mirror of
https://gitlab.gnome.org/GNOME/nautilus
synced 2024-08-24 18:25:50 +00:00
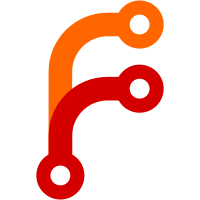
2008-07-13 Christian Neumair <cneumair@gnome.org> * libnautilus-private/nautilus-dnd.c (nautilus_drag_uri_array_from_selection_list), (nautilus_drag_uri_list_from_selection_list), (nautilus_drag_uri_array_from_list), (nautilus_drag_uri_list_from_array), (slot_proxy_drag_motion), (drag_info_clear), (slot_proxy_drag_leave), (slot_proxy_drag_drop), (slot_proxy_handle_drop), (slot_proxy_drag_data_received), (nautilus_drag_slot_proxy_init): * libnautilus-private/nautilus-dnd.h: Add simple slot drop proxy API to libnautilus-private, centered around nautilus_drag_slot_proxy_init(). The notebook tab label drop targets will later be ported to this API. Add API for converting URI lists to URI arrays, and for constructing URI lists and arrays from selection lists. * src/nautilus-notebook.c (notebook_tab_drag_data_received): Use nautilus_drag_uri_array_from_selection_list(). * src/nautilus-pathbar.c (slider_timeout), (nautilus_path_bar_slider_drag_motion), (nautilus_path_bar_slider_drag_leave), (nautilus_path_bar_init), (nautilus_path_bar_finalize), (button_data_free), (button_drag_data_get_cb), (setup_button_drag_source), (make_directory_button): * src/nautilus-pathbar.h: Add path bar drop targets, use nautilus_drag_slot_proxy_init() for setup. Also offer GNOME icon lists as drag target. Reveal hidden paths when hovering over the up/down sliders for some time. Fixes #309842. svn path=/trunk/; revision=14356
193 lines
7.5 KiB
C
193 lines
7.5 KiB
C
/* -*- Mode: C; indent-tabs-mode: t; c-basic-offset: 8; tab-width: 8 -*- */
|
|
|
|
/* nautilus-dnd.h - Common Drag & drop handling code shared by the icon container
|
|
and the list view.
|
|
|
|
Copyright (C) 2000 Eazel, Inc.
|
|
|
|
The Gnome Library is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU Library General Public License as
|
|
published by the Free Software Foundation; either version 2 of the
|
|
License, or (at your option) any later version.
|
|
|
|
The Gnome Library is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Library General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Library General Public
|
|
License along with the Gnome Library; see the file COPYING.LIB. If not,
|
|
write to the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
|
|
Authors: Pavel Cisler <pavel@eazel.com>,
|
