mirror of
https://github.com/godotengine/godot
synced 2024-09-05 19:49:39 +00:00
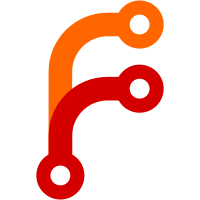
- Updates C# signal documentation and code examples to the new API in 4.0 - Replace all `nameof` usages with the exposed `StringName`
960 lines
49 KiB
XML
960 lines
49 KiB
XML
<?xml version="1.0" encoding="UTF-8" ?>
|
|
<class name="Object" version="4.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../class.xsd">
|
|
<brief_description>
|
|
Base class for all other classes in the engine.
|
|
</brief_description>
|
|
<description>
|
|
An advanced [Variant] type. All classes in the engine inherit from Object. Each class may define new properties, methods or signals, which are available to all inheriting classes. For example, a [Sprite2D] instance is able to call [method Node.add_child] because it inherits from [Node].
|
|
You can create new instances, using [code]Object.new()[/code] in GDScript, or [code]new Object[/code] in C#.
|
|
To delete an Object instance, call [method free]. This is necessary for most classes inheriting Object, because they do not manage memory on their own, and will otherwise cause memory leaks when no longer in use. There are a few classes that perform memory management. For example, [RefCounted] (and by extension [Resource]) deletes itself when no longer referenced, and [Node] deletes its children when freed.
|
|
Objects can have a [Script] attached to them. Once the [Script] is instantiated, it effectively acts as an extension to the base class, allowing it to define and inherit new properties, methods and signals.
|
|
Inside a [Script], [method _get_property_list] may be overridden to customize properties in several ways. This allows them to be available to the editor, display as lists of options, sub-divide into groups, save on disk, etc. Scripting languages offer easier ways to customize properties, such as with the [annotation @GDScript.@export] annotation.
|
|
Godot is very dynamic. An object's script, and therefore its properties, methods and signals, can be changed at run-time. Because of this, there can be occasions where, for example, a property required by a method may not exist. To prevent run-time errors, see methods such as [method set], [method get], [method call], [method has_method], [method has_signal], etc. Note that these methods are [b]much[/b] slower than direct references.
|
|
In GDScript, you can also check if a given property, method, or signal name exists in an object with the [code]in[/code] operator:
|
|
[codeblock]
|
|
var node = Node.new()
|
|
print("name" in node) # Prints true
|
|
print("get_parent" in node) # Prints true
|
|
print("tree_entered" in node) # Prints true
|
|
print("unknown" in node) # Prints false
|
|
[/codeblock]
|
|
Notifications are [int] constants commonly sent and received by objects. For example, on every rendered frame, the [SceneTree] notifies nodes inside the tree with a [constant Node.NOTIFICATION_PROCESS]. The nodes receive it and may call [method Node._process] to update. To make use of notifications, see [method notification] and [method _notification].
|
|
Lastly, every object can also contain metadata (data about data). [method set_meta] can be useful to store information that the object itself does not depend on. To keep your code clean, making excessive use of metadata is discouraged.
|
|
[b]Note:[/b] Unlike references to a [RefCounted], references to an object stored in a variable can become invalid without being set to [code]null[/code]. To check if an object has been deleted, do [i]not[/i] compare it against [code]null[/code]. Instead, use [method @GlobalScope.is_instance_valid]. It's also recommended to inherit from [RefCounted] for classes storing data instead of [Object].
|
|
[b]Note:[/b] The [code]script[/code] is not exposed like most properties. To set or get an object's [Script] in code, use [method set_script] and [method get_script], respectively.
|
|
</description>
|
|
<tutorials>
|
|
<link title="Object class introduction">$DOCS_URL/contributing/development/core_and_modules/object_class.html</link>
|
|
<link title="When and how to avoid using nodes for everything">$DOCS_URL/tutorials/best_practices/node_alternatives.html</link>
|
|
<link title="Object notifications">$DOCS_URL/tutorials/best_practices/godot_notifications.html</link>
|
|
</tutorials>
|
|
<methods>
|
|
<method name="_get" qualifiers="virtual">
|
|
<return type="Variant" />
|
|
<param index="0" name="property" type="StringName" />
|
|
<description>
|
|
Override this method to customize the behavior of [method get]. Should return the given [param property]'s value, or [code]null[/code] if the [param property] should be handled normally.
|
|
Combined with [method _set] and [method _get_property_list], this method allows defining custom properties, which is particularly useful for editor plugins. Note that a property must be present in [method get_property_list], otherwise this method will not be called.
|
|
[codeblocks]
|
|
[gdscript]
|
|
func _get(property):
|
|
if (property == "fake_property"):
|
|
print("Getting my property!")
|
|
return 4
|
|
|
|
func _get_property_list():
|
|
return [
|
|
{ "name": "fake_property", "type": TYPE_INT }
|
|
]
|
|
[/gdscript]
|
|
[csharp]
|
|
public override Variant _Get(StringName property)
|
|
{
|
|
if (property == "FakeProperty")
|
|
{
|
|
GD.Print("Getting my property!");
|
|
return 4;
|
|
}
|
|
return default;
|
|
}
|
|
|
|
public override Godot.Collections.Array<Godot.Collections.Dictionary> _GetPropertyList()
|
|
{
|
|
return new Godot.Collections.Array<Godot.Collections.Dictionary>()
|
|
{
|
|
new Godot.Collections.Dictionary()
|
|
{
|
|
{ "name", "FakeProperty" },
|
|
{ "type", (int)Variant.Type.Int }
|
|
}
|
|
};
|
|
}
|
|
[/csharp]
|
|
[/codeblocks]
|
|
</description>
|
|
</method>
|
|
<method name="_get_property_list" qualifiers="virtual">
|
|
<return type="Dictionary[]" />
|
|
<description>
|
|
Override this method to customize how script properties should be handled by the engine.
|
|
Should return a property list, as an [Array] of dictionaries. The result is added to the array of [method get_property_list], and should be formatted in the same way. Each [Dictionary] must at least contain the [code]name[/code] and [code]type[/code] entries.
