mirror of
https://github.com/git/git
synced 2024-08-24 02:11:08 +00:00
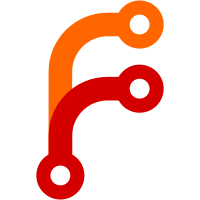
Instead of hard-coding 40-character shell patterns, use grep to determine if all of the paths have either zero or one levels of fanout, as appropriate. Note that the final test is implicitly dependent on the hash algorithm. Depending on the algorithm in use, the fanout may or may not completely compress. In its current state, this is not a problem, but it could be if the hash algorithm changes again. Signed-off-by: brian m. carlson <sandals@crustytoothpaste.net> Signed-off-by: Junio C Hamano <gitster@pobox.com>
83 lines
1.7 KiB
Bash
Executable file
83 lines
1.7 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
test_description='Test that adding/removing many notes triggers automatic fanout restructuring'
|
|
|
|
. ./test-lib.sh
|
|
|
|
test_expect_success 'creating many notes with git-notes' '
|
|
num_notes=300 &&
|
|
i=0 &&
|
|
while test $i -lt $num_notes
|
|
do
|
|
i=$(($i + 1)) &&
|
|
test_tick &&
|
|
echo "file for commit #$i" > file &&
|
|
git add file &&
|
|
git commit -q -m "commit #$i" &&
|
|
git notes add -m "note #$i" || return 1
|
|
done
|
|
'
|
|
|
|
test_expect_success 'many notes created correctly with git-notes' '
|
|
git log | grep "^ " > output &&
|
|
i=300 &&
|
|
while test $i -gt 0
|
|
do
|
|
echo " commit #$i" &&
|
|
echo " note #$i" &&
|
|
i=$(($i - 1));
|
|
done > expect &&
|
|
test_cmp expect output
|
|
'
|
|
|
|
test_expect_success 'many notes created with git-notes triggers fanout' '
|
|
# Expect entire notes tree to have a fanout == 1
|
|
git ls-tree -r --name-only refs/notes/commits |
|
|
while read path
|
|
do
|
|
echo $path | grep "^../[0-9a-f]*$" || {
|
|
echo "Invalid path \"$path\"" &&
|
|
return 1;
|
|
}
|
|
done
|
|
'
|
|
|
|
test_expect_success 'deleting most notes with git-notes' '
|
|
num_notes=250 &&
|
|
i=0 &&
|
|
git rev-list HEAD |
|
|
while test $i -lt $num_notes && read sha1
|
|
do
|
|
i=$(($i + 1)) &&
|
|
test_tick &&
|
|
git notes remove "$sha1" ||
|
|
exit 1
|
|
done
|
|
'
|
|
|
|
test_expect_success 'most notes deleted correctly with git-notes' '
|
|
git log HEAD~250 | grep "^ " > output &&
|
|
i=50 &&
|
|
while test $i -gt 0
|
|
do
|
|
echo " commit #$i" &&
|
|
echo " note #$i" &&
|
|
i=$(($i - 1));
|
|
done > expect &&
|
|
test_cmp expect output
|
|
'
|
|
|
|
test_expect_success 'deleting most notes triggers fanout consolidation' '
|
|
# Expect entire notes tree to have a fanout == 0
|
|
git ls-tree -r --name-only refs/notes/commits |
|
|
while read path
|
|
do
|
|
echo $path | grep -v "^../.*" || {
|
|
echo "Invalid path \"$path\"" &&
|
|
return 1;
|
|
}
|
|
done
|
|
'
|
|
|
|
test_done
|