|
Ettore Perazzoli <ettore@gnu.org>
|
|
*/
|
|
|
|
#ifndef NAUTILUS_DND_H
|
|
#define NAUTILUS_DND_H
|
|
|
|
#include <gtk/gtk.h>
|
|
#include <libnautilus-private/nautilus-window-slot-info.h>
|
|
|
|
/* Drag & Drop target names. */
|
|
#define NAUTILUS_ICON_DND_GNOME_ICON_LIST_TYPE "x-special/gnome-icon-list"
|
|
#define NAUTILUS_ICON_DND_URI_LIST_TYPE "text/uri-list"
|
|
#define NAUTILUS_ICON_DND_NETSCAPE_URL_TYPE "_NETSCAPE_URL"
|
|
#define NAUTILUS_ICON_DND_COLOR_TYPE "application/x-color"
|
|
#define NAUTILUS_ICON_DND_BGIMAGE_TYPE "property/bgimage"
|
|
#define NAUTILUS_ICON_DND_KEYWORD_TYPE "property/keyword"
|
|
#define NAUTILUS_ICON_DND_RESET_BACKGROUND_TYPE "x-special/gnome-reset-background"
|
|
#define NAUTILUS_ICON_DND_ROOTWINDOW_DROP_TYPE "application/x-rootwindow-drop"
|
|
#define NAUTILUS_ICON_DND_XDNDDIRECTSAVE_TYPE "XdndDirectSave0" /* XDS Protocol Type */
|
|
|
|
/* Item of the drag selection list */
|
|
typedef struct {
|
|
char *uri;
|
|
gboolean got_icon_position;
|
|
int icon_x, icon_y;
|
|
int icon_width, icon_height;
|
|
} NautilusDragSelectionItem;
|
|
|
|
/* Standard Drag & Drop types. */
|
|
typedef enum {
|
|
NAUTILUS_ICON_DND_GNOME_ICON_LIST,
|
|
NAUTILUS_ICON_DND_URI_LIST,
|
|
NAUTILUS_ICON_DND_NETSCAPE_URL,
|
|
NAUTILUS_ICON_DND_COLOR,
|
|
NAUTILUS_ICON_DND_BGIMAGE,
|
|
NAUTILUS_ICON_DND_KEYWORD,
|
|
NAUTILUS_ICON_DND_TEXT,
|
|
NAUTILUS_ICON_DND_RESET_BACKGROUND,
|
|
NAUTILUS_ICON_DND_XDNDDIRECTSAVE,
|
|
NAUTILUS_ICON_DND_ROOTWINDOW_DROP
|
|
} NautilusIconDndTargetType;
|
|
|
|
typedef enum {
|
|
NAUTILUS_DND_ACTION_FIRST = GDK_ACTION_ASK << 1,
|
|
NAUTILUS_DND_ACTION_SET_AS_BACKGROUND = NAUTILUS_DND_ACTION_FIRST << 0,
|
|
NAUTILUS_DND_ACTION_SET_AS_FOLDER_BACKGROUND = NAUTILUS_DND_ACTION_FIRST << 1,
|
|
NAUTILUS_DND_ACTION_SET_AS_GLOBAL_BACKGROUND = NAUTILUS_DND_ACTION_FIRST << 2
|
|
} NautilusDndAction;
|
|
|
|
/* drag&drop-related information. */
|
|
typedef struct {
|
|
GtkTargetList *target_list;
|
|
|
|
/* Stuff saved at "receive data" time needed later in the drag. */
|
|
gboolean got_drop_data_type;
|
|
NautilusIconDndTargetType data_type;
|
|
GtkSelectionData *selection_data;
|
|
char *direct_save_uri;
|
|
|
|
/* Start of the drag, in window coordinates. */
|
|
int start_x, start_y;
|
|
|
|
/* List of NautilusDragSelectionItems, representing items being dragged, or NULL
|
|
* if data about them has not been received from the source yet.
