mirror of
https://github.com/XAMPPRocky/tokei
synced 2024-10-30 07:11:48 +00:00
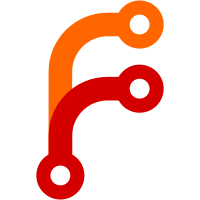
* Added CSharp example Using example from #330, for testing purposes * Updated Rust test case Rust test case now has a verbatim string that fails, with current version of Tokei. * Added C++ verbatim string to example C++ Syntax makes this incredibly difficult to parse using the current infrastructure, `R"PREFIX( )PREFIX"` where PREFIX can be almost anything. * Updated F# Test to have verbatim string * Add support for "verbatim_quotes" Quotes that neglect the `\"` rule, in the case of python as mentioned in #330 it produces the correct outcome with Tokei currently. * Add support for verbatim_quotes in syntax Properly keeps track of current state and handles escapes properly in the case of being inside of a verbatim_quote * Added `verbatim_quotes` to CONTRIBUTING.md
46 lines
711 B
C++
46 lines
711 B
C++
/* 46 lines 37 code 3 comments 6 blanks */
|
|
|
|
#include <stdio.h>
|
|
|
|
// bubble_sort_function
|
|
void bubble_sort(int a[10], int n) {
|
|
int t;
|
|
int j = n;
|
|
int s = 1;
|
|
while (s > 0) {
|
|
s = 0;
|
|
int i = 1;
|
|
while (i < j) {
|
|
if (a[i] < a[i - 1]) {
|
|
t = a[i];
|
|
a[i] = a[i - 1];
|
|
a[i - 1] = t;
|
|
s = 1;
|
|
}
|
|
i++;
|
|
}
|
|
j--;
|
|
}
|
|
}
|
|
|
|
int main() {
|
|
int a[] = {4, 65, 2, -31, 0, 99, 2, 83, 782, 1};
|
|
int n = 10;
|
|
int i = 0;
|
|
|
|
printf(R"(Before sorting:\n\n" )");
|
|
// Single line comment
|
|
while (i < n) {
|
|
printf("%d ", a[i]);
|
|
i++;
|
|
}
|
|
|
|
bubble_sort(a, n);
|
|
|
|
printf("\n\nAfter sorting:\n\n");
|
|
i = 0;
|
|
while (i < n) {
|
|
printf("%d ", a[i]);
|
|
i++;
|
|
}
|
|
}
|