mirror of
https://github.com/git/git
synced 2024-08-24 02:11:08 +00:00
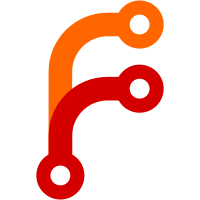
Replace the call to `FSEventStreamScheduleWithRunLoop()` function with the suggested `FSEventStreamSetDispatchQueue()` function. The MacOS version of the builtin FSMonitor feature uses the `FSEventStreamScheduleWithRunLoop()` function to drive the event loop and process FSEvents from the system. This routine has now been deprecated by Apple. The MacOS 13 (Ventura) compiler tool chain now generates a warning when compiling calls to this function. In DEVELOPER=1 mode, this now causes a compile error. The `FSEventStreamSetDispatchQueue()` function is conceptually similar and is the suggested replacement. However, there are some subtle thread-related differences. Previously, the event stream would be processed by the `fsm_listen__loop()` thread while it was in the `CFRunLoopRun()` method. (Conceptually, this was a blocking call on the lifetime of the event stream where our thread drove the event loop and individual events were handled by the `fsevent_callback()`.) With the change, a "dispatch queue" is created and FSEvents will be processed by a hidden queue-related thread (that calls the `fsevent_callback()` on our behalf). Our `fsm_listen__loop()` thread maintains the original blocking model by waiting on a mutex/condition variable pair while the hidden thread does all of the work. While the deprecated API used by the original were introduced in macOS 10.5 (Oct 2007), the API used by the updated code were introduced back in macOS 10.6 (Aug 2009) and has been available since then. So this change _could_ break those who have happily been using 10.5 (if there were such people), but these two dates both predate the oldest versions of macOS Apple seems to support anyway, so we should be safe. Signed-off-by: Jeff Hostetler <jeffhostetler@github.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
91 lines
3.8 KiB
C
91 lines
3.8 KiB
C
#ifndef FSM_DARWIN_GCC_H
|
|
#define FSM_DARWIN_GCC_H
|
|
|
|
#ifndef __clang__
|
|
/*
|
|
* It is possible to #include CoreFoundation/CoreFoundation.h when compiling
|
|
* with clang, but not with GCC as of time of writing.
|
|
*
|
|
* See https://gcc.gnu.org/bugzilla/show_bug.cgi?id=93082 for details.
|
|
*/
|
|
typedef unsigned int FSEventStreamCreateFlags;
|
|
#define kFSEventStreamEventFlagNone 0x00000000
|
|
#define kFSEventStreamEventFlagMustScanSubDirs 0x00000001
|
|
#define kFSEventStreamEventFlagUserDropped 0x00000002
|
|
#define kFSEventStreamEventFlagKernelDropped 0x00000004
|
|
#define kFSEventStreamEventFlagEventIdsWrapped 0x00000008
|
|
#define kFSEventStreamEventFlagHistoryDone 0x00000010
|
|
#define kFSEventStreamEventFlagRootChanged 0x00000020
|
|
#define kFSEventStreamEventFlagMount 0x00000040
|
|
#define kFSEventStreamEventFlagUnmount 0x00000080
|
|
#define kFSEventStreamEventFlagItemCreated 0x00000100
|
|
#define kFSEventStreamEventFlagItemRemoved 0x00000200
|
|
#define kFSEventStreamEventFlagItemInodeMetaMod 0x00000400
|
|
#define kFSEventStreamEventFlagItemRenamed 0x00000800
|
|
#define kFSEventStreamEventFlagItemModified 0x00001000
|
|
#define kFSEventStreamEventFlagItemFinderInfoMod 0x00002000
|
|
#define kFSEventStreamEventFlagItemChangeOwner 0x00004000
|
|
#define kFSEventStreamEventFlagItemXattrMod 0x00008000
|
|
#define kFSEventStreamEventFlagItemIsFile 0x00010000
|
|
#define kFSEventStreamEventFlagItemIsDir 0x00020000
|
|
#define kFSEventStreamEventFlagItemIsSymlink 0x00040000
|
|
#define kFSEventStreamEventFlagOwnEvent 0x00080000
|
|
#define kFSEventStreamEventFlagItemIsHardlink 0x00100000
|
|
#define kFSEventStreamEventFlagItemIsLastHardlink 0x00200000
|
|
#define kFSEventStreamEventFlagItemCloned 0x00400000
|
|
|
|
typedef struct __FSEventStream *FSEventStreamRef;
|
|
typedef const FSEventStreamRef ConstFSEventStreamRef;
|
|
|
|
typedef unsigned int CFStringEncoding;
|
|
#define kCFStringEncodingUTF8 0x08000100
|
|
|
|
typedef const struct __CFString *CFStringRef;
|
|
typedef const struct __CFArray *CFArrayRef;
|
|
typedef const struct __CFRunLoop *CFRunLoopRef;
|
|
|
|
struct FSEventStreamContext {
|
|
long long version;
|
|
void *cb_data, *retain, *release, *copy_description;
|
|
};
|
|
|
|
typedef struct FSEventStreamContext FSEventStreamContext;
|
|
typedef unsigned int FSEventStreamEventFlags;
|
|
#define kFSEventStreamCreateFlagNoDefer 0x02
|
|
#define kFSEventStreamCreateFlagWatchRoot 0x04
|
|
#define kFSEventStreamCreateFlagFileEvents 0x10
|
|
|
|
typedef unsigned long long FSEventStreamEventId;
|
|
#define kFSEventStreamEventIdSinceNow 0xFFFFFFFFFFFFFFFFULL
|
|
|
|
typedef void (*FSEventStreamCallback)(ConstFSEventStreamRef streamRef,
|
|
void *context,
|
|
__SIZE_TYPE__ num_of_events,
|
|
void *event_paths,
|
|
const FSEventStreamEventFlags event_flags[],
|
|
const FSEventStreamEventId event_ids[]);
|
|
typedef double CFTimeInterval;
|
|
FSEventStreamRef FSEventStreamCreate(void *allocator,
|
|
FSEventStreamCallback callback,
|
|
FSEventStreamContext *context,
|
|
CFArrayRef paths_to_watch,
|
|
FSEventStreamEventId since_when,
|
|
CFTimeInterval latency,
|
|
FSEventStreamCreateFlags flags);
|
|
CFStringRef CFStringCreateWithCString(void *allocator, const char *string,
|
|
CFStringEncoding encoding);
|
|
CFArrayRef CFArrayCreate(void *allocator, const void **items, long long count,
|
|
void *callbacks);
|
|
void CFRunLoopRun(void);
|
|
void CFRunLoopStop(CFRunLoopRef run_loop);
|
|
CFRunLoopRef CFRunLoopGetCurrent(void);
|
|
extern CFStringRef kCFRunLoopDefaultMode;
|
|
void FSEventStreamSetDispatchQueue(FSEventStreamRef stream, dispatch_queue_t q);
|
|
unsigned char FSEventStreamStart(FSEventStreamRef stream);
|
|
void FSEventStreamStop(FSEventStreamRef stream);
|
|
void FSEventStreamInvalidate(FSEventStreamRef stream);
|
|
void FSEventStreamRelease(FSEventStreamRef stream);
|
|
|
|
#endif /* !clang */
|
|
#endif /* FSM_DARWIN_GCC_H */
|