mirror of
https://github.com/git/git
synced 2024-08-24 18:26:02 +00:00
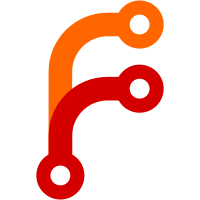
The ref-filter module provides routines for formatting a ref for output. The fundamental interface for the format is a "const char *" containing the format, and any additional options need to be passed to each invocation of show_ref_array_item. Instead, let's make a ref_format struct that holds the format, along with any associated format options. That will make some enhancements easier in the future: 1. new formatting options can be added without disrupting existing callers 2. some state can be carried in the struct rather than as global variables For now this just has the text format itself along with the quote_style option, but we'll add more fields in future patches. Signed-off-by: Jeff King <peff@peff.net> Signed-off-by: Junio C Hamano <gitster@pobox.com>
78 lines
1.9 KiB
C
78 lines
1.9 KiB
C
/*
|
|
* Builtin "git verify-tag"
|
|
*
|
|
* Copyright (c) 2007 Carlos Rica <jasampler@gmail.com>
|
|
*
|
|
* Based on git-verify-tag.sh
|
|
*/
|
|
#include "cache.h"
|
|
#include "config.h"
|
|
#include "builtin.h"
|
|
#include "tag.h"
|
|
#include "run-command.h"
|
|
#include <signal.h>
|
|
#include "parse-options.h"
|
|
#include "gpg-interface.h"
|
|
#include "ref-filter.h"
|
|
|
|
static const char * const verify_tag_usage[] = {
|
|
N_("git verify-tag [-v | --verbose] [--format=<format>] <tag>..."),
|
|
NULL
|
|
};
|
|
|
|
static int git_verify_tag_config(const char *var, const char *value, void *cb)
|
|
{
|
|
int status = git_gpg_config(var, value, cb);
|
|
if (status)
|
|
return status;
|
|
return git_default_config(var, value, cb);
|
|
}
|
|
|
|
int cmd_verify_tag(int argc, const char **argv, const char *prefix)
|
|
{
|
|
int i = 1, verbose = 0, had_error = 0;
|
|
unsigned flags = 0;
|
|
struct ref_format format = REF_FORMAT_INIT;
|
|
const struct option verify_tag_options[] = {
|
|
OPT__VERBOSE(&verbose, N_("print tag contents")),
|
|
OPT_BIT(0, "raw", &flags, N_("print raw gpg status output"), GPG_VERIFY_RAW),
|
|
OPT_STRING(0, "format", &format.format, N_("format"), N_("format to use for the output")),
|
|
OPT_END()
|
|
};
|
|
|
|
git_config(git_verify_tag_config, NULL);
|
|
|
|
argc = parse_options(argc, argv, prefix, verify_tag_options,
|
|
verify_tag_usage, PARSE_OPT_KEEP_ARGV0);
|
|
if (argc <= i)
|
|
usage_with_options(verify_tag_usage, verify_tag_options);
|
|
|
|
if (verbose)
|
|
flags |= GPG_VERIFY_VERBOSE;
|
|
|
|
if (format.format) {
|
|
if (verify_ref_format(&format))
|
|
usage_with_options(verify_tag_usage,
|
|
verify_tag_options);
|
|
flags |= GPG_VERIFY_OMIT_STATUS;
|
|
}
|
|
|
|
while (i < argc) {
|
|
unsigned char sha1[20];
|
|
const char *name = argv[i++];
|
|
if (get_sha1(name, sha1)) {
|
|
had_error = !!error("tag '%s' not found.", name);
|
|
continue;
|
|
}
|
|
|
|
if (gpg_verify_tag(sha1, name, flags)) {
|
|
had_error = 1;
|
|
continue;
|
|
}
|
|
|
|
if (format.format)
|
|
pretty_print_ref(name, sha1, &format);
|
|
}
|
|
return had_error;
|
|
}
|