|
|
*/
|
|
GList *selection_list;
|
|
|
|
/* has the drop occured ? */
|
|
gboolean drop_occured;
|
|
|
|
/* whether or not need to clean up the previous dnd data */
|
|
gboolean need_to_destroy;
|
|
|
|
/* autoscrolling during dragging */
|
|
int auto_scroll_timeout_id;
|
|
gboolean waiting_to_autoscroll;
|
|
gint64 start_auto_scroll_in;
|
|
|
|
} NautilusDragInfo;
|
|
|
|
typedef struct {
|
|
/* NB: the following elements are managed by us */
|
|
gboolean have_data;
|
|
gboolean have_valid_data;
|
|
|
|
gboolean drop_occured;
|
|
|
|
unsigned int info;
|
|
union {
|
|
GList *selection_list;
|
|
GList *uri_list;
|
|
char *netscape_url;
|
|
} data;
|
|
|
|
/* NB: the following elements are managed by the caller of
|
|
* nautilus_drag_slot_proxy_init() */
|
|
|
|
/* a fixed location, or NULL to use slot's location */
|
|
GFile *target_location;
|
|
/* a fixed slot, or NULL to use the window's active slot */
|
|
NautilusWindowSlotInfo *target_slot;
|
|
} NautilusDragSlotProxyInfo;
|
|
|
|
typedef void (* NautilusDragEachSelectedItemDataGet) (const char *url,
|
|
int x, int y, int w, int h,
|
|
gpointer data);
|
|
typedef void (* NautilusDragEachSelectedItemIterator) (NautilusDragEachSelectedItemDataGet iteratee,
|
|
gpointer iterator_context,
|
|
gpointer data);
|
|
|
|
void nautilus_drag_init (NautilusDragInfo *drag_info,
|
|
const GtkTargetEntry *drag_types,
|
|
int drag_type_count,
|
|
gboolean add_text_targets);
|
|
void nautilus_drag_finalize (NautilusDragInfo *drag_info);
|
|
NautilusDragSelectionItem *nautilus_drag_selection_item_new (void);
|
|
void nautilus_drag_destroy_selection_list (GList *selection_list);
|
|
GList *nautilus_drag_build_selection_list (GtkSelectionData *data);
|
|
|
|
char ** nautilus_drag_uri_array_from_selection_list (const GList *selection_list);
|
|
GList * nautilus_drag_uri_list_from_selection_list (const GList *selection_list);
|
|
|
|
char ** nautilus_drag_uri_array_from_list (const GList *uri_list);
|
|
GList * nautilus_drag_uri_list_from_array (const char **uris);
|
|
|
|
gboolean nautilus_drag_items_local (const char *target_uri,
|
|
const GList *selection_list);
|
|
gboolean nautilus_drag_uris_local (const char *target_uri,
|
|
const GList *source_uri_list);
|
|
gboolean nautilus_drag_items_in_trash (const GList *selection_list);
|
|
gboolean nautilus_drag_items_on_desktop (const GList *selection_list);
|
|
void nautilus_drag_default_drop_action_for_icons (GdkDragContext *context,
|
|
const char *target_uri,
|
|
const GList *items,
|
|
int *action);
|
|
GdkDragAction nautilus_drag_default_drop_action_for_netscape_url (GdkDragContext *context);
|
|
GdkDragAction nautilus_drag_default_drop_action_for_uri_list (GdkDragContext *context,
|
|
const char *target_uri_string);
|
|
gboolean nautilus_drag_drag_data_get (GtkWidget *widget,
|
|
GdkDragContext *context,
|
|
GtkSelectionData *selection_data,
|
|
guint info,
|
|
guint32 time,
|
|
gpointer container_context,
|
|
NautilusDragEachSelectedItemIterator each_selected_item_iterator);
|
|
int nautilus_drag_modifier_based_action (int default_action,
|
|
int non_default_action);
|
|
|
|
GdkDragAction nautilus_drag_drop_action_ask (GtkWidget *widget,
|
|
GdkDragAction possible_actions);
|
|
GdkDragAction nautilus_drag_drop_background_ask (GtkWidget *widget,
|
|
GdkDragAction possible_actions);
|
|
|
|
gboolean nautilus_drag_autoscroll_in_scroll_region (GtkWidget *widget);
|
|
void nautilus_drag_autoscroll_calculate_delta (GtkWidget *widget,
|
|
float *x_scroll_delta,
|
|
float *y_scroll_delta);
|
|
void nautilus_drag_autoscroll_start (NautilusDragInfo *drag_info,
|
|
GtkWidget *widget,
|
|
GtkFunction callback,
|
|
gpointer user_data);
|
|
void nautilus_drag_autoscroll_stop (NautilusDragInfo *drag_info);
|
|
|
|
gboolean nautilus_drag_selection_includes_special_link (GList *selection_list);
|
|
|
|
void nautilus_drag_slot_proxy_init (GtkWidget *widget,
|
|
NautilusDragSlotProxyInfo *drag_info);
|
|
|
|
#endif
|