mirror of
https://github.com/git/git
synced 2024-08-24 18:26:02 +00:00
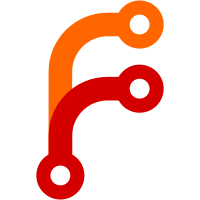
Commit aedcb7d
(branch.c: use 'ref-filter' APIs, 2015-09-23)
adjusted the symref-printing code to look like this:
if (item->symref) {
skip_prefix(item->symref, "refs/remotes/", &desc);
strbuf_addf(&out, " -> %s", desc);
}
This has three bugs in it:
1. It always skips past "refs/remotes/", instead of
skipping past the prefix associated with the branch we
are showing (so commonly we see "refs/remotes/" for the
refs/remotes/origin/HEAD symref, but the previous code
would skip "refs/heads/" when showing a symref it found
in refs/heads/.
2. If skip_prefix() does not match, it leaves "desc"
untouched, and we show whatever happened to be in it
(which is the refname from a call to skip_prefix()
earlier in the function).
3. If we do match with skip_prefix(), we stomp on the
"desc" variable, which is later passed to
add_verbose_info(). We probably want to retain the
original refname there (though it likely doesn't matter
in practice, since after all, one points to the other).
The fix to match the original code is fairly easy: record
the prefix to strip based on item->kind, and use it here.
However, since we already have a local variable named "prefix",
let's give the two prefixes verbose names so we don't
confuse them.
Signed-off-by: Jeff King <peff@peff.net>
Acked-by: Karthik Nayak <karthik.188@gmail.com>
Signed-off-by: Junio C Hamano <gitster@pobox.com>
200 lines
4.3 KiB
Bash
Executable file
200 lines
4.3 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
test_description='git branch display tests'
|
|
. ./test-lib.sh
|
|
|
|
test_expect_success 'make commits' '
|
|
echo content >file &&
|
|
git add file &&
|
|
git commit -m one &&
|
|
echo content >>file &&
|
|
git commit -a -m two
|
|
'
|
|
|
|
test_expect_success 'make branches' '
|
|
git branch branch-one &&
|
|
git branch branch-two HEAD^
|
|
'
|
|
|
|
test_expect_success 'make remote branches' '
|
|
git update-ref refs/remotes/origin/branch-one branch-one &&
|
|
git update-ref refs/remotes/origin/branch-two branch-two &&
|
|
git symbolic-ref refs/remotes/origin/HEAD refs/remotes/origin/branch-one
|
|
'
|
|
|
|
cat >expect <<'EOF'
|
|
branch-one
|
|
branch-two
|
|
* master
|
|
EOF
|
|
test_expect_success 'git branch shows local branches' '
|
|
git branch >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success 'git branch --list shows local branches' '
|
|
git branch --list >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
cat >expect <<'EOF'
|
|
branch-one
|
|
branch-two
|
|
EOF
|
|
test_expect_success 'git branch --list pattern shows matching local branches' '
|
|
git branch --list branch* >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
cat >expect <<'EOF'
|
|
origin/HEAD -> origin/branch-one
|
|
origin/branch-one
|
|
origin/branch-two
|
|
EOF
|
|
test_expect_success 'git branch -r shows remote branches' '
|
|
git branch -r >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
cat >expect <<'EOF'
|
|
branch-one
|
|
branch-two
|
|
* master
|
|
remotes/origin/HEAD -> origin/branch-one
|
|
remotes/origin/branch-one
|
|
remotes/origin/branch-two
|
|
EOF
|
|
test_expect_success 'git branch -a shows local and remote branches' '
|
|
git branch -a >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
cat >expect <<'EOF'
|
|
two
|
|
one
|
|
two
|
|
EOF
|
|
test_expect_success 'git branch -v shows branch summaries' '
|
|
git branch -v >tmp &&
|
|
awk "{print \$NF}" <tmp >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
cat >expect <<'EOF'
|
|
two
|
|
one
|
|
EOF
|
|
test_expect_success 'git branch --list -v pattern shows branch summaries' '
|
|
git branch --list -v branch* >tmp &&
|
|
awk "{print \$NF}" <tmp >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success 'git branch -v pattern does not show branch summaries' '
|
|
test_must_fail git branch -v branch*
|
|
'
|
|
|
|
test_expect_success 'git branch shows detached HEAD properly' '
|
|
cat >expect <<EOF &&
|
|
* (HEAD detached at $(git rev-parse --short HEAD^0))
|
|
branch-one
|
|
branch-two
|
|
master
|
|
EOF
|
|
git checkout HEAD^0 &&
|
|
git branch >actual &&
|
|
test_i18ncmp expect actual
|
|
'
|
|
|
|
test_expect_success 'git branch shows detached HEAD properly after checkout --detach' '
|
|
git checkout master &&
|
|
cat >expect <<EOF &&
|
|
* (HEAD detached at $(git rev-parse --short HEAD^0))
|
|
branch-one
|
|
branch-two
|
|
master
|
|
EOF
|
|
git checkout --detach &&
|
|
git branch >actual &&
|
|
test_i18ncmp expect actual
|
|
'
|
|
|
|
test_expect_success 'git branch shows detached HEAD properly after moving' '
|
|
cat >expect <<EOF &&
|
|
* (HEAD detached from $(git rev-parse --short HEAD))
|
|
branch-one
|
|
branch-two
|
|
master
|
|
EOF
|
|
git reset --hard HEAD^1 &&
|
|
git branch >actual &&
|
|
test_i18ncmp expect actual
|
|
'
|
|
|
|
test_expect_success 'git branch shows detached HEAD properly from tag' '
|
|
cat >expect <<EOF &&
|
|
* (HEAD detached at fromtag)
|
|
branch-one
|
|
branch-two
|
|
master
|
|
EOF
|
|
git tag fromtag master &&
|
|
git checkout fromtag &&
|
|
git branch >actual &&
|
|
test_i18ncmp expect actual
|
|
'
|
|
|
|
test_expect_success 'git branch shows detached HEAD properly after moving from tag' '
|
|
cat >expect <<EOF &&
|
|
* (HEAD detached from fromtag)
|
|
branch-one
|
|
branch-two
|
|
master
|
|
EOF
|
|
git reset --hard HEAD^1 &&
|
|
git branch >actual &&
|
|
test_i18ncmp expect actual
|
|
'
|
|
|
|
test_expect_success 'git branch `--sort` option' '
|
|
cat >expect <<-\EOF &&
|
|
* (HEAD detached from fromtag)
|
|
branch-two
|
|
branch-one
|
|
master
|
|
EOF
|
|
git branch --sort=objectsize >actual &&
|
|
test_i18ncmp expect actual
|
|
'
|
|
|
|
test_expect_success 'git branch --points-at option' '
|
|
cat >expect <<-\EOF &&
|
|
branch-one
|
|
master
|
|
EOF
|
|
git branch --points-at=branch-one >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success 'ambiguous branch/tag not marked' '
|
|
git tag ambiguous &&
|
|
git branch ambiguous &&
|
|
echo " ambiguous" >expect &&
|
|
git branch --list ambiguous >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success 'local-branch symrefs shortened properly' '
|
|
git symbolic-ref refs/heads/ref-to-branch refs/heads/branch-one &&
|
|
git symbolic-ref refs/heads/ref-to-remote refs/remotes/origin/branch-one &&
|
|
cat >expect <<-\EOF &&
|
|
ref-to-branch -> branch-one
|
|
ref-to-remote -> refs/remotes/origin/branch-one
|
|
EOF
|
|
git branch >actual.raw &&
|
|
grep ref-to <actual.raw >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_done
|