mirror of
https://github.com/git/git
synced 2024-08-28 20:20:07 +00:00
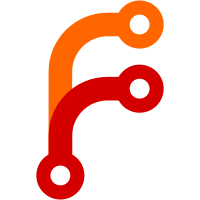
We don't actually need to know at the time of transport_get if the caller wants to fetch, push, or do both on the returned object. It is easier to just delay the initialization of the HTTP walker until we know we will need it by providing a CURL specific fetch function in the curl_transport that makes sure the walker instance is initialized before use. Signed-off-by: Shawn O. Pearce <spearce@spearce.org> Signed-off-by: Junio C Hamano <gitster@pobox.com>
79 lines
2.2 KiB
C
79 lines
2.2 KiB
C
#ifndef TRANSPORT_H
|
|
#define TRANSPORT_H
|
|
|
|
#include "cache.h"
|
|
#include "remote.h"
|
|
|
|
struct transport {
|
|
unsigned verbose : 1;
|
|
struct remote *remote;
|
|
const char *url;
|
|
|
|
void *data;
|
|
|
|
struct ref *remote_refs;
|
|
|
|
const struct transport_ops *ops;
|
|
char *pack_lockfile;
|
|
};
|
|
|
|
#define TRANSPORT_PUSH_ALL 1
|
|
#define TRANSPORT_PUSH_FORCE 2
|
|
|
|
struct transport_ops {
|
|
/**
|
|
* Returns 0 if successful, positive if the option is not
|
|
* recognized or is inapplicable, and negative if the option
|
|
* is applicable but the value is invalid.
|
|
**/
|
|
int (*set_option)(struct transport *connection, const char *name,
|
|
const char *value);
|
|
|
|
struct ref *(*get_refs_list)(const struct transport *transport);
|
|
int (*fetch)(struct transport *transport, int refs_nr, struct ref **refs);
|
|
int (*push)(struct transport *connection, int refspec_nr, const char **refspec, int flags);
|
|
|
|
int (*disconnect)(struct transport *connection);
|
|
};
|
|
|
|
/* Returns a transport suitable for the url */
|
|
struct transport *transport_get(struct remote *, const char *);
|
|
|
|
/* Transport options which apply to git:// and scp-style URLs */
|
|
|
|
/* The program to use on the remote side to send a pack */
|
|
#define TRANS_OPT_UPLOADPACK "uploadpack"
|
|
|
|
/* The program to use on the remote side to receive a pack */
|
|
#define TRANS_OPT_RECEIVEPACK "receivepack"
|
|
|
|
/* Transfer the data as a thin pack if not null */
|
|
#define TRANS_OPT_THIN "thin"
|
|
|
|
/* Keep the pack that was transferred if not null */
|
|
#define TRANS_OPT_KEEP "keep"
|
|
|
|
/* Unpack the objects if fewer than this number of objects are fetched */
|
|
#define TRANS_OPT_UNPACKLIMIT "unpacklimit"
|
|
|
|
/* Limit the depth of the fetch if not null */
|
|
#define TRANS_OPT_DEPTH "depth"
|
|
|
|
/**
|
|
* Returns 0 if the option was used, non-zero otherwise. Prints a
|
|
* message to stderr if the option is not used.
|
|
**/
|
|
int transport_set_option(struct transport *transport, const char *name,
|
|
const char *value);
|
|
|
|
int transport_push(struct transport *connection,
|
|
int refspec_nr, const char **refspec, int flags);
|
|
|
|
struct ref *transport_get_remote_refs(struct transport *transport);
|
|
|
|
int transport_fetch_refs(struct transport *transport, struct ref *refs);
|
|
void transport_unlock_pack(struct transport *transport);
|
|
int transport_disconnect(struct transport *transport);
|
|
|
|
#endif
|