mirror of
https://gitlab.gnome.org/GNOME/gparted
synced 2024-09-20 00:11:42 +00:00
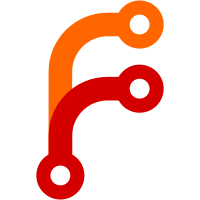
Background
GParted stored a list of paths for Device and Partition objects. It
sorted this list [1][2] and treated the first specially as that is what
get_path() returned and was used almost everywhere; with the file system
specific tools, looked up in various *_Info caches, etc.
[1] Device::add_path(), ::add_paths()
[2] Partition::add_path(), ::add_paths()
Mount point display [3] was the only bit of GParted which really worked
with the path list. Busy file system detection [4] just used the path
provided by libparted, or for LUKS /dev/mapper/* names. It checked that
single path against the mounted file systems found from /proc/mounts,
expanded with additional block device names when symlinks were
encountered.
[3] GParted_Core::set_mountpoints() -> set_mountpoints_helper()
[4] GParted_Core::set_device_partitions() -> is_busy()
GParted_Core::set_device_one_partition() -> is_busy()
GParted_Core::set_luks_partition() -> is_busy()
Having the first path, by sort order, treated specially by being used
everywhere and virtually ignoring the others was wrong, complicated to
remember and difficult code with. As all the additional paths were
virtually unused and made no difference, remove them. The "improved
detection of mountpoins, free space, etc.." benefit from commit [5]
doesn't seem to exist. Therefore simplify to a single path for Device
and Partition objects.
[5] commit 6d8b169e73
changed the way devices and partitions store their device paths.
Instead of holding a 'realpath' and a symbolic path we store paths
in a list. This allows for improved detection of mountpoins, free
space, etc..
This patch
Simplify the Device object from a vector of paths to a single path.
Remove add_paths() and get_paths() methods. Keep add_path() and
get_path() for now.
Bug 767842 - File system usage missing when tools report alternate block
device names
67 lines
1.8 KiB
C++
67 lines
1.8 KiB
C++
/* Copyright (C) 2004 Bart
|
|
* Copyright (C) 2010 Curtis Gedak
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef GPARTED_DEVICE_H
|
|
#define GPARTED_DEVICE_H
|
|
|
|
#include "../include/Partition.h"
|
|
#include "../include/PartitionVector.h"
|
|
|
|
namespace GParted
|
|
{
|
|
|
|
class Device
|
|
{
|
|
|
|
public:
|
|
Device() ;
|
|
~Device() ;
|
|
|
|
Device get_copy_without_partitions() const;
|
|
void add_path( const Glib::ustring & path, bool clear_paths = false ) ;
|
|
Glib::ustring get_path() const ;
|
|
void enable_partition_naming( int length ); // > 0 => enable partition naming support
|
|
bool partition_naming_supported() const;
|
|
int get_max_partition_name_length() const;
|
|
|
|
bool operator==( const Device & device ) const ;
|
|
bool operator!=( const Device & device ) const ;
|
|
|
|
void Reset() ;
|
|
PartitionVector partitions;
|
|
Sector length;
|
|
Sector heads ;
|
|
Sector sectors ;
|
|
Sector cylinders ;
|
|
Sector cylsize ;
|
|
Glib::ustring model;
|
|
Glib::ustring serial_number;
|
|
Glib::ustring disktype;
|
|
int sector_size ;
|
|
int max_prims ;
|
|
int highest_busy ;
|
|
bool readonly ;
|
|
|
|
private:
|
|
Glib::ustring path;
|
|
int max_partition_name_length; // > 0 => naming of partitions is supported on this device
|
|
};
|
|
|
|
} //GParted
|
|
|
|
#endif /* GPARTED_DEVICE_H */
|