mirror of
https://github.com/git/git
synced 2024-07-17 11:07:55 +00:00
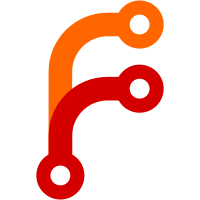
Commit dc944b65f1
(get_sha1_with_context: dynamically
allocate oc->path, 2017-05-19) changed the rules that
callers must follow for seeing if we parsed a path in the
object name. The rules switched from "check if the oc.path
buffer is empty" to "check if the oc.path pointer is NULL".
But that commit forgot to update some sites in
cat_one_file(), meaning we might dereference a NULL pointer.
You can see this by making a path-aware request like
--textconv without specifying --path, and giving an object
name that doesn't have a path in it. Like:
git cat-file --textconv HEAD
which will reliably segfault.
Signed-off-by: Jeff King <peff@peff.net>
Signed-off-by: Junio C Hamano <gitster@pobox.com>
70 lines
1.9 KiB
Bash
Executable file
70 lines
1.9 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
test_description='git cat-file filters support'
|
|
. ./test-lib.sh
|
|
|
|
test_expect_success 'setup ' '
|
|
echo "*.txt eol=crlf diff=txt" >.gitattributes &&
|
|
echo "hello" | append_cr >world.txt &&
|
|
git add .gitattributes world.txt &&
|
|
test_tick &&
|
|
git commit -m "Initial commit"
|
|
'
|
|
|
|
has_cr () {
|
|
tr '\015' Q <"$1" | grep Q >/dev/null
|
|
}
|
|
|
|
test_expect_success 'no filters with `git show`' '
|
|
git show HEAD:world.txt >actual &&
|
|
! has_cr actual
|
|
|
|
'
|
|
|
|
test_expect_success 'no filters with cat-file' '
|
|
git cat-file blob HEAD:world.txt >actual &&
|
|
! has_cr actual
|
|
'
|
|
|
|
test_expect_success 'cat-file --filters converts to worktree version' '
|
|
git cat-file --filters HEAD:world.txt >actual &&
|
|
has_cr actual
|
|
'
|
|
|
|
test_expect_success 'cat-file --filters --path=<path> works' '
|
|
sha1=$(git rev-parse -q --verify HEAD:world.txt) &&
|
|
git cat-file --filters --path=world.txt $sha1 >actual &&
|
|
has_cr actual
|
|
'
|
|
|
|
test_expect_success 'cat-file --textconv --path=<path> works' '
|
|
sha1=$(git rev-parse -q --verify HEAD:world.txt) &&
|
|
test_config diff.txt.textconv "tr A-Za-z N-ZA-Mn-za-m <" &&
|
|
git cat-file --textconv --path=hello.txt $sha1 >rot13 &&
|
|
test uryyb = "$(cat rot13 | remove_cr)"
|
|
'
|
|
|
|
test_expect_success '--path=<path> complains without --textconv/--filters' '
|
|
sha1=$(git rev-parse -q --verify HEAD:world.txt) &&
|
|
test_must_fail git cat-file --path=hello.txt blob $sha1 >actual 2>err &&
|
|
test ! -s actual &&
|
|
grep "path.*needs.*filters" err
|
|
'
|
|
|
|
test_expect_success '--textconv/--filters complain without path' '
|
|
test_must_fail git cat-file --textconv HEAD &&
|
|
test_must_fail git cat-file --filters HEAD
|
|
'
|
|
|
|
test_expect_success 'cat-file --textconv --batch works' '
|
|
sha1=$(git rev-parse -q --verify HEAD:world.txt) &&
|
|
test_config diff.txt.textconv "tr A-Za-z N-ZA-Mn-za-m <" &&
|
|
printf "%s hello.txt\n%s hello\n" $sha1 $sha1 |
|
|
git cat-file --textconv --batch >actual &&
|
|
printf "%s blob 6\nuryyb\r\n\n%s blob 6\nhello\n\n" \
|
|
$sha1 $sha1 >expect &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_done
|