|
|
The example below displays [code]hammer_type[/code] in the Inspector dock, only if [code]holding_hammer[/code] is [code]true[/code]:
|
|
[codeblocks]
|
|
[gdscript]
|
|
@tool
|
|
extends Node2D
|
|
|
|
@export var holding_hammer = false:
|
|
set(value):
|
|
holding_hammer = value
|
|
notify_property_list_changed()
|
|
var hammer_type = 0
|
|
|
|
func _get_property_list():
|
|
# By default, `hammer_type` is not visible in the editor.
|
|
var property_usage = PROPERTY_USAGE_NO_EDITOR
|
|
|
|
if holding_hammer:
|
|
property_usage = PROPERTY_USAGE_DEFAULT
|
|
|
|
var properties = []
|
|
properties.append({
|
|
"name": "hammer_type",
|
|
"type": TYPE_INT,
|
|
"usage": property_usage, # See above assignment.
|
|
"hint": PROPERTY_HINT_ENUM,
|
|
"hint_string": "Wooden,Iron,Golden,Enchanted"
|
|
})
|
|
|
|
return properties
|
|
[/gdscript]
|
|
[csharp]
|
|
[Tool]
|
|
public class MyNode2D : Node2D
|
|
{
|
|
private bool _holdingHammer;
|
|
|
|
[Export]
|
|
public bool HoldingHammer
|
|
{
|
|
get => _holdingHammer;
|
|
set
|
|
{
|
|
_holdingHammer = value;
|
|
NotifyPropertyListChanged();
|
|
}
|
|
}
|
|
|
|
public int HammerType { get; set; }
|
|
|
|
public override Godot.Collections.Array<Godot.Collections.Dictionary> _GetPropertyList()
|
|
{
|
|
// By default, `HammerType` is not visible in the editor.
|
|
var propertyUsage = PropertyUsageFlags.NoEditor;
|
|
|
|
if (HoldingHammer)
|
|
{
|
|
propertyUsage = PropertyUsageFlags.Default;
|
|
}
|
|
|
|
var properties = new Godot.Collections.Array<Godot.Collections.Dictionary>();
|
|
properties.Add(new Godot.Collections.Dictionary()
|
|
{
|
|
{ "name", "HammerType" },
|
|
{ "type", (int)Variant.Type.Int },
|
|
{ "usage", (int)propertyUsage }, // See above assignment.
|
|
{ "hint", (int)PropertyHint.Enum },
|
|
{ "hint_string", "Wooden,Iron,Golden,Enchanted" }
|
|
});
|
|
|
|
return properties;
|
|
}
|
|
}
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b]Note:[/b] This method is intended for advanced purposes. For most common use cases, the scripting languages offer easier ways to handle properties. See [annotation @GDScript.@export], [annotation @GDScript.@export_enum], [annotation @GDScript.@export_group], etc.
|
|
[b]Note:[/b] If the object's script is not [annotation @GDScript.@tool], this method will not be called in the editor.
|
|
</description>
|
|
</method>
|
|
<method name="_init" qualifiers="virtual">
|
|
<return type="void" />
|
|
<description>
|
|
Called when the object's script is instantiated, oftentimes after the object is initialized in memory (through [code]Object.new()[/code] in GDScript, or [code]new Object[/code] in C#). It can be also defined to take in parameters. This method is similar to a constructor in most programming languages.
|
|
[b]Note:[/b] If [method _init] is defined with [i]required[/i] parameters, the Object with script may only be created directly. If any other means (such as [method PackedScene.instantiate] or [method Node.duplicate]) are used, the script's initialization will fail.
|
|
</description>
|
|
</method>
|
|
<method name="_notification" qualifiers="virtual">
|
|
<return type="void" />
|
|
<param index="0" name="what" type="int" />
|
|
<description>
|
|
Called when the object receives a notification, which can be identified in [param what] by comparing it with a constant. See also [method notification].
|
|
[codeblocks]
|
|
[gdscript]
|
|
func _notification(what):
|
|
if what == NOTIFICATION_PREDELETE:
|
|
print("Goodbye!")
|
|
[/gdscript]
|
|
[csharp]
|
|
public override void _Notification(long what)
|
|
{
|
|
if (what == NotificationPredelete)
|
|
{
|
|
GD.Print("Goodbye!");
|
|
}
|
|
}
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b]Note:[/b] The base [Object] defines a few notifications ([constant NOTIFICATION_POSTINITIALIZE] and [constant NOTIFICATION_PREDELETE]). Inheriting classes such as [Node] define a lot more notifications, which are also received by this method.
|
|
</description>
|
|
</method>
|
|
<method name="_property_can_revert" qualifiers="virtual">
|
|
<return type="bool" />
|
|
<param index="0" name="property" type="StringName" />
|
|
<description>
|
|
Override this method to customize the given [param property]'s revert behavior. Should return [code]true[/code] if the [param property] can be reverted in the Inspector dock. Use [method _property_get_revert] to specify the [param property]'s default value.
|
|
[b]Note:[/b] This method must return consistently, regardless of the current value of the [param property].
|
|
</description>
|
|
</method>
|
|
<method name="_property_get_revert" qualifiers="virtual">
|
|
<return type="Variant" />
|
|
<param index="0" name="property" type="StringName" />
|
|
<description>
|
|
Override this method to customize the given [param property]'s revert behavior. Should return the default value for the [param property]. If the default value differs from the [param property]'s current value, a revert icon is displayed in the Inspector dock.
|
|
[b]Note:[/b] [method _property_can_revert] must also be overridden for this method to be called.
|
|
</description>
|
|
</method>
|
|
<method name="_set" qualifiers="virtual">
|
|
<return type="bool" />
|
|
<param index="0" name="property" type="StringName" />
|
|
<param index="1" name="value" type="Variant" />
|
|
<description>
|
|
Override this method to customize the behavior of [method set]. Should set the [param property] to [param value] and return [code]true[/code], or [code]false[/code] if the [param property] should be handled normally. The [i]exact[/i] way to set the [param property] is up to this method's implementation.
|
|
Combined with [method _get] and [method _get_property_list], this method allows defining custom properties, which is particularly useful for editor plugins. Note that a property [i]must[/i] be present in [method get_property_list], otherwise this method will not be called.
