mirror of
https://github.com/git/git
synced 2024-08-25 10:45:59 +00:00
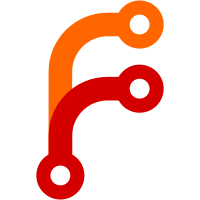
Currently, there is only one attempt to acquire any lockfile, and if the lock is held by another process, the locking attempt fails immediately. This is not such a limitation for loose reference files. First, they don't take long to rewrite. Second, most reference updates have a known "old" value, so if another process is updating a reference at the same moment that we are trying to lock it, then probably the expected "old" value will not longer be valid, and the update will fail anyway. But these arguments do not hold for packed-refs: * The packed-refs file can be large and take significant time to rewrite. * Many references are stored in a single packed-refs file, so it could be that the other process was changing a different reference than the one that we are interested in. Therefore, it is much more likely for there to be spurious lock conflicts in connection to the packed-refs file, resulting in unnecessary command failures. So, if the first attempt to lock the packed-refs file fails, continue retrying for a configurable length of time before giving up. The default timeout is 1 second. Signed-off-by: Michael Haggerty <mhagger@alum.mit.edu> Signed-off-by: Junio C Hamano <gitster@pobox.com>
208 lines
5.5 KiB
Bash
Executable file
208 lines
5.5 KiB
Bash
Executable file
#!/bin/sh
|
|
#
|
|
# Copyright (c) 2005 Amos Waterland
|
|
# Copyright (c) 2006 Christian Couder
|
|
#
|
|
|
|
test_description='git pack-refs should not change the branch semantic
|
|
|
|
This test runs git pack-refs and git show-ref and checks that the branch
|
|
semantic is still the same.
|
|
'
|
|
. ./test-lib.sh
|
|
|
|
test_expect_success 'enable reflogs' '
|
|
git config core.logallrefupdates true
|
|
'
|
|
|
|
test_expect_success \
|
|
'prepare a trivial repository' \
|
|
'echo Hello > A &&
|
|
git update-index --add A &&
|
|
git commit -m "Initial commit." &&
|
|
HEAD=$(git rev-parse --verify HEAD)'
|
|
|
|
SHA1=
|
|
|
|
test_expect_success \
|
|
'see if git show-ref works as expected' \
|
|
'git branch a &&
|
|
SHA1=`cat .git/refs/heads/a` &&
|
|
echo "$SHA1 refs/heads/a" >expect &&
|
|
git show-ref a >result &&
|
|
test_cmp expect result'
|
|
|
|
test_expect_success \
|
|
'see if a branch still exists when packed' \
|
|
'git branch b &&
|
|
git pack-refs --all &&
|
|
rm -f .git/refs/heads/b &&
|
|
echo "$SHA1 refs/heads/b" >expect &&
|
|
git show-ref b >result &&
|
|
test_cmp expect result'
|
|
|
|
test_expect_success 'git branch c/d should barf if branch c exists' '
|
|
git branch c &&
|
|
git pack-refs --all &&
|
|
rm -f .git/refs/heads/c &&
|
|
test_must_fail git branch c/d
|
|
'
|
|
|
|
test_expect_success \
|
|
'see if a branch still exists after git pack-refs --prune' \
|
|
'git branch e &&
|
|
git pack-refs --all --prune &&
|
|
echo "$SHA1 refs/heads/e" >expect &&
|
|
git show-ref e >result &&
|
|
test_cmp expect result'
|
|
|
|
test_expect_success 'see if git pack-refs --prune remove ref files' '
|
|
git branch f &&
|
|
git pack-refs --all --prune &&
|
|
! test -f .git/refs/heads/f
|
|
'
|
|
|
|
test_expect_success 'see if git pack-refs --prune removes empty dirs' '
|
|
git branch r/s/t &&
|
|
git pack-refs --all --prune &&
|
|
! test -e .git/refs/heads/r
|
|
'
|
|
|
|
test_expect_success \
|
|
'git branch g should work when git branch g/h has been deleted' \
|
|
'git branch g/h &&
|
|
git pack-refs --all --prune &&
|
|
git branch -d g/h &&
|
|
git branch g &&
|
|
git pack-refs --all &&
|
|
git branch -d g'
|
|
|
|
test_expect_success 'git branch i/j/k should barf if branch i exists' '
|
|
git branch i &&
|
|
git pack-refs --all --prune &&
|
|
test_must_fail git branch i/j/k
