mirror of
https://github.com/git/git
synced 2024-10-28 19:25:47 +00:00
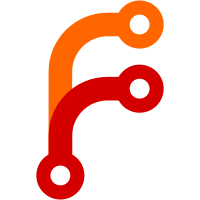
test to hash a large buffer in one go is more important than hashing large amount of data in small fixed chunks. Signed-off-by: Junio C Hamano <junkio@cox.net>
47 lines
815 B
C
47 lines
815 B
C
#include "cache.h"
|
|
|
|
int main(int ac, char **av)
|
|
{
|
|
SHA_CTX ctx;
|
|
unsigned char sha1[20];
|
|
unsigned bufsz = 8192;
|
|
char *buffer;
|
|
|
|
if (ac == 2)
|
|
bufsz = strtoul(av[1], NULL, 10) * 1024 * 1024;
|
|
|
|
if (!bufsz)
|
|
bufsz = 8192;
|
|
|
|
while ((buffer = malloc(bufsz)) == NULL) {
|
|
fprintf(stderr, "bufsz %u is too big, halving...\n", bufsz);
|
|
bufsz /= 2;
|
|
if (bufsz < 1024)
|
|
die("OOPS");
|
|
}
|
|
|
|
SHA1_Init(&ctx);
|
|
|
|
while (1) {
|
|
ssize_t sz, this_sz;
|
|
char *cp = buffer;
|
|
unsigned room = bufsz;
|
|
this_sz = 0;
|
|
while (room) {
|
|
sz = xread(0, cp, room);
|
|
if (sz == 0)
|
|
break;
|
|
if (sz < 0)
|
|
die("test-sha1: %s", strerror(errno));
|
|
this_sz += sz;
|
|
cp += sz;
|
|
room -= sz;
|
|
}
|
|
if (this_sz == 0)
|
|
break;
|
|
SHA1_Update(&ctx, buffer, this_sz);
|
|
}
|
|
SHA1_Final(sha1, &ctx);
|
|
puts(sha1_to_hex(sha1));
|
|
exit(0);
|
|
}
|