mirror of
https://gitlab.gnome.org/GNOME/gparted
synced 2024-10-01 13:44:34 +00:00
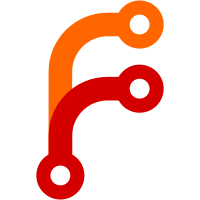
So far GParted is still loading the default non-reversible encoded labels from blkid in the initial loading of the FS_Info module cache. This encoded label is used to match LABEL=<label> when reading /etc/fstab, via the get_path_by_label() call, so works for ASCII only labels. This prevents GParted enabling the "mount on >" partition menu item when non-ASCII labels are used. To fix this: 1) Stop reading the labels the wrong way. Via the blkid command used to initially load the FS_Info module cache and is subject to default non-reversible encoding of non-printable ASCII bytes. 2) Read all the labels the right way, but only when needed. Only when /etc/fstab file contains LABEL=<label> and get_path_by_label() is called, read all the labels from blkid without encoding them via run_blkid_update_cache_one_label(). 3) Return label from the cache. get_label() returns the cached label, loading it into the cache first if needed with run_blkid_update_cache_one_label(). In the worst case scenario of having a LABEL=<label> in /etc/fstab blkid will be run for every partition containing a recognised file system to read the label. On my desktop with 5 hard drives, 4 SWRaid arrays and 31 recognised file systems running 'blkid -o value -s LABEL ...' 31 times took 0.074 seconds of a total scan time of 9.072 seconds. Less that 1% of the total scanning time. When LABEL=<label> is not used in /etc/fstab individual blkid executions are only used to read labels for file systems where there is no file system specific tool available reducing the impact further. Blkid itself caches the data in it's blkid.tab cache file rather than reading all file systems on each invocation. Also the Linux file system cache will already contain the blkid executable file, needed libraries files and the blkid.tab cache file itself. Hence why repeated execution of blkid is so fast. Further to the updated comment in set_partition_label_and_uuid(). Matching LABEL=<label> from /etc/fstab uses the label obtained from blkid run in the C locale so this kind of assumes it returns the label correctly and it does for my limited testing on Unicode enabled desktops. Just not sure if it would be true for all cases in all locales compared to the FS specific command run in the users default locale. Bug 786502 - Support reading Unicode labels when file system specific tools aren't available
67 lines
2 KiB
C++
67 lines
2 KiB
C++
/* Copyright (C) 2008, 2009, 2010 Curtis Gedak
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef GPARTED_FS_INFO_H
|
|
#define GPARTED_FS_INFO_H
|
|
|
|
#include "BlockSpecial.h"
|
|
|
|
#include <glibmm/ustring.h>
|
|
#include <vector>
|
|
|
|
namespace GParted
|
|
{
|
|
|
|
struct FS_Entry
|
|
{
|
|
BlockSpecial path;
|
|
Glib::ustring type;
|
|
Glib::ustring sec_type;
|
|
Glib::ustring uuid;
|
|
bool have_label;
|
|
Glib::ustring label;
|
|
};
|
|
|
|
class FS_Info
|
|
{
|
|
public:
|
|
static void load_cache();
|
|
static Glib::ustring get_fs_type( const Glib::ustring & path );
|
|
static Glib::ustring get_label( const Glib::ustring & path, bool & found );
|
|
static Glib::ustring get_uuid( const Glib::ustring & path );
|
|
static Glib::ustring get_path_by_uuid( const Glib::ustring & uuid );
|
|
static Glib::ustring get_path_by_label( const Glib::ustring & label );
|
|
|
|
private:
|
|
static void initialize_if_required();
|
|
static void set_commands_found();
|
|
static const FS_Entry & get_cache_entry_by_path( const Glib::ustring & path );
|
|
static void load_fs_info_cache();
|
|
static void load_fs_info_cache_extra_for_path( const Glib::ustring & path );
|
|
static bool run_blkid_load_cache( const Glib::ustring & path = "" );
|
|
static void update_fs_info_cache_all_labels();
|
|
static bool run_blkid_update_cache_one_label( FS_Entry & fs_entry );
|
|
|
|
static bool fs_info_cache_initialized ;
|
|
static bool blkid_found ;
|
|
static bool need_blkid_vfat_cache_update_workaround;
|
|
static std::vector<FS_Entry> fs_info_cache;
|
|
};
|
|
|
|
}//GParted
|
|
|
|
#endif /* GPARTED_FS_INFO_H */
|