mirror of
https://github.com/git/git
synced 2024-10-28 19:25:47 +00:00
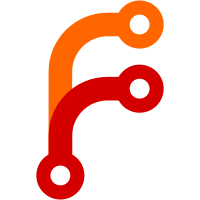
If we want to re-pack just local packfiles, we need to know whether a particular object is local or not. Signed-off-by: Linus Torvalds <torvalds@osdl.org> Signed-off-by: Junio C Hamano <junkio@cox.net>
57 lines
1.1 KiB
C
57 lines
1.1 KiB
C
#include "cache.h"
|
|
#include "pack.h"
|
|
|
|
static int verify_one_pack(char *arg, int verbose)
|
|
{
|
|
int len = strlen(arg);
|
|
struct packed_git *g;
|
|
|
|
while (1) {
|
|
/* Should name foo.idx, but foo.pack may be named;
|
|
* convert it to foo.idx
|
|
*/
|
|
if (!strcmp(arg + len - 5, ".pack")) {
|
|
strcpy(arg + len - 5, ".idx");
|
|
len--;
|
|
}
|
|
/* Should name foo.idx now */
|
|
if ((g = add_packed_git(arg, len, 1)))
|
|
break;
|
|
/* No? did you name just foo? */
|
|
strcpy(arg + len, ".idx");
|
|
len += 4;
|
|
if ((g = add_packed_git(arg, len, 1)))
|
|
break;
|
|
return error("packfile %s not found.", arg);
|
|
}
|
|
return verify_pack(g, verbose);
|
|
}
|
|
|
|
static const char verify_pack_usage[] = "git-verify-pack [-v] <pack>...";
|
|
|
|
int main(int ac, char **av)
|
|
{
|
|
int errs = 0;
|
|
int verbose = 0;
|
|
int no_more_options = 0;
|
|
|
|
while (1 < ac) {
|
|
char path[PATH_MAX];
|
|
|
|
if (!no_more_options && av[1][0] == '-') {
|
|
if (!strcmp("-v", av[1]))
|
|
verbose = 1;
|
|
else if (!strcmp("--", av[1]))
|
|
no_more_options = 1;
|
|
else
|
|
usage(verify_pack_usage);
|
|
}
|
|
else {
|
|
strcpy(path, av[1]);
|
|
if (verify_one_pack(path, verbose))
|
|
errs++;
|
|
}
|
|
ac--; av++;
|
|
}
|
|
return !!errs;
|
|
}
|