|
|
[codeblocks]
|
|
[gdscript]
|
|
func _set(property, value):
|
|
if (property == "fake_property"):
|
|
print("Setting my property to ", value)
|
|
|
|
func _get_property_list():
|
|
return [
|
|
{ "name": "fake_property", "type": TYPE_INT }
|
|
]
|
|
[/gdscript]
|
|
[csharp]
|
|
public override void _Set(StringName property, Variant value)
|
|
{
|
|
if (property == "FakeProperty")
|
|
{
|
|
GD.Print($"Setting my property to {value}");
|
|
return true;
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
public override Godot.Collections.Array<Godot.Collections.Dictionary> _GetPropertyList()
|
|
{
|
|
return new Godot.Collections.Array<Godot.Collections.Dictionary>()
|
|
{
|
|
new Godot.Collections.Dictionary()
|
|
{
|
|
{ "name", "FakeProperty" },
|
|
{ "type", (int)Variant.Type.Int }
|
|
}
|
|
};
|
|
}
|
|
[/csharp]
|
|
[/codeblocks]
|
|
</description>
|
|
</method>
|
|
<method name="_to_string" qualifiers="virtual">
|
|
<return type="String" />
|
|
<description>
|
|
Override this method to customize the return value of [method to_string], and therefore the object's representation as a [String].
|
|
[codeblock]
|
|
func _to_string():
|
|
return "Welcome to Godot 4!"
|
|
|
|
func _init():
|
|
print(self) # Prints Welcome to Godot 4!"
|
|
var a = str(self) # a is "Welcome to Godot 4!"
|
|
[/codeblock]
|
|
</description>
|
|
</method>
|
|
<method name="add_user_signal">
|
|
<return type="void" />
|
|
<param index="0" name="signal" type="String" />
|
|
<param index="1" name="arguments" type="Array" default="[]" />
|
|
<description>
|
|
Adds a user-defined [param signal]. Optional arguments for the signal can be added as an [Array] of dictionaries, each defining a [code]name[/code] [String] and a [code]type[/code] [int] (see [enum Variant.Type]). See also [method has_user_signal].
|
|
[codeblocks]
|
|
[gdscript]
|
|
add_user_signal("hurt", [
|
|
{ "name": "damage", "type": TYPE_INT },
|
|
{ "name": "source", "type": TYPE_OBJECT }
|
|
])
|
|
[/gdscript]
|
|
[csharp]
|
|
AddUserSignal("Hurt", new Godot.Collections.Array()
|
|
{
|
|
new Godot.Collections.Dictionary()
|
|
{
|
|
{ "name", "damage" },
|
|
{ "type", (int)Variant.Type.Int }
|
|
},
|
|
new Godot.Collections.Dictionary()
|
|
{
|
|
{ "name", "source" },
|
|
{ "type", (int)Variant.Type.Object }
|
|
}
|
|
});
|
|
[/csharp]
|
|
[/codeblocks]
|
|
</description>
|
|
</method>
|
|
<method name="call" qualifiers="vararg">
|
|
<return type="Variant" />
|
|
<param index="0" name="method" type="StringName" />
|
|
<description>
|
|
Calls the [param method] on the object and returns the result. This method supports a variable number of arguments, so parameters can be passed as a comma separated list.
|
|
[codeblocks]
|
|
[gdscript]
|
|
var node = Node3D.new()
|
|
node.call("rotate", Vector3(1.0, 0.0, 0.0), 1.571)
|
|
[/gdscript]
|
|
[csharp]
|
|
var node = new Node3D();
|
|
node.Call(Node3D.MethodName.Rotate, new Vector3(1f, 0f, 0f), 1.571f);
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b]Note:[/b] In C#, [param method] must be in snake_case when referring to built-in Godot methods. Prefer using the names exposed in the [code]MethodName[/code] class to avoid allocating a new [StringName] on each call.
|
|
</description>
|
|
</method>
|
|
<method name="call_deferred" qualifiers="vararg">
|
|
<return type="Variant" />
|
|
<param index="0" name="method" type="StringName" />
|
|
<description>
|
|
Calls the [param method] on the object during idle time. This method supports a variable number of arguments, so parameters can be passed as a comma separated list.
|
|
[codeblocks]
|
|
[gdscript]
|
|
var node = Node3D.new()
|
|
node.call_deferred("rotate", Vector3(1.0, 0.0, 0.0), 1.571)
|
|
[/gdscript]
|
|
[csharp]
|
|
var node = new Node3D();
|
|
node.CallDeferred(Node3D.MethodName.Rotate, new Vector3(1f, 0f, 0f), 1.571f);
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b]Note:[/b] In C#, [param method] must be in snake_case when referring to built-in Godot methods. Prefer using the names exposed in the [code]MethodName[/code] class to avoid allocating a new [StringName] on each call.
|
|
</description>
|
|
</method>
|
|
<method name="callv">
|
|
<return type="Variant" />
|
|
<param index="0" name="method" type="StringName" />
|
|
<param index="1" name="arg_array" type="Array" />
|
|
<description>
|
|
Calls the [param method] on the object and returns the result. Unlike [method call], this method expects all parameters to be contained inside [param arg_array].
|
|
[codeblocks]
|
|
[gdscript]
|
|
var node = Node3D.new()
|
|
node.callv("rotate", [Vector3(1.0, 0.0, 0.0), 1.571])
|
|
[/gdscript]
|
|
[csharp]
|
|
var node = new Node3D();
|
|
node.Callv(Node3D.MethodName.Rotate, new Godot.Collections.Array { new Vector3(1f, 0f, 0f), 1.571f });
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b]Note:[/b] In C#, [param method] must be in snake_case when referring to built-in Godot methods. Prefer using the names exposed in the [code]MethodName[/code] class to avoid allocating a new [StringName] on each call
|
|
</description>
|
|
</method>
|
|
<method name="can_translate_messages" qualifiers="const">
|
|
<return type="bool" />
|
|
<description>
|
|
Returns [code]true[/code] if the object is allowed to translate messages with [method tr] and [method tr_n]. See also [method set_message_translation].
|
|
</description>
|
|
</method>
|
|
<method name="connect">
|
|
<return type="int" enum="Error" />
|
|
<param index="0" name="signal" type="StringName" />
|
|
<param index="1" name="callable" type="Callable" />
|
|
<param index="2" name="flags" type="int" default="0" />
|
|
<description>
|
|
Connects a [param signal] by name to a [param callable]. Optional [param flags] can be also added to configure the connection's behavior (see [enum ConnectFlags] constants).
|
|
A signal can only be connected once to the same [Callable]. If the signal is already connected, this method returns [constant ERR_INVALID_PARAMETER] and pushes an error message, unless the signal is connected with [constant CONNECT_REFERENCE_COUNTED]. To prevent this, use [method is_connected] first to check for existing connections.
|
|
If the [param callable]'s object is freed, the connection will be lost.
|
|
[b]Examples with recommended syntax:[/b]
|
|
Connecting signals is one of the most common operations in Godot and the API gives many options to do so, which are described further down. The code block below shows the recommended approach.
