mirror of
https://github.com/git/git
synced 2024-10-28 19:25:47 +00:00
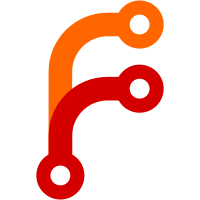
The tests for the merge machinery are spread over several places.
Collect them into t64xx for simplicity. Some notes:
t60[234]*.sh:
Merge tests started in t602*, overgrew bisect and remote tracking
tests in t6030, t6040, and t6041, and nearly overtook replace tests
in t6050. This made picking out relevant tests that I wanted to run
in a tighter loop slightly more annoying for years.
t303*.sh:
These started out as tests for the 'merge-recursive' toplevel command,
but did not restrict to that and had lots of overlap with the
underlying merge machinery.
t7405, t7613:
submodule-specific merge logic started out in submodule.c but was
moved to merge-recursive.c in commit 18cfc08866
("submodule.c: move
submodule merging to merge-recursive.c", 2018-05-15). Since these
tests are about the logic found in the merge machinery, moving these
tests to be with the merge tests makes sense.
t7607, t7609:
Having tests spread all over the place makes it more likely that
additional tests related to a certain piece of logic grow in all those
other places. Much like t303*.sh, these two tests were about the
underlying merge machinery rather than outer levels.
Tests that were NOT moved:
t76[01]*.sh:
Other than the four tests mentioned above, the remaining tests in
t76[01]*.sh are related to non-recursive merge strategies, parameter
parsing, and other stuff associated with the highlevel builtin/merge.c
rather than the recursive merge machinery.
t3[45]*.sh:
The rebase testcases in t34*.sh also test the merge logic pretty
heavily; sometimes changes I make only trigger failures in the rebase
tests. The rebase tests are already nicely coupled together, though,
and I didn't want to mess that up. Similar comments apply for the
cherry-pick tests in t35*.sh.
Signed-off-by: Elijah Newren <newren@gmail.com>
Signed-off-by: Junio C Hamano <gitster@pobox.com>
216 lines
4.5 KiB
Bash
Executable file
216 lines
4.5 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
test_description="merges with unrelated index changes"
|
|
|
|
. ./test-lib.sh
|
|
|
|
# Testcase for some simple merges
|
|
# A
|
|
# o-------o B
|
|
# \
|
|
# \-----o C
|
|
# \
|
|
# \---o D
|
|
# \
|
|
# \-o E
|
|
# \
|
|
# o F
|
|
# Commit A: some file a
|
|
# Commit B: adds file b, modifies end of a
|
|
# Commit C: adds file c
|
|
# Commit D: adds file d, modifies beginning of a
|
|
# Commit E: renames a->subdir/a, adds subdir/e
|
|
# Commit F: empty commit
|
|
|
|
test_expect_success 'setup trivial merges' '
|
|
test_seq 1 10 >a &&
|
|
git add a &&
|
|
test_tick && git commit -m A &&
|
|
|
|
git branch A &&
|
|
git branch B &&
|
|
git branch C &&
|
|
git branch D &&
|
|
git branch E &&
|
|
git branch F &&
|
|
|
|
git checkout B &&
|
|
echo b >b &&
|
|
echo 11 >>a &&
|
|
git add a b &&
|
|
test_tick && git commit -m B &&
|
|
|
|
git checkout C &&
|
|
echo c >c &&
|
|
git add c &&
|
|
test_tick && git commit -m C &&
|
|
|
|
git checkout D &&
|
|
test_seq 2 10 >a &&
|
|
echo d >d &&
|
|
git add a d &&
|
|
test_tick && git commit -m D &&
|
|
|
|
git checkout E &&
|
|
mkdir subdir &&
|
|
git mv a subdir/a &&
|
|
echo e >subdir/e &&
|
|
git add subdir &&
|
|
test_tick && git commit -m E &&
|
|
|
|
git checkout F &&
|
|
test_tick && git commit --allow-empty -m F
|
|
'
|
|
|
|
test_expect_success 'ff update' '
|
|
git reset --hard &&
|
|
git checkout A^0 &&
|
|
|
|
touch random_file && git add random_file &&
|
|
|
|
git merge E^0 &&
|
|
|
|
test_must_fail git rev-parse HEAD:random_file &&
|
|
test "$(git diff --name-only --cached E)" = "random_file"
|
|
'
|
|
|
|
