mirror of
https://github.com/Microsoft/vscode
synced 2024-10-28 14:18:54 +00:00
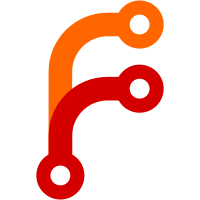
Adds a lint rule that ensures ensureNoDisposablesAreLeakedInTestSuite is called in suites. It grandfathers in existing files that were lacking the call entirely. This PR also includes manual fixes to files that used the function already but were missing it in one or more suites, which the lint rule detects.
38 lines
1.3 KiB
TypeScript
38 lines
1.3 KiB
TypeScript
/*---------------------------------------------------------------------------------------------
|
|
* Copyright (c) Microsoft Corporation. All rights reserved.
|
|
* Licensed under the MIT License. See License.txt in the project root for license information.
|
|
*--------------------------------------------------------------------------------------------*/
|
|
|
|
import * as eslint from 'eslint';
|
|
import { Node } from 'estree';
|
|
|
|
export = new class EnsureNoDisposablesAreLeakedInTestSuite implements eslint.Rule.RuleModule {
|
|
|
|
readonly meta: eslint.Rule.RuleMetaData = {
|
|
type: 'problem',
|
|
messages: {
|
|
ensure: 'Suites should include a call to `ensureNoDisposablesAreLeakedInTestSuite()` to ensure no disposables are leaked in tests.'
|
|
}
|
|
};
|
|
|
|
create(context: eslint.Rule.RuleContext): eslint.Rule.RuleListener {
|
|
const config = <{ exclude: string[] }>context.options[0];
|
|
|
|
const needle = context.getFilename().replace(/\\/g, '/');
|
|
if (config.exclude.some((e) => needle.endsWith(e))) {
|
|
return {};
|
|
}
|
|
|
|
return {
|
|
[`Program > ExpressionStatement > CallExpression[callee.name='suite']`]: (node: Node) => {
|
|
const src = context.getSourceCode().getText(node)
|
|
if (!src.includes('ensureNoDisposablesAreLeakedInTestSuite(')) {
|
|
context.report({
|
|
node,
|
|
messageId: 'ensure',
|
|
});
|
|
}
|
|
},
|
|
};
|
|
}
|
|
};
|