|
|
[codeblocks]
|
|
[gdscript]
|
|
func _ready():
|
|
var button = Button.new()
|
|
# `button_down` here is a Signal variant type, and we thus call the Signal.connect() method, not Object.connect().
|
|
# See discussion below for a more in-depth overview of the API.
|
|
button.button_down.connect(_on_button_down)
|
|
|
|
# This assumes that a `Player` class exists, which defines a `hit` signal.
|
|
var player = Player.new()
|
|
# We use Signal.connect() again, and we also use the Callable.bind() method,
|
|
# which returns a new Callable with the parameter binds.
|
|
player.hit.connect(_on_player_hit.bind("sword", 100))
|
|
|
|
func _on_button_down():
|
|
print("Button down!")
|
|
|
|
func _on_player_hit(weapon_type, damage):
|
|
print("Hit with weapon %s for %d damage." % [weapon_type, damage])
|
|
[/gdscript]
|
|
[csharp]
|
|
public override void _Ready()
|
|
{
|
|
var button = new Button();
|
|
// C# supports passing signals as events, so we can use this idiomatic construct:
|
|
button.ButtonDown += OnButtonDown;
|
|
|
|
// This assumes that a `Player` class exists, which defines a `Hit` signal.
|
|
var player = new Player();
|
|
// We can use lambdas when we need to bind additional parameters.
|
|
player.Hit += () => OnPlayerHit("sword", 100);
|
|
}
|
|
|
|
private void OnButtonDown()
|
|
{
|
|
GD.Print("Button down!");
|
|
}
|
|
|
|
private void OnPlayerHit(string weaponType, int damage)
|
|
{
|
|
GD.Print($"Hit with weapon {weaponType} for {damage} damage.");
|
|
}
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b][code]Object.connect()[/code] or [code]Signal.connect()[/code]?[/b]
|
|
As seen above, the recommended method to connect signals is not [method Object.connect]. The code block below shows the four options for connecting signals, using either this legacy method or the recommended [method Signal.connect], and using either an implicit [Callable] or a manually defined one.
|
|
[codeblocks]
|
|
[gdscript]
|
|
func _ready():
|
|
var button = Button.new()
|
|
# Option 1: Object.connect() with an implicit Callable for the defined function.
|
|
button.connect("button_down", _on_button_down)
|
|
# Option 2: Object.connect() with a constructed Callable using a target object and method name.
|
|
button.connect("button_down", Callable(self, "_on_button_down"))
|
|
# Option 3: Signal.connect() with an implicit Callable for the defined function.
|
|
button.button_down.connect(_on_button_down)
|
|
# Option 4: Signal.connect() with a constructed Callable using a target object and method name.
|
|
button.button_down.connect(Callable(self, "_on_button_down"))
|
|
|
|
func _on_button_down():
|
|
print("Button down!")
|
|
[/gdscript]
|
|
[csharp]
|
|
public override void _Ready()
|
|
{
|
|
var button = new Button();
|
|
// Option 1: In C#, we can use signals as events and connect with this idiomatic syntax:
|
|
button.ButtonDown += OnButtonDown;
|
|
// Option 2: Object.Connect() with a constructed Callable from a method group.
|
|
button.Connect(Button.SignalName.ButtonDown, Callable.From(OnButtonDown));
|
|
// Option 3: Object.Connect() with a constructed Callable using a target object and method name.
|
|
button.Connect(Button.SignalName.ButtonDown, new Callable(this, MethodName.OnButtonDown));
|
|
}
|
|
|
|
private void OnButtonDown()
|
|
{
|
|
GD.Print("Button down!");
|
|
}
|
|
[/csharp]
|
|
[/codeblocks]
|
|
While all options have the same outcome ([code]button[/code]'s [signal BaseButton.button_down] signal will be connected to [code]_on_button_down[/code]), [b]option 3[/b] offers the best validation: it will print a compile-time error if either the [code]button_down[/code] [Signal] or the [code]_on_button_down[/code] [Callable] are not defined. On the other hand, [b]option 2[/b] only relies on string names and will only be able to validate either names at runtime: it will print a runtime error if [code]"button_down"[/code] doesn't correspond to a signal, or if [code]"_on_button_down"[/code] is not a registered method in the object [code]self[/code]. The main reason for using options 1, 2, or 4 would be if you actually need to use strings (e.g. to connect signals programmatically based on strings read from a configuration file). Otherwise, option 3 is the recommended (and fastest) method.
|
|
[b]Binding and passing parameters:[/b]
|
|
The syntax to bind parameters is through [method Callable.bind], which returns a copy of the [Callable] with its parameters bound.
|
|
When calling [method emit_signal], the signal parameters can be also passed. The examples below show the relationship between these signal parameters and bound parameters.
|
|
[codeblocks]
|
|
[gdscript]
|
|
func _ready():
|
|
# This assumes that a `Player` class exists, which defines a `hit` signal.
|
|
var player = Player.new()
|
|
# Using Callable.bind().
|
|
player.hit.connect(_on_player_hit.bind("sword", 100))
|
|
|
|
# Parameters added when emitting the signal are passed first.
|
|
player.emit_signal("hit", "Dark lord", 5)
|
|
|
|
# We pass two arguments when emitting (`hit_by`, `level`),
|
|
# and bind two more arguments when connecting (`weapon_type`, `damage`).
|
|
func _on_player_hit(hit_by, level, weapon_type, damage):
|
|
print("Hit by %s (level %d) with weapon %s for %d damage." % [hit_by, level, weapon_type, damage])
|
|
[/gdscript]
|
|
[csharp]
|
|
public override void _Ready()
|
|
{
|
|
// This assumes that a `Player` class exists, which defines a `Hit` signal.
|
|
var player = new Player();
|
|
// Using lambda expressions that create a closure that captures the additional parameters.
|
|
// The lambda only receives the parameters defined by the signal's delegate.
|
|
player.Hit += (hitBy, level) => OnPlayerHit(hitBy, level, "sword", 100);
|
|
|
|
// Parameters added when emitting the signal are passed first.
|
|
player.EmitSignal(SignalName.Hit, "Dark lord", 5);
|
|
}
|
|
|
|
// We pass two arguments when emitting (`hit_by`, `level`),
|
|
// and bind two more arguments when connecting (`weapon_type`, `damage`).
