mirror of
https://github.com/git/git
synced 2024-07-16 10:38:05 +00:00
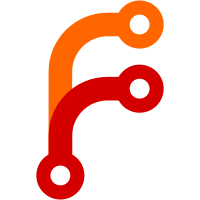
Suggesting "'reset HEAD <path>' to unstage" is dead wrong if we are about to record a merge commit. For either an unmerged path (i.e. with unresolved conflicts), or an updated path, it would result in discarding what the other branch did. Note that we do not do anything special in a case where we are amending a merge. The user is making an evil merge starting from an already committed merge, and running "reset HEAD <path>" is the right way to get rid of the local edit that has been added to the index. Once "reset --unresolve <path>" becomes available, we might want to suggest it for a merged path that has unresolve information, but until then, just remove the incorrect advice. We might also want to suggest "checkout --conflict <path>" to revert the file in the work tree to the state of failed automerge for an unmerged path, but we never did that, and this commit does not change that. Signed-off-by: Junio C Hamano <gitster@pobox.com>
64 lines
1.3 KiB
C
64 lines
1.3 KiB
C
#ifndef STATUS_H
|
|
#define STATUS_H
|
|
|
|
#include <stdio.h>
|
|
#include "string-list.h"
|
|
#include "color.h"
|
|
|
|
enum color_wt_status {
|
|
WT_STATUS_HEADER = 0,
|
|
WT_STATUS_UPDATED,
|
|
WT_STATUS_CHANGED,
|
|
WT_STATUS_UNTRACKED,
|
|
WT_STATUS_NOBRANCH,
|
|
WT_STATUS_UNMERGED,
|
|
};
|
|
|
|
enum untracked_status_type {
|
|
SHOW_NO_UNTRACKED_FILES,
|
|
SHOW_NORMAL_UNTRACKED_FILES,
|
|
SHOW_ALL_UNTRACKED_FILES
|
|
};
|
|
|
|
struct wt_status_change_data {
|
|
int worktree_status;
|
|
int index_status;
|
|
int stagemask;
|
|
char *head_path;
|
|
};
|
|
|
|
struct wt_status {
|
|
int is_initial;
|
|
char *branch;
|
|
const char *reference;
|
|
const char **pathspec;
|
|
int verbose;
|
|
int amend;
|
|
int in_merge;
|
|
int nowarn;
|
|
int use_color;
|
|
int relative_paths;
|
|
int submodule_summary;
|
|
enum untracked_status_type show_untracked_files;
|
|
char color_palette[WT_STATUS_UNMERGED+1][COLOR_MAXLEN];
|
|
|
|
/* These are computed during processing of the individual sections */
|
|
int commitable;
|
|
int workdir_dirty;
|
|
int workdir_untracked;
|
|
const char *index_file;
|
|
FILE *fp;
|
|
const char *prefix;
|
|
struct string_list change;
|
|
struct string_list untracked;
|
|
};
|
|
|
|
void wt_status_prepare(struct wt_status *s);
|
|
void wt_status_print(struct wt_status *s);
|
|
void wt_status_collect(struct wt_status *s);
|
|
|
|
void wt_shortstatus_print(struct wt_status *s, int null_termination);
|
|
void wt_porcelain_print(struct wt_status *s, int null_termination);
|
|
|
|
#endif /* STATUS_H */
|