mirror of
https://gitlab.gnome.org/GNOME/gparted
synced 2024-08-27 11:40:03 +00:00
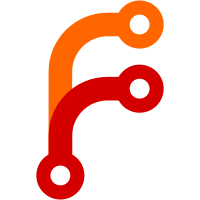
Provide and use a single interface for getting the file system string for display, regardless of whether the partition is encrypted or the encryption mapping is active or not. Example return values for get_filesystem_string() for different types and states of Partition objects: 1) Plain ext4 file system: -> "ext4" 2) Closed encrypted: -> "[Encrypted]" 3) Open encrypted ext4 file system: -> "[Encrypted] ext4" This simplifies the code in TreeView_Detail::create_row() which sets the file system type displayed in the main window. The same method will then also be used when setting the operation description as each operation is updated to handle encrypted file systems. Bug 774818 - Implement LUKS read-write actions NOT requiring a passphrase
67 lines
1.9 KiB
C++
67 lines
1.9 KiB
C++
/* Copyright (C) 2015 Mike Fleetwood
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
|
|
#ifndef GPARTED_PARTITIONLUKS_H
|
|
#define GPARTED_PARTITIONLUKS_H
|
|
|
|
#include "Partition.h"
|
|
#include "Utils.h"
|
|
|
|
#include <glibmm/ustring.h>
|
|
|
|
namespace GParted
|
|
{
|
|
|
|
class PartitionLUKS : public Partition
|
|
{
|
|
public:
|
|
PartitionLUKS();
|
|
virtual ~PartitionLUKS();
|
|
virtual PartitionLUKS * clone() const;
|
|
|
|
void set_luks( const Glib::ustring & path,
|
|
FILESYSTEM fstype,
|
|
Sector header_size,
|
|
Sector mapping_size,
|
|
Byte_Value sector_size,
|
|
bool busy );
|
|
|
|
Partition & get_encrypted() { return encrypted; };
|
|
const Partition & get_encrypted() const { return encrypted; };
|
|
|
|
virtual bool sector_usage_known() const;
|
|
virtual Sector estimated_min_size() const;
|
|
virtual Sector get_sectors_used() const;
|
|
virtual Sector get_sectors_unused() const;
|
|
virtual Sector get_sectors_unallocated() const;
|
|
virtual bool have_messages() const;
|
|
virtual std::vector<Glib::ustring> get_messages() const;
|
|
virtual void clear_messages();
|
|
|
|
virtual const Partition & get_filesystem_partition() const;
|
|
|
|
virtual const Glib::ustring get_filesystem_string() const;
|
|
|
|
private:
|
|
Partition encrypted;
|
|
Sector header_size; // Size of the LUKS header (everything up to the start of the mapping)
|
|
};
|
|
|
|
}//GParted
|
|
|
|
#endif /* GPARTED_PARTITIONLUKS_H */
|