|
|
private void OnPlayerHit(string hitBy, int level, string weaponType, int damage)
|
|
{
|
|
GD.Print($"Hit by {hitBy} (level {level}) with weapon {weaponType} for {damage} damage.");
|
|
}
|
|
[/csharp]
|
|
[/codeblocks]
|
|
</description>
|
|
</method>
|
|
<method name="disconnect">
|
|
<return type="void" />
|
|
<param index="0" name="signal" type="StringName" />
|
|
<param index="1" name="callable" type="Callable" />
|
|
<description>
|
|
Disconnects a [param signal] by name from a given [param callable]. If the connection does not exist, generates an error. Use [method is_connected] to make sure that the connection exists.
|
|
</description>
|
|
</method>
|
|
<method name="emit_signal" qualifiers="vararg">
|
|
<return type="int" enum="Error" />
|
|
<param index="0" name="signal" type="StringName" />
|
|
<description>
|
|
Emits the given [param signal] by name. The signal must exist, so it should be a built-in signal of this class or one of its inherited classes, or a user-defined signal (see [method add_user_signal]). This method supports a variable number of arguments, so parameters can be passed as a comma separated list.
|
|
Returns [constant ERR_UNAVAILABLE] if [param signal] does not exist or the parameters are invalid.
|
|
[codeblocks]
|
|
[gdscript]
|
|
emit_signal("hit", "sword", 100)
|
|
emit_signal("game_over")
|
|
[/gdscript]
|
|
[csharp]
|
|
EmitSignal(SignalName.Hit, "sword", 100);
|
|
EmitSignal(SignalName.GameOver);
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b]Note:[/b] In C#, [param signal] must be in snake_case when referring to built-in Godot signals. Prefer using the names exposed in the [code]SignalName[/code] class to avoid allocating a new [StringName] on each call.
|
|
</description>
|
|
</method>
|
|
<method name="free">
|
|
<return type="void" />
|
|
<description>
|
|
Deletes the object from memory. Pre-existing references to the object become invalid, and any attempt to access them will result in a run-time error. Checking the references with [method @GlobalScope.is_instance_valid] will return [code]false[/code].
|
|
</description>
|
|
</method>
|
|
<method name="get" qualifiers="const">
|
|
<return type="Variant" />
|
|
<param index="0" name="property" type="StringName" />
|
|
<description>
|
|
Returns the [Variant] value of the given [param property]. If the [param property] does not exist, this method returns [code]null[/code].
|
|
[codeblocks]
|
|
[gdscript]
|
|
var node = Node2D.new()
|
|
node.rotation = 1.5
|
|
var a = node.get("rotation") # a is 1.5
|
|
[/gdscript]
|
|
[csharp]
|
|
var node = new Node2D();
|
|
node.Rotation = 1.5f;
|
|
var a = node.Get("rotation"); // a is 1.5
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b]Note:[/b] In C#, [param property] must be in snake_case when referring to built-in Godot properties. Prefer using the names exposed in the [code]PropertyName[/code] class to avoid allocating a new [StringName] on each call.
|
|
</description>
|
|
</method>
|
|
<method name="get_class" qualifiers="const">
|
|
<return type="String" />
|
|
<description>
|
|
Returns the object's built-in class name, as a [String]. See also [method is_class].
|
|
[b]Note:[/b] This method ignores [code]class_name[/code] declarations. If this object's script has defined a [code]class_name[/code], the base, built-in class name is returned instead.
|
|
</description>
|
|
</method>
|
|
<method name="get_incoming_connections" qualifiers="const">
|
|
<return type="Dictionary[]" />
|
|
<description>
|
|
Returns an [Array] of signal connections received by this object. Each connection is represented as a [Dictionary] that contains three entries:
|
|
- [code]signal[/code] is a reference to the [Signal];
|
|
- [code]callable[/code] is a reference to the [Callable];
|
|
- [code]flags[/code] is a combination of [enum ConnectFlags].
|
|
</description>
|
|
</method>
|
|
<method name="get_indexed" qualifiers="const">
|
|
<return type="Variant" />
|
|
<param index="0" name="property_path" type="NodePath" />
|
|
<description>
|
|
Gets the object's property indexed by the given [param property_path]. The path should be a [NodePath] relative to the current object and can use the colon character ([code]:[/code]) to access nested properties.
|
|
[b]Examples:[/b] [code]"position:x"[/code] or [code]"material:next_pass:blend_mode"[/code].
|
|
[codeblocks]
|
|
[gdscript]
|
|
var node = Node2D.new()
|
|
node.position = Vector2(5, -10)
|
|
var a = node.get_indexed("position") # a is Vector2(5, -10)
|
|
var b = node.get_indexed("position:y") # b is -10
|
|
[/gdscript]
|
|
[csharp]
|
|
var node = new Node2D();
|
|
node.Position = new Vector2(5, -10);
|
|
var a = node.GetIndexed("position"); // a is Vector2(5, -10)
|
|
var b = node.GetIndexed("position:y"); // b is -10
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b]Note:[/b] In C#, [param property_path] must be in snake_case when referring to built-in Godot properties. Prefer using the names exposed in the [code]PropertyName[/code] class to avoid allocating a new [StringName] on each call.
|
|
[b]Note:[/b] This method does not support actual paths to nodes in the [SceneTree], only sub-property paths. In the context of nodes, use [method Node.get_node_and_resource] instead.
|
|
</description>
|
|
</method>
|
|
<method name="get_instance_id" qualifiers="const">
|
|
<return type="int" />
|
|
<description>
|
|
Returns the object's unique instance ID. This ID can be saved in [EncodedObjectAsID], and can be used to retrieve this object instance with [method @GlobalScope.instance_from_id].
|
|
</description>
|
|
</method>
|
|
<method name="get_meta" qualifiers="const">
|
|
<return type="Variant" />
|
|
<param index="0" name="name" type="StringName" />
|
|
<param index="1" name="default" type="Variant" default="null" />
|
|
<description>
|
|
Returns the object's metadata value for the given entry [param name]. If the entry does not exist, returns [param default]. If [param default] is [code]null[/code], an error is also generated.
|
|
[b]Note:[/b] Metadata that has a [param name] starting with an underscore ([code]_[/code]) is considered editor-only. Editor-only metadata is not displayed in the Inspector dock and should not be edited.
|
|
</description>
|
|
</method>
|
|
<method name="get_meta_list" qualifiers="const">
|
|
<return type="PackedStringArray" />
|
|
<description>
|
|
Returns the object's metadata entry names as a [PackedStringArray].