test_expect_success 'ff update, important file modified' '
|
|
git reset --hard &&
|
|
git checkout A^0 &&
|
|
|
|
mkdir subdir &&
|
|
touch subdir/e &&
|
|
git add subdir/e &&
|
|
|
|
test_must_fail git merge E^0 &&
|
|
test_path_is_missing .git/MERGE_HEAD
|
|
'
|
|
|
|
test_expect_success 'resolve, trivial' '
|
|
git reset --hard &&
|
|
git checkout B^0 &&
|
|
|
|
touch random_file && git add random_file &&
|
|
|
|
test_must_fail git merge -s resolve C^0 &&
|
|
test_path_is_missing .git/MERGE_HEAD
|
|
'
|
|
|
|
test_expect_success 'resolve, non-trivial' '
|
|
git reset --hard &&
|
|
git checkout B^0 &&
|
|
|
|
touch random_file && git add random_file &&
|
|
|
|
test_must_fail git merge -s resolve D^0 &&
|
|
test_path_is_missing .git/MERGE_HEAD
|
|
'
|
|
|
|
test_expect_success 'recursive' '
|
|
git reset --hard &&
|
|
git checkout B^0 &&
|
|
|
|
touch random_file && git add random_file &&
|
|
|
|
test_must_fail git merge -s recursive C^0 &&
|
|
test_path_is_missing .git/MERGE_HEAD
|
|
'
|
|
|
|
test_expect_success 'recursive, when merge branch matches merge base' '
|
|
git reset --hard &&
|
|
git checkout B^0 &&
|
|
|
|
touch random_file && git add random_file &&
|
|
|
|
test_must_fail git merge -s recursive F^0 &&
|
|
test_path_is_missing .git/MERGE_HEAD
|
|
'
|
|
|
|
test_expect_success 'merge-recursive, when index==head but head!=HEAD' '
|
|
git reset --hard &&
|
|
git checkout C^0 &&
|
|
|
|
# Make index match B
|
|
git diff C B -- | git apply --cached &&
|
|
# Merge B & F, with B as "head"
|
|
git merge-recursive A -- B F > out &&
|
|
test_i18ngrep "Already up to date" out
|
|
'
|
|
|
|
test_expect_success 'recursive, when file has staged changes not matching HEAD nor what a merge would give' '
|
|
git reset --hard &&
|
|
git checkout B^0 &&
|
|
|
|
mkdir subdir &&
|
|
test_seq 1 10 >subdir/a &&
|
|
git add subdir/a &&
|
|
|
|
# We have staged changes; merge should error out
|
|
test_must_fail git merge -s recursive E^0 2>err &&
|
|
test_i18ngrep "changes to the following files would be overwritten" err
|
|
'
|
|
|
|
test_expect_success 'recursive, when file has staged changes matching what a merge would give' '
|
|
git reset --hard &&
|
|
git checkout B^0 &&
|
|
|
|
mkdir subdir &&
|
|
test_seq 1 11 >subdir/a &&
|
|
git add subdir/a &&
|
|
|
|
# We have staged changes; merge should error out
|
|
test_must_fail git merge -s recursive E^0 2>err &&
|
|
test_i18ngrep "changes to the following files would be overwritten" err
|
|
'
|
|
|
|
test_expect_success 'octopus, unrelated file touched' '
|
|
git reset --hard &&
|
|
git checkout B^0 &&
|
|
|
|
touch random_file && git add random_file &&
|
|
|
|
test_must_fail git merge C^0 D^0 &&
|
|
test_path_is_missing .git/MERGE_HEAD
|
|
'
|
|
|
|
test_expect_success 'octopus, related file removed' '
|
|
git reset --hard &&
|
|
git checkout B^0 &&
|
|
|
|
git rm b &&
|
|
|
|
test_must_fail git merge C^0 D^0 &&
|
|
test_path_is_missing .git/MERGE_HEAD
|
|
'
|
|
|
|
test_expect_success 'octopus, related file modified' '
|
|
git reset --hard &&
|
|
git checkout B^0 &&
|
|
|
|
echo 12 >>a && git add a &&
|
|
|
|
test_must_fail git merge C^0 D^0 &&
|
|
test_path_is_missing .git/MERGE_HEAD
|
|
'
|
|
|
|
test_expect_success 'ours' '
|
|
git reset --hard &&
|
|
git checkout B^0 &&
|
|
|
|
touch random_file && git add random_file &&
|
|
|
|
test_must_fail git merge -s ours C^0 &&
|
|
test_path_is_missing .git/MERGE_HEAD
|
|
'
|
|
|
|
test_expect_success 'subtree' '
|
|
git reset --hard &&
|
|
git checkout B^0 &&
|
|
|
|
touch random_file && git add random_file &&
|
|
|
|
test_must_fail git merge -s subtree E^0 &&
|
|
test_path_is_missing .git/MERGE_HEAD
|
|
'
|
|
|
|
test_done
|