mirror of
https://github.com/git/git
synced 2024-11-05 18:59:29 +00:00
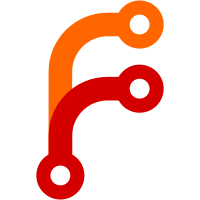
The shell+perl "[de]packetize()" helper functions were added in4414a15002
(t/lib-git-daemon: add network-protocol helpers, 2018-01-24), and around the same time we added the "pkt-line" helper in74e7002961
(test-pkt-line: introduce a packet-line test helper, 2018-03-14). For some reason it seems we've mostly used the shell+perl version instead of the helper since then. There were discussions around88124ab263
(test-lib-functions: make packetize() more efficient, 2020-03-27) andcacae4329f
(test-lib-functions: simplify packetize() stdin code, 2020-03-29) to improve them and make them more efficient. There was one good reason to do so, we needed an equivalent of "test-tool pkt-line pack", but that command wasn't capable of handling input with "\n" (a feature) or "\0" (just because it happens to be printf-based under the hood). Let's add a "pkt-line-raw" helper for that, and expose is at a packetize_raw() to go with the existing packetize() on the shell level, this gives us the smallest amount of change to the tests themselves. Signed-off-by: Ævar Arnfjörð Bjarmason <avarab@gmail.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
137 lines
2.9 KiB
C
137 lines
2.9 KiB
C
#include "cache.h"
|
|
#include "test-tool.h"
|
|
#include "pkt-line.h"
|
|
|
|
static void pack_line(const char *line)
|
|
{
|
|
if (!strcmp(line, "0000") || !strcmp(line, "0000\n"))
|
|
packet_flush(1);
|
|
else if (!strcmp(line, "0001") || !strcmp(line, "0001\n"))
|
|
packet_delim(1);
|
|
else
|
|
packet_write_fmt(1, "%s", line);
|
|
}
|
|
|
|
static void pack(int argc, const char **argv)
|
|
{
|
|
if (argc) { /* read from argv */
|
|
int i;
|
|
for (i = 0; i < argc; i++)
|
|
pack_line(argv[i]);
|
|
} else { /* read from stdin */
|
|
char line[LARGE_PACKET_MAX];
|
|
while (fgets(line, sizeof(line), stdin)) {
|
|
pack_line(line);
|
|
}
|
|
}
|
|
}
|
|
|
|
static void pack_raw_stdin(void)
|
|
{
|
|
struct strbuf sb = STRBUF_INIT;
|
|
|
|
if (strbuf_read(&sb, 0, 0) < 0)
|
|
die_errno("failed to read from stdin");
|
|
packet_write(1, sb.buf, sb.len);
|
|
strbuf_release(&sb);
|
|
}
|
|
|
|
static void unpack(void)
|
|
{
|
|
struct packet_reader reader;
|
|
packet_reader_init(&reader, 0, NULL, 0,
|
|
PACKET_READ_GENTLE_ON_EOF |
|
|
PACKET_READ_CHOMP_NEWLINE);
|
|
|
|
while (packet_reader_read(&reader) != PACKET_READ_EOF) {
|
|
switch (reader.status) {
|
|
case PACKET_READ_EOF:
|
|
break;
|
|
case PACKET_READ_NORMAL:
|
|
printf("%s\n", reader.line);
|
|
break;
|
|
case PACKET_READ_FLUSH:
|
|
printf("0000\n");
|
|
break;
|
|
case PACKET_READ_DELIM:
|
|
printf("0001\n");
|
|
break;
|
|
case PACKET_READ_RESPONSE_END:
|
|
printf("0002\n");
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
|
|
static void unpack_sideband(void)
|
|
{
|
|
struct packet_reader reader;
|
|
packet_reader_init(&reader, 0, NULL, 0,
|
|
PACKET_READ_GENTLE_ON_EOF |
|
|
PACKET_READ_CHOMP_NEWLINE);
|
|
|
|
while (packet_reader_read(&reader) != PACKET_READ_EOF) {
|
|
int band;
|
|
int fd;
|
|
|
|
switch (reader.status) {
|
|
case PACKET_READ_EOF:
|
|
break;
|
|
case PACKET_READ_NORMAL:
|
|
band = reader.line[0] & 0xff;
|
|
if (band < 1 || band > 2)
|
|
continue; /* skip non-sideband packets */
|
|
fd = band;
|
|
|
|
write_or_die(fd, reader.line + 1, reader.pktlen - 1);
|
|
break;
|
|
case PACKET_READ_FLUSH:
|
|
return;
|
|
case PACKET_READ_DELIM:
|
|
case PACKET_READ_RESPONSE_END:
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
|
|
static int send_split_sideband(void)
|
|
{
|
|
const char *part1 = "Hello,";
|
|
const char *primary = "\001primary: regular output\n";
|
|
const char *part2 = " world!\n";
|
|
|
|
send_sideband(1, 2, part1, strlen(part1), LARGE_PACKET_MAX);
|
|
packet_write(1, primary, strlen(primary));
|
|
send_sideband(1, 2, part2, strlen(part2), LARGE_PACKET_MAX);
|
|
packet_response_end(1);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int receive_sideband(void)
|
|
{
|
|
return recv_sideband("sideband", 0, 1);
|
|
}
|
|
|
|
int cmd__pkt_line(int argc, const char **argv)
|
|
{
|
|
if (argc < 2)
|
|
die("too few arguments");
|
|
|
|
if (!strcmp(argv[1], "pack"))
|
|
pack(argc - 2, argv + 2);
|
|
else if (!strcmp(argv[1], "pack-raw-stdin"))
|
|
pack_raw_stdin();
|
|
else if (!strcmp(argv[1], "unpack"))
|
|
unpack();
|
|
else if (!strcmp(argv[1], "unpack-sideband"))
|
|
unpack_sideband();
|
|
else if (!strcmp(argv[1], "send-split-sideband"))
|
|
send_split_sideband();
|
|
else if (!strcmp(argv[1], "receive-sideband"))
|
|
receive_sideband();
|
|
else
|
|
die("invalid argument '%s'", argv[1]);
|
|
|
|
return 0;
|
|
}
|