|
|
</description>
|
|
</method>
|
|
<method name="get_method_list" qualifiers="const">
|
|
<return type="Dictionary[]" />
|
|
<description>
|
|
Returns this object's methods and their signatures as an [Array] of dictionaries. Each [Dictionary] contains the following entries:
|
|
- [code]name[/code] is the name of the method, as a [String];
|
|
- [code]args[/code] is an [Array] of dictionaries representing the arguments;
|
|
- [code]default_args[/code] is the default arguments as an [Array] of variants;
|
|
- [code]flags[/code] is a combination of [enum MethodFlags];
|
|
- [code]id[/code] is the method's internal identifier [int];
|
|
- [code]return[/code] is the returned value, as a [Dictionary];
|
|
[b]Note:[/b] The dictionaries of [code]args[/code] and [code]return[/code] are formatted identically to the results of [method get_property_list], although not all entries are used.
|
|
</description>
|
|
</method>
|
|
<method name="get_property_list" qualifiers="const">
|
|
<return type="Dictionary[]" />
|
|
<description>
|
|
Returns the object's property list as an [Array] of dictionaries. Each [Dictionary] contains the following entries:
|
|
- [code]name[/code] is the property's name, as a [String];
|
|
- [code]class_name[/code] is an empty [StringName], unless the property is [constant TYPE_OBJECT] and it inherits from a class;
|
|
- [code]type[/code] is the property's type, as an [int] (see [enum Variant.Type]);
|
|
- [code]hint[/code] is [i]how[/i] the property is meant to be edited (see [enum PropertyHint]);
|
|
- [code]hint_string[/code] depends on the hint (see [enum PropertyHint]);
|
|
- [code]usage[/code] is a combination of [enum PropertyUsageFlags].
|
|
</description>
|
|
</method>
|
|
<method name="get_script" qualifiers="const">
|
|
<return type="Variant" />
|
|
<description>
|
|
Returns the object's [Script] instance, or [code]null[/code] if no script is attached.
|
|
</description>
|
|
</method>
|
|
<method name="get_signal_connection_list" qualifiers="const">
|
|
<return type="Dictionary[]" />
|
|
<param index="0" name="signal" type="StringName" />
|
|
<description>
|
|
Returns an [Array] of connections for the given [param signal] name. Each connection is represented as a [Dictionary] that contains three entries:
|
|
- [code]signal[/code] is a reference to the [Signal];
|
|
- [code]callable[/code] is a reference to the [Callable];
|
|
- [code]flags[/code] is a combination of [enum ConnectFlags].
|
|
</description>
|
|
</method>
|
|
<method name="get_signal_list" qualifiers="const">
|
|
<return type="Dictionary[]" />
|
|
<description>
|
|
Returns the list of existing signals as an [Array] of dictionaries.
|
|
[b]Note:[/b] Due of the implementation, each [Dictionary] is formatted very similarly to the returned values of [method get_method_list].
|
|
</description>
|
|
</method>
|
|
<method name="has_meta" qualifiers="const">
|
|
<return type="bool" />
|
|
<param index="0" name="name" type="StringName" />
|
|
<description>
|
|
Returns [code]true[/code] if a metadata entry is found with the given [param name]. See also [method get_meta], [method set_meta] and [method remove_meta].
|
|
[b]Note:[/b] Metadata that has a [param name] starting with an underscore ([code]_[/code]) is considered editor-only. Editor-only metadata is not displayed in the Inspector and should not be edited, although it can still be found by this method.
|
|
</description>
|
|
</method>
|
|
<method name="has_method" qualifiers="const">
|
|
<return type="bool" />
|
|
<param index="0" name="method" type="StringName" />
|
|
<description>
|
|
Returns [code]true[/code] if the the given [param method] name exists in the object.
|
|
[b]Note:[/b] In C#, [param method] must be in snake_case when referring to built-in Godot methods. Prefer using the names exposed in the [code]MethodName[/code] class to avoid allocating a new [StringName] on each call.
|
|
</description>
|
|
</method>
|
|
<method name="has_signal" qualifiers="const">
|
|
<return type="bool" />
|
|
<param index="0" name="signal" type="StringName" />
|
|
<description>
|
|
Returns [code]true[/code] if the given [param signal] name exists in the object.
|
|
[b]Note:[/b] In C#, [param signal] must be in snake_case when referring to built-in Godot methods. Prefer using the names exposed in the [code]SignalName[/code] class to avoid allocating a new [StringName] on each call.
|
|
</description>
|
|
</method>
|
|
<method name="has_user_signal" qualifiers="const">
|
|
<return type="bool" />
|
|
<param index="0" name="signal" type="StringName" />
|
|
<description>
|
|
Returns [code]true[/code] if the given user-defined [param signal] name exists. Only signals added with [method add_user_signal] are included.
|
|
</description>
|
|
</method>
|
|
<method name="is_blocking_signals" qualifiers="const">
|
|
<return type="bool" />
|
|
<description>
|
|
Returns [code]true[/code] if the object is blocking its signals from being emitted. See [method set_block_signals].
|
|
</description>
|
|
</method>
|
|
<method name="is_class" qualifiers="const">
|
|
<return type="bool" />
|
|
<param index="0" name="class" type="String" />
|
|
<description>
|
|
Returns [code]true[/code] if the object inherits from the given [param class]. See also [method get_class].
|
|
[codeblocks]
|
|
[gdscript]
|
|
var sprite2d = Sprite2D.new()
|
|
sprite2d.is_class("Sprite2D") # Returns true
|
|
sprite2d.is_class("Node") # Returns true
|
|
sprite2d.is_class("Node3D") # Returns false
|
|
[/gdscript]
|
|
[csharp]
|
|
var sprite2d = new Sprite2D();
|
|
sprite2d.IsClass("Sprite2D"); // Returns true
|
|
sprite2d.IsClass("Node"); // Returns true
|
|
sprite2d.IsClass("Node3D"); // Returns false
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b]Note:[/b] This method ignores [code]class_name[/code] declarations in the object's script.
|
|
</description>
|
|
</method>
|
|
<method name="is_connected" qualifiers="const">
|
|
<return type="bool" />
|
|
<param index="0" name="signal" type="StringName" />
|
|
<param index="1" name="callable" type="Callable" />
|
|
<description>
|
|
Returns [code]true[/code] if a connection exists between the given [param signal] name and [param callable].
|
|
[b]Note:[/b] In C#, [param signal] must be in snake_case when referring to built-in Godot methods. Prefer using the names exposed in the [code]SignalName[/code] class to avoid allocating a new [StringName] on each call.
