mirror of
https://github.com/git/git
synced 2024-09-19 16:31:33 +00:00
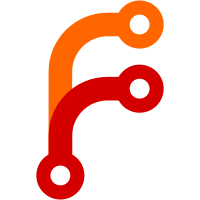
This command allows to delete a worktree. Like 'move' you cannot remove the main worktree, or one with submodules inside [1]. For deleting $GIT_WORK_TREE, Untracked files or any staged entries are considered precious and therefore prevent removal by default. Ignored files are not precious. When it comes to deleting $GIT_DIR, there's no "clean" check because there should not be any valuable data in there, except: - HEAD reflog. There is nothing we can do about this until somebody steps up and implements the ref graveyard. - Detached HEAD. Technically it can still be recovered. Although it may be nice to warn about orphan commits like 'git checkout' does. [1] We do 'git status' with --ignore-submodules=all for safety anyway. But this needs a closer look by submodule people before we can allow deletion. For example, if a submodule is totally clean, but its repo not absorbed to the main .git dir, then deleting worktree also deletes the valuable .submodule repo too. Signed-off-by: Nguyễn Thái Ngọc Duy <pclouds@gmail.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
130 lines
3.5 KiB
Bash
Executable file
130 lines
3.5 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
test_description='test git worktree move, remove, lock and unlock'
|
|
|
|
. ./test-lib.sh
|
|
|
|
test_expect_success 'setup' '
|
|
test_commit init &&
|
|
git worktree add source &&
|
|
git worktree list --porcelain | grep "^worktree" >actual &&
|
|
cat <<-EOF >expected &&
|
|
worktree $(pwd)
|
|
worktree $(pwd)/source
|
|
EOF
|
|
test_cmp expected actual
|
|
'
|
|
|
|
test_expect_success 'lock main worktree' '
|
|
test_must_fail git worktree lock .
|
|
'
|
|
|
|
test_expect_success 'lock linked worktree' '
|
|
git worktree lock --reason hahaha source &&
|
|
echo hahaha >expected &&
|
|
test_cmp expected .git/worktrees/source/locked
|
|
'
|
|
|
|
test_expect_success 'lock linked worktree from another worktree' '
|
|
rm .git/worktrees/source/locked &&
|
|
git worktree add elsewhere &&
|
|
git -C elsewhere worktree lock --reason hahaha ../source &&
|
|
echo hahaha >expected &&
|
|
test_cmp expected .git/worktrees/source/locked
|
|
'
|
|
|
|
test_expect_success 'lock worktree twice' '
|
|
test_must_fail git worktree lock source &&
|
|
echo hahaha >expected &&
|
|
test_cmp expected .git/worktrees/source/locked
|
|
'
|
|
|
|
test_expect_success 'lock worktree twice (from the locked worktree)' '
|
|
test_must_fail git -C source worktree lock . &&
|
|
echo hahaha >expected &&
|
|
test_cmp expected .git/worktrees/source/locked
|
|
'
|
|
|
|
test_expect_success 'unlock main worktree' '
|
|
test_must_fail git worktree unlock .
|
|
'
|
|
|
|
test_expect_success 'unlock linked worktree' '
|
|
git worktree unlock source &&
|
|
test_path_is_missing .git/worktrees/source/locked
|
|
'
|
|
|
|
test_expect_success 'unlock worktree twice' '
|
|
test_must_fail git worktree unlock source &&
|
|
test_path_is_missing .git/worktrees/source/locked
|
|
'
|
|
|
|
test_expect_success 'move non-worktree' '
|
|
mkdir abc &&
|
|
test_must_fail git worktree move abc def
|
|
'
|
|
|
|
test_expect_success 'move locked worktree' '
|
|
git worktree lock source &&
|
|
test_when_finished "git worktree unlock source" &&
|
|
test_must_fail git worktree move source destination
|
|
'
|
|
|
|
test_expect_success 'move worktree' '
|
|
toplevel="$(pwd)" &&
|
|
git worktree move source destination &&
|
|
test_path_is_missing source &&
|
|
git worktree list --porcelain | grep "^worktree.*/destination" &&
|
|
! git worktree list --porcelain | grep "^worktree.*/source" >empty &&
|
|
git -C destination log --format=%s >actual2 &&
|
|
echo init >expected2 &&
|
|
test_cmp expected2 actual2
|
|
'
|
|
|
|
test_expect_success 'move main worktree' '
|
|
test_must_fail git worktree move . def
|
|
'
|
|
|
|
test_expect_success 'move worktree to another dir' '
|
|
toplevel="$(pwd)" &&
|
|
mkdir some-dir &&
|
|
git worktree move destination some-dir &&
|
|
test_path_is_missing source &&
|
|
git worktree list --porcelain | grep "^worktree.*/some-dir/destination" &&
|
|
git -C some-dir/destination log --format=%s >actual2 &&
|
|
echo init >expected2 &&
|
|
test_cmp expected2 actual2
|
|
'
|
|
|
|
test_expect_success 'remove main worktree' '
|
|
test_must_fail git worktree remove .
|
|
'
|
|
|
|
test_expect_success 'move some-dir/destination back' '
|
|
git worktree move some-dir/destination destination
|
|
'
|
|
|
|
test_expect_success 'remove locked worktree' '
|
|
git worktree lock destination &&
|
|
test_when_finished "git worktree unlock destination" &&
|
|
test_must_fail git worktree remove destination
|
|
'
|
|
|
|
test_expect_success 'remove worktree with dirty tracked file' '
|
|
echo dirty >>destination/init.t &&
|
|
test_when_finished "git -C destination checkout init.t" &&
|
|
test_must_fail git worktree remove destination
|
|
'
|
|
|
|
test_expect_success 'remove worktree with untracked file' '
|
|
: >destination/untracked &&
|
|
test_must_fail git worktree remove destination
|
|
'
|
|
|
|
test_expect_success 'force remove worktree with untracked file' '
|
|
git worktree remove --force destination &&
|
|
test_path_is_missing destination
|
|
'
|
|
|
|
test_done
|