mirror of
https://github.com/git/git
synced 2024-08-27 03:29:21 +00:00
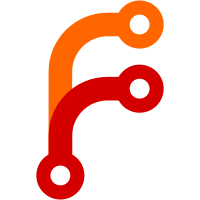
Parseopt wraps argument help strings in a pair of angular brackets by default, to tell users that they need to replace it with an actual value. This is useful in most cases, because most option arguments are indeed single values of a certain type. The option PARSE_OPT_LITERAL_ARGHELP needs to be used in option definitions with arguments that have multiple parts or are literal strings. Stop adding these angular brackets if special characters are present, as they indicate that we don't deal with a simple placeholder. This simplifies the code a bit and makes defining special options slightly easier. Remove the flag PARSE_OPT_LITERAL_ARGHELP in the cases where the new and more cautious handling suffices. Signed-off-by: Rene Scharfe <l.s.r@web.de> Signed-off-by: Junio C Hamano <gitster@pobox.com>
57 lines
1.5 KiB
C
57 lines
1.5 KiB
C
/*
|
|
* GIT - The information manager from hell
|
|
*
|
|
* Copyright (C) Linus Torvalds, 2005
|
|
*/
|
|
#include "builtin.h"
|
|
#include "cache.h"
|
|
#include "config.h"
|
|
#include "tree.h"
|
|
#include "cache-tree.h"
|
|
#include "parse-options.h"
|
|
|
|
static const char * const write_tree_usage[] = {
|
|
N_("git write-tree [--missing-ok] [--prefix=<prefix>/]"),
|
|
NULL
|
|
};
|
|
|
|
int cmd_write_tree(int argc, const char **argv, const char *unused_prefix)
|
|
{
|
|
int flags = 0, ret;
|
|
const char *prefix = NULL;
|
|
unsigned char sha1[20];
|
|
const char *me = "git-write-tree";
|
|
struct option write_tree_options[] = {
|
|
OPT_BIT(0, "missing-ok", &flags, N_("allow missing objects"),
|
|
WRITE_TREE_MISSING_OK),
|
|
OPT_STRING(0, "prefix", &prefix, N_("<prefix>/"),
|
|
N_("write tree object for a subdirectory <prefix>")),
|
|
{ OPTION_BIT, 0, "ignore-cache-tree", &flags, NULL,
|
|
N_("only useful for debugging"),
|
|
PARSE_OPT_HIDDEN | PARSE_OPT_NOARG, NULL,
|
|
WRITE_TREE_IGNORE_CACHE_TREE },
|
|
OPT_END()
|
|
};
|
|
|
|
git_config(git_default_config, NULL);
|
|
argc = parse_options(argc, argv, unused_prefix, write_tree_options,
|
|
write_tree_usage, 0);
|
|
|
|
ret = write_cache_as_tree(sha1, flags, prefix);
|
|
switch (ret) {
|
|
case 0:
|
|
printf("%s\n", sha1_to_hex(sha1));
|
|
break;
|
|
case WRITE_TREE_UNREADABLE_INDEX:
|
|
die("%s: error reading the index", me);
|
|
break;
|
|
case WRITE_TREE_UNMERGED_INDEX:
|
|
die("%s: error building trees", me);
|
|
break;
|
|
case WRITE_TREE_PREFIX_ERROR:
|
|
die("%s: prefix %s not found", me, prefix);
|
|
break;
|
|
}
|
|
return ret;
|
|
}
|