mirror of
https://github.com/git/git
synced 2024-10-28 19:25:47 +00:00
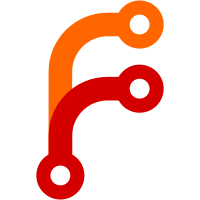
git_config() only had a function parameter, but no callback data parameter. This assumes that all callback functions only modify global variables. With this patch, every callback gets a void * parameter, and it is hoped that this will help the libification effort. Signed-off-by: Johannes Schindelin <johannes.schindelin@gmx.de> Signed-off-by: Junio C Hamano <gitster@pobox.com>
53 lines
1.2 KiB
C
53 lines
1.2 KiB
C
#include "builtin.h"
|
|
#include "cache.h"
|
|
#include "refs.h"
|
|
#include "parse-options.h"
|
|
|
|
static const char * const git_symbolic_ref_usage[] = {
|
|
"git-symbolic-ref [options] name [ref]",
|
|
NULL
|
|
};
|
|
|
|
static void check_symref(const char *HEAD, int quiet)
|
|
{
|
|
unsigned char sha1[20];
|
|
int flag;
|
|
const char *refs_heads_master = resolve_ref(HEAD, sha1, 0, &flag);
|
|
|
|
if (!refs_heads_master)
|
|
die("No such ref: %s", HEAD);
|
|
else if (!(flag & REF_ISSYMREF)) {
|
|
if (!quiet)
|
|
die("ref %s is not a symbolic ref", HEAD);
|
|
else
|
|
exit(1);
|
|
}
|
|
puts(refs_heads_master);
|
|
}
|
|
|
|
int cmd_symbolic_ref(int argc, const char **argv, const char *prefix)
|
|
{
|
|
int quiet = 0;
|
|
const char *msg = NULL;
|
|
struct option options[] = {
|
|
OPT__QUIET(&quiet),
|
|
OPT_STRING('m', NULL, &msg, "reason", "reason of the update"),
|
|
OPT_END(),
|
|
};
|
|
|
|
git_config(git_default_config, NULL);
|
|
argc = parse_options(argc, argv, options, git_symbolic_ref_usage, 0);
|
|
if (msg &&!*msg)
|
|
die("Refusing to perform update with empty message");
|
|
switch (argc) {
|
|
case 1:
|
|
check_symref(argv[0], quiet);
|
|
break;
|
|
case 2:
|
|
create_symref(argv[0], argv[1], msg);
|
|
break;
|
|
default:
|
|
usage_with_options(git_symbolic_ref_usage, options);
|
|
}
|
|
return 0;
|
|
}
|