|
|
'
|
|
|
|
test_expect_success \
|
|
'test git branch k after branch k/l/m and k/lm have been deleted' \
|
|
'git branch k/l &&
|
|
git branch k/lm &&
|
|
git branch -d k/l &&
|
|
git branch k/l/m &&
|
|
git branch -d k/l/m &&
|
|
git branch -d k/lm &&
|
|
git branch k'
|
|
|
|
test_expect_success \
|
|
'test git branch n after some branch deletion and pruning' \
|
|
'git branch n/o &&
|
|
git branch n/op &&
|
|
git branch -d n/o &&
|
|
git branch n/o/p &&
|
|
git branch -d n/op &&
|
|
git pack-refs --all --prune &&
|
|
git branch -d n/o/p &&
|
|
git branch n'
|
|
|
|
test_expect_success \
|
|
'see if up-to-date packed refs are preserved' \
|
|
'git branch q &&
|
|
git pack-refs --all --prune &&
|
|
git update-ref refs/heads/q refs/heads/q &&
|
|
! test -f .git/refs/heads/q'
|
|
|
|
test_expect_success 'pack, prune and repack' '
|
|
git tag foo &&
|
|
git pack-refs --all --prune &&
|
|
git show-ref >all-of-them &&
|
|
git pack-refs &&
|
|
git show-ref >again &&
|
|
test_cmp all-of-them again
|
|
'
|
|
|
|
test_expect_success 'explicit pack-refs with dangling packed reference' '
|
|
git commit --allow-empty -m "soon to be garbage-collected" &&
|
|
git pack-refs --all &&
|
|
git reset --hard HEAD^ &&
|
|
git reflog expire --expire=all --all &&
|
|
git prune --expire=all &&
|
|
git pack-refs --all 2>result &&
|
|
test_cmp /dev/null result
|
|
'
|
|
|
|
test_expect_success 'delete ref with dangling packed version' '
|
|
git checkout -b lamb &&
|
|
git commit --allow-empty -m "future garbage" &&
|
|
git pack-refs --all &&
|
|
git reset --hard HEAD^ &&
|
|
git checkout master &&
|
|
git reflog expire --expire=all --all &&
|
|
git prune --expire=all &&
|
|
git branch -d lamb 2>result &&
|
|
test_cmp /dev/null result
|
|
'
|
|
|
|
test_expect_success 'delete ref while another dangling packed ref' '
|
|
git branch lamb &&
|
|
git commit --allow-empty -m "future garbage" &&
|
|
git pack-refs --all &&
|
|
git reset --hard HEAD^ &&
|
|
git reflog expire --expire=all --all &&
|
|
git prune --expire=all &&
|
|
git branch -d lamb 2>result &&
|
|
test_cmp /dev/null result
|
|
'
|
|
|
|
test_expect_success 'pack ref directly below refs/' '
|
|
git update-ref refs/top HEAD &&
|
|
git pack-refs --all --prune &&
|
|
grep refs/top .git/packed-refs &&
|
|
test_path_is_missing .git/refs/top
|
|
'
|
|
|
|
test_expect_success 'disable reflogs' '
|
|
git config core.logallrefupdates false &&
|
|
rm -rf .git/logs
|
|
'
|
|
|
|
test_expect_success 'create packed foo/bar/baz branch' '
|
|
git branch foo/bar/baz &&
|
|
git pack-refs --all --prune &&
|
|
test_path_is_missing .git/refs/heads/foo/bar/baz &&
|
|
test_path_is_missing .git/logs/refs/heads/foo/bar/baz
|
|
'
|
|
|
|
test_expect_success 'notice d/f conflict with existing directory' '
|
|
test_must_fail git branch foo &&
|
|
test_must_fail git branch foo/bar
|
|
'
|
|
|
|
test_expect_success 'existing directory reports concrete ref' '
|
|
test_must_fail git branch foo 2>stderr &&
|
|
grep refs/heads/foo/bar/baz stderr
|
|
'
|
|
|
|
test_expect_success 'notice d/f conflict with existing ref' '
|
|
test_must_fail git branch foo/bar/baz/extra &&
|
|
test_must_fail git branch foo/bar/baz/lots/of/extra/components
|
|
'
|
|
|
|
test_expect_success 'timeout if packed-refs.lock exists' '
|
|
LOCK=.git/packed-refs.lock &&
|
|
>"$LOCK" &&
|
|
test_when_finished "rm -f $LOCK" &&
|
|
test_must_fail git pack-refs --all --prune
|
|
'
|
|
|
|
test_expect_success 'retry acquiring packed-refs.lock' '
|
|
LOCK=.git/packed-refs.lock &&
|
|
>"$LOCK" &&
|
|
test_when_finished "wait; rm -f $LOCK" &&
|
|
{
|
|
( sleep 1 ; rm -f $LOCK ) &
|
|
} &&
|
|
git -c core.packedrefstimeout=3000 pack-refs --all --prune
|
|
'
|
|
|
|
test_done
|