mirror of
https://github.com/git/git
synced 2024-09-13 21:34:42 +00:00
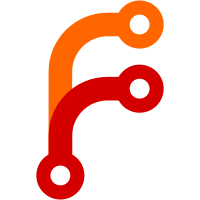
The pack bitmap format requires that we have a single bit for each object in the pack, and that each object's bitmap represents its complete set of reachable objects. Therefore we have no way to represent the bitmap of an object which references objects outside the pack. We notice this problem while generating the bitmaps, as we try to find the offset of a particular object and realize that we do not have it. In this case we die, and neither the bitmap nor the pack is generated. This is correct, but perhaps a little unfriendly. If you have bitmaps turned on in the config, many repacks will fail which would otherwise succeed. E.g., incremental repacks, repacks with "-l" when you have alternates, ".keep" files. Instead, this patch notices early that we are omitting some objects from the pack and turns off bitmaps (with a warning). Note that this is not strictly correct, as it's possible that the object being omitted is not reachable from any other object in the pack. In practice, this is almost never the case, and there are two advantages to doing it this way: 1. The code is much simpler, as we do not have to cleanly abort the bitmap-generation process midway through. 2. We do not waste time partially generating bitmaps only to find out that some object deep in the history is not being packed. Signed-off-by: Jeff King <peff@peff.net> Signed-off-by: Junio C Hamano <gitster@pobox.com>
143 lines
3.5 KiB
Bash
Executable file
143 lines
3.5 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
test_description='exercise basic bitmap functionality'
|
|
. ./test-lib.sh
|
|
|
|
test_expect_success 'setup repo with moderate-sized history' '
|
|
for i in $(test_seq 1 10); do
|
|
test_commit $i
|
|
done &&
|
|
git checkout -b other HEAD~5 &&
|
|
for i in $(test_seq 1 10); do
|
|
test_commit side-$i
|
|
done &&
|
|
git checkout master &&
|
|
blob=$(echo tagged-blob | git hash-object -w --stdin) &&
|
|
git tag tagged-blob $blob &&
|
|
git config pack.writebitmaps true &&
|
|
git config pack.writebitmaphashcache true
|
|
'
|
|
|
|
test_expect_success 'full repack creates bitmaps' '
|
|
git repack -ad &&
|
|
ls .git/objects/pack/ | grep bitmap >output &&
|
|
test_line_count = 1 output
|
|
'
|
|
|
|
test_expect_success 'rev-list --test-bitmap verifies bitmaps' '
|
|
git rev-list --test-bitmap HEAD
|
|
'
|
|
|
|
rev_list_tests() {
|
|
state=$1
|
|
|
|
test_expect_success "counting commits via bitmap ($state)" '
|
|
git rev-list --count HEAD >expect &&
|
|
git rev-list --use-bitmap-index --count HEAD >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success "counting partial commits via bitmap ($state)" '
|
|
git rev-list --count HEAD~5..HEAD >expect &&
|
|
git rev-list --use-bitmap-index --count HEAD~5..HEAD >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success "counting non-linear history ($state)" '
|
|
git rev-list --count other...master >expect &&
|
|
git rev-list --use-bitmap-index --count other...master >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success "enumerate --objects ($state)" '
|
|
git rev-list --objects --use-bitmap-index HEAD >tmp &&
|
|
cut -d" " -f1 <tmp >tmp2 &&
|
|
sort <tmp2 >actual &&
|
|
git rev-list --objects HEAD >tmp &&
|
|
cut -d" " -f1 <tmp >tmp2 &&
|
|
sort <tmp2 >expect &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success "bitmap --objects handles non-commit objects ($state)" '
|
|
git rev-list --objects --use-bitmap-index HEAD tagged-blob >actual &&
|
|
grep $blob actual
|
|
'
|
|
}
|
|
|
|
rev_list_tests 'full bitmap'
|
|
|
|
test_expect_success 'clone from bitmapped repository' '
|
|
git clone --no-local --bare . clone.git &&
|
|
git rev-parse HEAD >expect &&
|
|
git --git-dir=clone.git rev-parse HEAD >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success 'setup further non-bitmapped commits' '
|
|
for i in $(test_seq 1 10); do
|
|
test_commit further-$i
|
|
done
|
|
'
|
|
|
|
rev_list_tests 'partial bitmap'
|
|
|
|
test_expect_success 'fetch (partial bitmap)' '
|
|
git --git-dir=clone.git fetch origin master:master &&
|
|
git rev-parse HEAD >expect &&
|
|
git --git-dir=clone.git rev-parse HEAD >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success 'incremental repack cannot create bitmaps' '
|
|
test_commit more-1 &&
|
|
find .git/objects/pack -name "*.bitmap" >expect &&
|
|
git repack -d &&
|
|
find .git/objects/pack -name "*.bitmap" >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success 'incremental repack can disable bitmaps' '
|
|
test_commit more-2 &&
|
|
git repack -d --no-write-bitmap-index
|
|
'
|
|
|
|
test_expect_success 'full repack, reusing previous bitmaps' '
|
|
git repack -ad &&
|
|
ls .git/objects/pack/ | grep bitmap >output &&
|
|
test_line_count = 1 output
|
|
'
|
|
|
|
test_expect_success 'fetch (full bitmap)' '
|
|
git --git-dir=clone.git fetch origin master:master &&
|
|
git rev-parse HEAD >expect &&
|
|
git --git-dir=clone.git rev-parse HEAD >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_lazy_prereq JGIT '
|
|
type jgit
|
|
'
|
|
|
|
test_expect_success JGIT 'we can read jgit bitmaps' '
|
|
git clone . compat-jgit &&
|
|
(
|
|
cd compat-jgit &&
|
|
rm -f .git/objects/pack/*.bitmap &&
|
|
jgit gc &&
|
|
git rev-list --test-bitmap HEAD
|
|
)
|
|
'
|
|
|
|
test_expect_success JGIT 'jgit can read our bitmaps' '
|
|
git clone . compat-us &&
|
|
(
|
|
cd compat-us &&
|
|
git repack -adb &&
|
|
# jgit gc will barf if it does not like our bitmaps
|
|
jgit gc
|
|
)
|
|
'
|
|
|
|
test_done
|