mirror of
https://github.com/RPCS3/rpcs3
synced 2024-09-15 22:30:28 +00:00
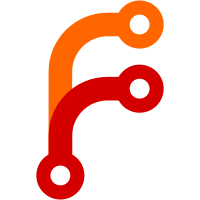
- Beware of clone operations. Blindly inheriting the parent's metadata is wrong. - It is possible, especially when reusing a pre-existing slice, that the parent and child info has diverged
47 lines
889 B
C++
47 lines
889 B
C++
#pragma once
|
|
#include <util/types.hpp>
|
|
#include <util/logs.hpp>
|
|
|
|
namespace utils
|
|
{
|
|
namespace stack_trace
|
|
{
|
|
// Printing utilities
|
|
|
|
template <typename T>
|
|
concept Logger = requires (T& t, const std::string& msg)
|
|
{
|
|
{ t.print(msg) };
|
|
};
|
|
|
|
struct print_to_log
|
|
{
|
|
logs::channel& log;
|
|
|
|
public:
|
|
print_to_log(logs::channel& chan)
|
|
: log(chan)
|
|
{}
|
|
|
|
void print(const std::string& s)
|
|
{
|
|
log.error("%s", s);
|
|
}
|
|
};
|
|
}
|
|
|
|
std::vector<void*> get_backtrace(int max_depth = 255);
|
|
std::vector<std::string> get_backtrace_symbols(const std::vector<void*>& stack);
|
|
|
|
FORCE_INLINE void print_trace(stack_trace::Logger auto& logger, int max_depth = 255)
|
|
{
|
|
const auto trace = get_backtrace(max_depth);
|
|
const auto lines = get_backtrace_symbols(trace);
|
|
|
|
for (const auto& line : lines)
|
|
{
|
|
logger.print(line);
|
|
}
|
|
}
|
|
}
|