|
|
</description>
|
|
</method>
|
|
<method name="is_queued_for_deletion" qualifiers="const">
|
|
<return type="bool" />
|
|
<description>
|
|
Returns [code]true[/code] if the [method Node.queue_free] method was called for the object.
|
|
</description>
|
|
</method>
|
|
<method name="notification">
|
|
<return type="void" />
|
|
<param index="0" name="what" type="int" />
|
|
<param index="1" name="reversed" type="bool" default="false" />
|
|
<description>
|
|
Sends the given [param what] notification to all classes inherited by the object, triggering calls to [method _notification], starting from the highest ancestor (the [Object] class) and going down to the object's script.
|
|
If [param reversed] is [code]true[/code], the call order is reversed.
|
|
[codeblocks]
|
|
[gdscript]
|
|
var player = Node2D.new()
|
|
player.set_script(load("res://player.gd"))
|
|
|
|
player.notification(NOTIFICATION_ENTER_TREE)
|
|
# The call order is Object -> Node -> Node2D -> player.gd.
|
|
|
|
player.notification(NOTIFICATION_ENTER_TREE, true)
|
|
# The call order is player.gd -> Node2D -> Node -> Object.
|
|
[/gdscript]
|
|
[csharp]
|
|
var player = new Node2D();
|
|
player.SetScript(GD.Load("res://player.gd"));
|
|
|
|
player.Notification(NotificationEnterTree);
|
|
// The call order is Object -> Node -> Node2D -> player.gd.
|
|
|
|
player.notification(NotificationEnterTree, true);
|
|
// The call order is player.gd -> Node2D -> Node -> Object.
|
|
[/csharp]
|
|
[/codeblocks]
|
|
</description>
|
|
</method>
|
|
<method name="notify_property_list_changed">
|
|
<return type="void" />
|
|
<description>
|
|
Emits the [signal property_list_changed] signal. This is mainly used to refresh the editor, so that the Inspector and editor plugins are properly updated.
|
|
</description>
|
|
</method>
|
|
<method name="property_can_revert" qualifiers="const">
|
|
<return type="bool" />
|
|
<param index="0" name="property" type="StringName" />
|
|
<description>
|
|
Returns [code]true[/code] if the given [param property] has a custom default value. Use [method property_get_revert] to get the [param property]'s default value.
|
|
[b]Note:[/b] This method is used by the Inspector dock to display a revert icon. The object must implement [method _property_can_revert] to customize the default value. If [method _property_can_revert] is not implemented, this method returns [code]false[/code].
|
|
</description>
|
|
</method>
|
|
<method name="property_get_revert" qualifiers="const">
|
|
<return type="Variant" />
|
|
<param index="0" name="property" type="StringName" />
|
|
<description>
|
|
Returns the custom default value of the given [param property]. Use [method property_can_revert] to check if the [param property] has a custom default value.
|
|
[b]Note:[/b] This method is used by the Inspector dock to display a revert icon. The object must implement [method _property_get_revert] to customize the default value. If [method _property_get_revert] is not implemented, this method returns [code]null[/code].
|
|
</description>
|
|
</method>
|
|
<method name="remove_meta">
|
|
<return type="void" />
|
|
<param index="0" name="name" type="StringName" />
|
|
<description>
|
|
Removes the given entry [param name] from the object's metadata. See also [method has_meta], [method get_meta] and [method set_meta].
|
|
[b]Note:[/b] Metadata that has a [param name] starting with an underscore ([code]_[/code]) is considered editor-only. Editor-only metadata is not displayed in the Inspector and should not be edited.
|
|
</description>
|
|
</method>
|
|
<method name="set">
|
|
<return type="void" />
|
|
<param index="0" name="property" type="StringName" />
|
|
<param index="1" name="value" type="Variant" />
|
|
<description>
|
|
Assigns [param value] to the given [param property]. If the property does not exist or the given [param value]'s type doesn't match, nothing happens.
|
|
[codeblocks]
|
|
[gdscript]
|
|
var node = Node2D.new()
|
|
node.set("global_scale", Vector2(8, 2.5))
|
|
print(node.global_scale) # Prints (8, 2.5)
|
|
[/gdscript]
|
|
[csharp]
|
|
var node = new Node2D();
|
|
node.Set("global_scale", new Vector2(8, 2.5));
|
|
GD.Print(node.GlobalScale); // Prints Vector2(8, 2.5)
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b]Note:[/b] In C#, [param property] must be in snake_case when referring to built-in Godot properties. Prefer using the names exposed in the [code]PropertyName[/code] class to avoid allocating a new [StringName] on each call.
|
|
</description>
|
|
</method>
|
|
<method name="set_block_signals">
|
|
<return type="void" />
|
|
<param index="0" name="enable" type="bool" />
|
|
<description>
|
|
If set to [code]true[/code], the object becomes unable to emit signals. As such, [method emit_signal] and signal connections will not work, until it is set to [code]false[/code].
|
|
</description>
|
|
</method>
|
|
<method name="set_deferred">
|
|
<return type="void" />
|
|
<param index="0" name="property" type="StringName" />
|
|
<param index="1" name="value" type="Variant" />
|
|
<description>
|
|
Assigns [param value] to the given [param property], after the current frame's physics step. This is equivalent to calling [method set] through [method call_deferred].
|
|
[codeblocks]
|
|
[gdscript]
|
|
var node = Node2D.new()
|
|
add_child(node)
|
|
|
|
node.rotation = 45.0
|
|
node.set_deferred("rotation", 90.0)
|
|
print(node.rotation) # Prints 45.0
|
|
|
|
await get_tree().process_frame
|
|
print(node.rotation) # Prints 90.0
|
|
[/gdscript]
|
|
[csharp]
|
|
var node = new Node2D();
|
|
node.Rotation = 45f;
|
|
node.SetDeferred("rotation", 90f);
|
|
GD.Print(node.Rotation); // Prints 45.0
|
|
|
|
await ToSignal(GetTree(), SceneTree.SignalName.ProcessFrame);
|
|
GD.Print(node.Rotation); // Prints 90.0
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b]Note:[/b] In C#, [param property] must be in snake_case when referring to built-in Godot properties. Prefer using the names exposed in the [code]PropertyName[/code] class to avoid allocating a new [StringName] on each call.
