mirror of
https://github.com/godotengine/godot
synced 2024-11-02 12:55:22 +00:00
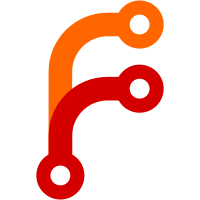
As a cryptographically secure random generator. Internally it uses mbedTLS CTR-DRBG implementation which gets re-seeded with entropy from OS::get_entropy when needed. CryptoCore now additionally depends on `ctr_drbg.c` and `entropy.c` thirdparty mbedtls files.
54 lines
1.8 KiB
Python
54 lines
1.8 KiB
Python
#!/usr/bin/env python
|
|
|
|
Import("env")
|
|
|
|
env_crypto = env.Clone()
|
|
|
|
is_builtin = env["builtin_mbedtls"]
|
|
has_module = env["module_mbedtls_enabled"]
|
|
thirdparty_obj = []
|
|
|
|
if is_builtin or not has_module:
|
|
# Use our headers for builtin or if the module is not going to be compiled.
|
|
# We decided not to depend on system mbedtls just for these few files that can
|
|
# be easily extracted.
|
|
env_crypto.Prepend(CPPPATH=["#thirdparty/mbedtls/include"])
|
|
|
|
# MbedTLS core functions (for CryptoCore).
|
|
# If the mbedtls module is compiled we don't need to add the .c files with our
|
|
# custom config since they will be built by the module itself.
|
|
# Only if the module is not enabled, we must compile here the required sources
|
|
# to make a "light" build with only the necessary mbedtls files.
|
|
if not has_module:
|
|
env_thirdparty = env_crypto.Clone()
|
|
env_thirdparty.disable_warnings()
|
|
# Custom config file
|
|
env_thirdparty.Append(
|
|
CPPDEFINES=[("MBEDTLS_CONFIG_FILE", '\\"thirdparty/mbedtls/include/godot_core_mbedtls_config.h\\"')]
|
|
)
|
|
thirdparty_mbedtls_dir = "#thirdparty/mbedtls/library/"
|
|
thirdparty_mbedtls_sources = [
|
|
"aes.c",
|
|
"base64.c",
|
|
"constant_time.c",
|
|
"ctr_drbg.c",
|
|
"entropy.c",
|
|
"md5.c",
|
|
"sha1.c",
|
|
"sha256.c",
|
|
"godot_core_mbedtls_platform.c",
|
|
]
|
|
thirdparty_mbedtls_sources = [thirdparty_mbedtls_dir + file for file in thirdparty_mbedtls_sources]
|
|
env_thirdparty.add_source_files(thirdparty_obj, thirdparty_mbedtls_sources)
|
|
env.core_sources += thirdparty_obj
|
|
|
|
|
|
# Godot source files
|
|
|
|
core_obj = []
|
|
|
|
env_crypto.add_source_files(core_obj, "*.cpp")
|
|
env.core_sources += core_obj
|
|
|
|
# Needed to force rebuilding the core files when the thirdparty library is updated.
|
|
env.Depends(core_obj, thirdparty_obj)
|