mirror of
https://github.com/git/git
synced 2024-08-25 10:45:59 +00:00
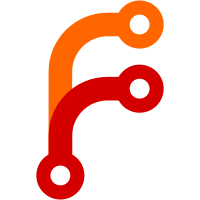
Originally, the filter_spec field was just a string pointer. In
cf9ceb5a12
(list-objects-filter-options: make filter_spec a string_list,
2019-06-27) it became a string_list, but that commit notes:
A strbuf would seem to be a more natural choice for this object, but
it unfortunately requires initialization besides just zero'ing out
the memory. This results in all container structs, and all
containers of those structs, etc., to also require initialization.
Initializing them all would be more cumbersome that simply using a
string_list, which behaves properly when its contents are zero'd.
Now that we've changed the struct to require non-zero initialization
anyway (ironically, because string_list also needed non-zero
initialization to avoid leaks), we can now convert to that more natural
type.
This makes the list_objects_filter_spec() function much less awkward, as
it had to collapse the string_list to a single-entry list on the fly.
Signed-off-by: Jeff King <peff@peff.net>
Signed-off-by: Junio C Hamano <gitster@pobox.com>
178 lines
5.7 KiB
C
178 lines
5.7 KiB
C
#ifndef LIST_OBJECTS_FILTER_OPTIONS_H
|
|
#define LIST_OBJECTS_FILTER_OPTIONS_H
|
|
|
|
#include "cache.h"
|
|
#include "parse-options.h"
|
|
#include "string-list.h"
|
|
|
|
/*
|
|
* The list of defined filters for list-objects.
|
|
*/
|
|
enum list_objects_filter_choice {
|
|
LOFC_DISABLED = 0,
|
|
LOFC_BLOB_NONE,
|
|
LOFC_BLOB_LIMIT,
|
|
LOFC_TREE_DEPTH,
|
|
LOFC_SPARSE_OID,
|
|
LOFC_OBJECT_TYPE,
|
|
LOFC_COMBINE,
|
|
LOFC__COUNT /* must be last */
|
|
};
|
|
|
|
/*
|
|
* Returns a configuration key suitable for describing the given object filter,
|
|
* e.g.: "blob:none", "combine", etc.
|
|
*/
|
|
const char *list_object_filter_config_name(enum list_objects_filter_choice c);
|
|
|
|
struct list_objects_filter_options {
|
|
/*
|
|
* 'filter_spec' is the raw argument value given on the command line
|
|
* or protocol request. (The part after the "--keyword=".) For
|
|
* commands that launch filtering sub-processes, or for communication
|
|
* over the network, don't use this value; use the result of
|
|
* expand_list_objects_filter_spec() instead.
|
|
* To get the raw filter spec given by the user, use the result of
|
|
* list_objects_filter_spec().
|
|
*/
|
|
struct strbuf filter_spec;
|
|
|
|
/*
|
|
* 'choice' is determined by parsing the filter-spec. This indicates
|
|
* the filtering algorithm to use.
|
|
*/
|
|
enum list_objects_filter_choice choice;
|
|
|
|
/*
|
|
* Choice is LOFC_DISABLED because "--no-filter" was requested.
|
|
|