|
|
</description>
|
|
</method>
|
|
<method name="set_indexed">
|
|
<return type="void" />
|
|
<param index="0" name="property_path" type="NodePath" />
|
|
<param index="1" name="value" type="Variant" />
|
|
<description>
|
|
Assigns a new [param value] to the property identified by the [param property_path]. The path should be a [NodePath] relative to this object, and can use the colon character ([code]:[/code]) to access nested properties.
|
|
[codeblocks]
|
|
[gdscript]
|
|
var node = Node2D.new()
|
|
node.set_indexed("position", Vector2(42, 0))
|
|
node.set_indexed("position:y", -10)
|
|
print(node.position) # Prints (42, -10)
|
|
[/gdscript]
|
|
[csharp]
|
|
var node = new Node2D();
|
|
node.SetIndexed("position", new Vector2(42, 0));
|
|
node.SetIndexed("position:y", -10);
|
|
GD.Print(node.Position); // Prints (42, -10)
|
|
[/csharp]
|
|
[/codeblocks]
|
|
[b]Note:[/b] In C#, [param property_path] must be in snake_case when referring to built-in Godot properties. Prefer using the names exposed in the [code]PropertyName[/code] class to avoid allocating a new [StringName] on each call.
|
|
</description>
|
|
</method>
|
|
<method name="set_message_translation">
|
|
<return type="void" />
|
|
<param index="0" name="enable" type="bool" />
|
|
<description>
|
|
If set to [code]true[/code], allows the object to translate messages with [method tr] and [method tr_n]. Enabled by default. See also [method can_translate_messages].
|
|
</description>
|
|
</method>
|
|
<method name="set_meta">
|
|
<return type="void" />
|
|
<param index="0" name="name" type="StringName" />
|
|
<param index="1" name="value" type="Variant" />
|
|
<description>
|
|
Adds or changes the entry [param name] inside the object's metadata. The metadata [param value] can be any [Variant], although some types cannot be serialized correctly.
|
|
If [param value] is [code]null[/code], the entry is removed. This is the equivalent of using [method remove_meta]. See also [method has_meta] and [method get_meta].
|
|
[b]Note:[/b] Metadata that has a [param name] starting with an underscore ([code]_[/code]) is considered editor-only. Editor-only metadata is not displayed in the Inspector dock and should not be edited.
|
|
</description>
|
|
</method>
|
|
<method name="set_script">
|
|
<return type="void" />
|
|
<param index="0" name="script" type="Variant" />
|
|
<description>
|
|
Attaches [param script] to the object, and instantiates it. As a result, the script's [method _init] is called. A [Script] is used to extend the object's functionality.
|
|
If a script already exists, its instance is detached, and its property values and state are lost. Built-in property values are still kept.
|
|
</description>
|
|
</method>
|
|
<method name="to_string">
|
|
<return type="String" />
|
|
<description>
|
|
Returns a [String] representing the object. Defaults to [code]"<ClassName#RID>"[/code]. Override [method _to_string] to customize the string representation of the object.
|
|
</description>
|
|
</method>
|
|
<method name="tr" qualifiers="const">
|
|
<return type="String" />
|
|
<param index="0" name="message" type="StringName" />
|
|
<param index="1" name="context" type="StringName" default="""" />
|
|
<description>
|
|
Translates a [param message], using the translation catalogs configured in the Project Settings. Further [param context] can be specified to help with the translation.
|
|
If [method can_translate_messages] is [code]false[/code], or no translation is available, this method returns the [param message] without changes. See [method set_message_translation].
|
|
For detailed examples, see [url=$DOCS_URL/tutorials/i18n/internationalizing_games.html]Internationalizing games[/url].
|
|
</description>
|
|
</method>
|
|
<method name="tr_n" qualifiers="const">
|
|
<return type="String" />
|
|
<param index="0" name="message" type="StringName" />
|
|
<param index="1" name="plural_message" type="StringName" />
|
|
<param index="2" name="n" type="int" />
|
|
<param index="3" name="context" type="StringName" default="""" />
|
|
<description>
|
|
Translates a [param message] or [param plural_message], using the translation catalogs configured in the Project Settings. Further [param context] can be specified to help with the translation.
|
|
If [method can_translate_messages] is [code]false[/code], or no translation is available, this method returns [param message] or [param plural_message], without changes. See [method set_message_translation].
|
|
The [param n] is the number, or amount, of the message's subject. It is used by the translation system to fetch the correct plural form for the current language.
|
|
For detailed examples, see [url=$DOCS_URL/tutorials/i18n/localization_using_gettext.html]Localization using gettext[/url].
|
|
[b]Note:[/b] Negative and [float] numbers may not properly apply to some countable subjects. It's recommended handling these cases with [method tr].
|
|
</description>
|
|
</method>
|
|
</methods>
|
|
<signals>
|
|
<signal name="property_list_changed">
|
|
<description>
|
|
Emitted when [method notify_property_list_changed] is called.
|
|
</description>
|
|
</signal>
|
|
<signal name="script_changed">
|
|
<description>
|
|
Emitted when the object's script is changed.
|
|
[b]Note:[/b] When this signal is emitted, the new script is not initialized yet. If you need to access the new script, defer connections to this signal with [constant CONNECT_DEFERRED].
|
|
</description>
|
|
</signal>
|
|
</signals>
|
|
<constants>
|
|
<constant name="NOTIFICATION_POSTINITIALIZE" value="0">
|
|
Notification received when the object is initialized, before its script is attached. Used internally.
|
|
</constant>
|
|
<constant name="NOTIFICATION_PREDELETE" value="1">
|
|
Notification received when the object is about to be deleted. Can act as the deconstructor of some programming languages.
|
|
</constant>
|
|
<constant name="CONNECT_DEFERRED" value="1" enum="ConnectFlags">
|
|
Deferred connections trigger their [Callable]s on idle time, rather than instantly.
|
|
</constant>
|
|
<constant name="CONNECT_PERSIST" value="2" enum="ConnectFlags">
|
|
Persisting connections are stored when the object is serialized (such as when using [method PackedScene.pack]). In the editor, connections created through the Node dock are always persisting.
|
|
</constant>
|
|
<constant name="CONNECT_ONE_SHOT" value="4" enum="ConnectFlags">
|
|
One-shot connections disconnect themselves after emission.
|
|
</constant>
|
|
<constant name="CONNECT_REFERENCE_COUNTED" value="8" enum="ConnectFlags">
|
|
Reference-counted connections can be assigned to the same [Callable] multiple times. Each disconnection decreases the internal counter. The signal fully disconnects only when the counter reaches 0.
|
|
</constant>
|
|
</constants>
|
|
</class>
|