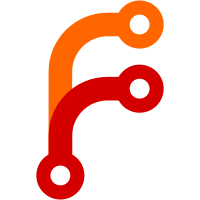
creating a scalable icon. Instead it should be possible to get a scalable icon and then get either an anti-aliased-optimized or non-anti-alias-optimized version of the icon. * libnautilus-extensions/nautilus-bookmark.h: * libnautilus-extensions/nautilus-bookmark.c: (nautilus_bookmark_get_pixmap_and_mask): Pass FALSE so we get the non-anti-aliased version. (nautilus_bookmark_get_pixbuf): Add parameter to indicate if we want the anti-aliased-optimized version or not. (nautilus_bookmark_update_icon): Get rid of anti-aliased boolean here since we are just updating the scalable icon. (nautilus_bookmark_set_icon_to_default): Get rid of anti-aliased boolean here since we are just updating the scalable icon. * libnautilus-extensions/nautilus-icon-container.c: (nautilus_icon_container_update_icon): Pass in a flag to indicate if we need the anti-aliased version of the icon or not. * libnautilus-extensions/nautilus-icon-factory.h: * libnautilus-extensions/nautilus-icon-factory.c: (nautilus_scalable_icon_new_from_text_pieces): Removed the anti-aliased parameter. (nautilus_scalable_icon_hash), (nautilus_scalable_icon_equal): Remove the anti-aliased flag. A scalable icon itself is created before we make the "anti-aliased or not" choice. (nautilus_icon_factory_get_icon_for_file): Since we no longer know whether the icon will be needed anti-aliased or not, make the thumbnail code always use the anti-aliased version (and added FIXME). Also changed the "loading" image to use the normal icon name case instead of hard-coding. (nautilus_icon_factory_get_emblem_icon_by_name): Removed the anti-aliased parameter. (nautilus_icon_factory_get_emblem_icons_for_file): Removed the anti-aliased parameter. (load_specific_icon): Add an anti-aliased parameter. (load_icon_for_scaling): Add an anti-aliased parameter. (load_icon_scale_if_necessary): Pass in the anti-aliased parameter from the icon size request. (get_icon_from_cache): Pass in the anti-aliased parameter from the icon size request. (nautilus_icon_factory_get_pixbuf_for_icon): Add an anti-aliased parameter. (cache_key_hash): Include the anti-aliased flag in the hash. (cache_key_equal): Include the anti-aliased flag in the compare. (nautilus_icon_factory_get_pixbuf_for_file): Pass the anti-aliased parameter into the get_pixbuf half, not the get_icon_for_file half. (nautilus_icon_factory_get_pixbuf_from_name): Pass the anti-aliased parameter into the get_pixbuf half, not the scalable_icon_new half. (load_icon_with_embedded_text): Remove the anti-aliased parameter. * src/file-manager/fm-icon-view.c: (get_icon_images_callback): Get rid of code to pass in the anti-aliased parameter. * src/file-manager/fm-list-view.c: (fm_list_view_get_emblem_pixbufs_for_file): Pass the anti-aliased parameter to the pixbuf-getting function, not the scalable-icon-getting part. * src/nautilus-bookmark-parsing.c: (nautilus_bookmark_new_from_node): No need to pass an anti-aliased parameter (before it was always FALSE). * src/nautilus-sidebar-title.c: (update_emblems): Pass FALSE for the anti-aliased parameter to the get_pixbuf call rather than to the get_icons call. * src/nautilus-window-manage-views.c: (get_history_list_callback): No need to pass FALSE for anti-aliased any more. * src/nautilus-window-menus.c: (append_bookmark_to_menu): No need to pass FALSE for anti-aliased any more.
101 lines
4 KiB
C
101 lines
4 KiB
C
/* -*- Mode: C; indent-tabs-mode: t; c-basic-offset: 8; tab-width: 8 -*- */
|
|
|
|
/* nautilus-bookmark.h - interface for individual bookmarks.
|
|
|
|
Copyright (C) 1999, 2000 Eazel, Inc.
|
|
|
|
The Gnome Library is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU Library General Public License as
|
|
published by the Free Software Foundation; either version 2 of the
|
|
License, or (at your option) any later version.
