mirror of
https://github.com/git/git
synced 2024-10-28 19:25:47 +00:00
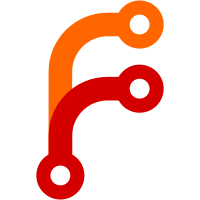
The sha1_to_hex and find_unique_abbrev functions always write into reusable static buffers. There are a few problems with this: - future calls overwrite our result. This is especially annoying with find_unique_abbrev, which does not have a ring of buffers, so you cannot even printf() a result that has two abbreviated sha1s. - if you want to put the result into another buffer, we often strcpy, which looks suspicious when auditing for overflows. This patch introduces sha1_to_hex_r and find_unique_abbrev_r, which write into a user-provided buffer. Of course this is just punting on the overflow-auditing, as the buffer obviously needs to be GIT_SHA1_HEXSZ + 1 bytes. But it is much easier to audit, since that is a well-known size. We retain the non-reentrant forms, which just become thin wrappers around the reentrant ones. This patch also adds a strbuf variant of find_unique_abbrev, which will be handy in later patches. Signed-off-by: Jeff King <peff@peff.net> Signed-off-by: Junio C Hamano <gitster@pobox.com>
90 lines
2.6 KiB
C
90 lines
2.6 KiB
C
#include "cache.h"
|
|
|
|
const signed char hexval_table[256] = {
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 00-07 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 08-0f */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 10-17 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 18-1f */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 20-27 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 28-2f */
|
|
0, 1, 2, 3, 4, 5, 6, 7, /* 30-37 */
|
|
8, 9, -1, -1, -1, -1, -1, -1, /* 38-3f */
|
|
-1, 10, 11, 12, 13, 14, 15, -1, /* 40-47 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 48-4f */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 50-57 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 58-5f */
|
|
-1, 10, 11, 12, 13, 14, 15, -1, /* 60-67 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 68-67 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 70-77 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 78-7f */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 80-87 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 88-8f */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 90-97 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* 98-9f */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* a0-a7 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* a8-af */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* b0-b7 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* b8-bf */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* c0-c7 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* c8-cf */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* d0-d7 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* d8-df */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* e0-e7 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* e8-ef */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* f0-f7 */
|
|
-1, -1, -1, -1, -1, -1, -1, -1, /* f8-ff */
|
|
};
|
|
|
|
int get_sha1_hex(const char *hex, unsigned char *sha1)
|
|
{
|
|
int i;
|
|
for (i = 0; i < GIT_SHA1_RAWSZ; i++) {
|
|
unsigned int val;
|
|
/*
|
|
* hex[1]=='\0' is caught when val is checked below,
|
|
* but if hex[0] is NUL we have to avoid reading
|
|
* past the end of the string:
|
|
*/
|
|
if (!hex[0])
|
|
return -1;
|
|
val = (hexval(hex[0]) << 4) | hexval(hex[1]);
|
|
if (val & ~0xff)
|
|
return -1;
|
|
*sha1++ = val;
|
|
hex += 2;
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
int get_oid_hex(const char *hex, struct object_id *oid)
|
|
{
|
|
return get_sha1_hex(hex, oid->hash);
|
|
}
|
|
|
|
char *sha1_to_hex_r(char *buffer, const unsigned char *sha1)
|
|
{
|
|
static const char hex[] = "0123456789abcdef";
|
|
char *buf = buffer;
|
|
int i;
|
|
|
|
for (i = 0; i < GIT_SHA1_RAWSZ; i++) {
|
|
unsigned int val = *sha1++;
|
|
*buf++ = hex[val >> 4];
|
|
*buf++ = hex[val & 0xf];
|
|
}
|
|
*buf = '\0';
|
|
|
|
return buffer;
|
|
}
|
|
|
|
char *sha1_to_hex(const unsigned char *sha1)
|
|
{
|
|
static int bufno;
|
|
static char hexbuffer[4][GIT_SHA1_HEXSZ + 1];
|
|
return sha1_to_hex_r(hexbuffer[3 & ++bufno], sha1);
|
|
}
|
|
|
|
char *oid_to_hex(const struct object_id *oid)
|
|
{
|
|
return sha1_to_hex(oid->hash);
|
|
}
|