|
|
|
|
The Gnome Library is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Library General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Library General Public
|
|
License along with the Gnome Library; see the file COPYING.LIB. If not,
|
|
write to the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
|
|
Authors: John Sullivan <sullivan@eazel.com>
|
|
*/
|
|
|
|
#ifndef NAUTILUS_BOOKMARK_H
|
|
#define NAUTILUS_BOOKMARK_H
|
|
|
|
#include "nautilus-icon-factory.h"
|
|
|
|
#include <gtk/gtkwidget.h>
|
|
#include <gdk/gdktypes.h>
|
|
#include <gdk-pixbuf/gdk-pixbuf.h>
|
|
|
|
typedef struct NautilusBookmark NautilusBookmark;
|
|
|
|
#define NAUTILUS_TYPE_BOOKMARK \
|
|
(nautilus_bookmark_get_type ())
|
|
#define NAUTILUS_BOOKMARK(obj) \
|
|
(GTK_CHECK_CAST ((obj), NAUTILUS_TYPE_BOOKMARK, NautilusBookmark))
|
|
#define NAUTILUS_BOOKMARK_CLASS(klass) \
|
|
(GTK_CHECK_CLASS_CAST ((klass), NAUTILUS_TYPE_BOOKMARK, NautilusBookmarkClass))
|
|
#define NAUTILUS_IS_BOOKMARK(obj) \
|
|
(GTK_CHECK_TYPE ((obj), NAUTILUS_TYPE_BOOKMARK))
|
|
#define NAUTILUS_IS_BOOKMARK_CLASS(klass) \
|
|
(GTK_CHECK_CLASS_TYPE ((klass), NAUTILUS_TYPE_BOOKMARK))
|
|
|
|
typedef struct NautilusBookmarkDetails NautilusBookmarkDetails;
|
|
|
|
struct NautilusBookmark {
|
|
GtkObject object;
|
|
NautilusBookmarkDetails *details;
|
|
};
|
|
|
|
struct NautilusBookmarkClass {
|
|
GtkObjectClass parent_class;
|
|
|
|
/* Signals that clients can connect to. */
|
|
|
|
/* The appearance_changed signal is emitted when the bookmark's
|
|
* name or icon has changed.
|
|
*/
|
|
void (* appearance_changed) (NautilusBookmark *bookmark);
|
|
|
|
/* The contents_changed signal is emitted when the bookmark's
|
|
* URI has changed.
|
|
*/
|
|
void (* contents_changed) (NautilusBookmark *bookmark);
|
|
};
|
|
|
|
typedef struct NautilusBookmarkClass NautilusBookmarkClass;
|
|
|
|
GtkType nautilus_bookmark_get_type (void);
|
|
NautilusBookmark * nautilus_bookmark_new (const char *uri,
|
|
const char *name);
|
|
NautilusBookmark * nautilus_bookmark_new_with_icon (const char *uri,
|
|
const char *name,
|
|
NautilusScalableIcon *icon);
|
|
NautilusBookmark * nautilus_bookmark_copy (NautilusBookmark *bookmark);
|
|
char * nautilus_bookmark_get_name (NautilusBookmark *bookmark);
|
|
char * nautilus_bookmark_get_uri (NautilusBookmark *bookmark);
|
|
NautilusScalableIcon *nautilus_bookmark_get_icon (NautilusBookmark *bookmark);
|
|
void nautilus_bookmark_set_name (NautilusBookmark *bookmark,
|
|
const char *new_name);
|
|
gboolean nautilus_bookmark_uri_known_not_to_exist (NautilusBookmark *bookmark);
|
|
int nautilus_bookmark_compare_with (gconstpointer a,
|
|
gconstpointer b);
|
|
int nautilus_bookmark_compare_uris (gconstpointer a,
|
|
gconstpointer b);
|
|
|
|
/* Helper functions for displaying bookmarks */
|
|
gboolean nautilus_bookmark_get_pixmap_and_mask (NautilusBookmark *bookmark,
|
|
guint icon_size,
|
|
GdkPixmap **pixmap_return,
|
|
GdkBitmap **mask_return);
|
|
GdkPixbuf * nautilus_bookmark_get_pixbuf (NautilusBookmark *bookmark,
|
|
guint icon_size,
|
|
gboolean optimize_for_anti_aliasing);
|
|
GtkWidget * nautilus_bookmark_menu_item_new (NautilusBookmark *bookmark);
|
|
|
|
|
|
#endif /* NAUTILUS_BOOKMARK_H */
|