mirror of
https://github.com/git/git
synced 2024-09-13 21:34:42 +00:00
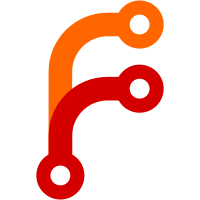
This adds the ability to perform real merges rather than just trivial merges (meaning handling three way content merges, recursive ancestor consolidation, renames, proper directory/file conflict handling, and so forth). However, unlike `git merge`, the working tree and index are left alone and no branch is updated. The only output is: - the toplevel resulting tree printed on stdout - exit status of 0 (clean), 1 (conflicts present), anything else (merge could not be performed; unknown if clean or conflicted) This output is meant to be used by some higher level script, perhaps in a sequence of steps like this: NEWTREE=$(git merge-tree --write-tree $BRANCH1 $BRANCH2) test $? -eq 0 || die "There were conflicts..." NEWCOMMIT=$(git commit-tree $NEWTREE -p $BRANCH1 -p $BRANCH2) git update-ref $BRANCH1 $NEWCOMMIT Note that higher level scripts may also want to access the conflict/warning messages normally output during a merge, or have quick access to a list of files with conflicts. That is not available in this preliminary implementation, but subsequent commits will add that ability (meaning that NEWTREE would be a lot more than a tree in the case of conflicts). This also marks the traditional trivial merge of merge-tree as deprecated. The trivial merge not only had limited applicability, the output format was also difficult to work with (and its format undocumented), and will generally be less performant than real merges. Signed-off-by: Elijah Newren <newren@gmail.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
107 lines
2.9 KiB
Bash
Executable file
107 lines
2.9 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
test_description='git merge-tree --write-tree'
|
|
|
|
. ./test-lib.sh
|
|
|
|
# This test is ort-specific
|
|
if test "$GIT_TEST_MERGE_ALGORITHM" != "ort"
|
|
then
|
|
skip_all="GIT_TEST_MERGE_ALGORITHM != ort"
|
|
test_done
|
|
fi
|
|
|
|
test_expect_success setup '
|
|
test_write_lines 1 2 3 4 5 >numbers &&
|
|
echo hello >greeting &&
|
|
echo foo >whatever &&
|
|
git add numbers greeting whatever &&
|
|
test_tick &&
|
|
git commit -m initial &&
|
|
|
|
git branch side1 &&
|
|
git branch side2 &&
|
|
git branch side3 &&
|
|
|
|
git checkout side1 &&
|
|
test_write_lines 1 2 3 4 5 6 >numbers &&
|
|
echo hi >greeting &&
|
|
echo bar >whatever &&
|
|
git add numbers greeting whatever &&
|
|
test_tick &&
|
|
git commit -m modify-stuff &&
|
|
|
|
git checkout side2 &&
|
|
test_write_lines 0 1 2 3 4 5 >numbers &&
|
|
echo yo >greeting &&
|
|
git rm whatever &&
|
|
mkdir whatever &&
|
|
>whatever/empty &&
|
|
git add numbers greeting whatever/empty &&
|
|
test_tick &&
|
|
git commit -m other-modifications &&
|
|
|
|
git checkout side3 &&
|
|
git mv numbers sequence &&
|
|
test_tick &&
|
|
git commit -m rename-numbers
|
|
'
|
|
|
|
test_expect_success 'Clean merge' '
|
|
TREE_OID=$(git merge-tree --write-tree side1 side3) &&
|
|
q_to_tab <<-EOF >expect &&
|
|
100644 blob $(git rev-parse side1:greeting)Qgreeting
|
|
100644 blob $(git rev-parse side1:numbers)Qsequence
|
|
100644 blob $(git rev-parse side1:whatever)Qwhatever
|
|
EOF
|
|
|
|
git ls-tree $TREE_OID >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success 'Content merge and a few conflicts' '
|
|
git checkout side1^0 &&
|
|
test_must_fail git merge side2 &&
|
|
expected_tree=$(git rev-parse AUTO_MERGE) &&
|
|
|
|
# We will redo the merge, while we are still in a conflicted state!
|
|
test_when_finished "git reset --hard" &&
|
|
|
|
test_expect_code 1 git merge-tree --write-tree side1 side2 >RESULT &&
|
|
actual_tree=$(head -n 1 RESULT) &&
|
|
|
|
# Due to differences of e.g. "HEAD" vs "side1", the results will not
|
|
# exactly match. Dig into individual files.
|
|
|
|
# Numbers should have three-way merged cleanly
|
|
test_write_lines 0 1 2 3 4 5 6 >expect &&
|
|
git show ${actual_tree}:numbers >actual &&
|
|
test_cmp expect actual &&
|
|
|
|
# whatever and whatever~<branch> should have same HASHES
|
|
git rev-parse ${expected_tree}:whatever ${expected_tree}:whatever~HEAD >expect &&
|
|
git rev-parse ${actual_tree}:whatever ${actual_tree}:whatever~side1 >actual &&
|
|
test_cmp expect actual &&
|
|
|
|
# greeting should have a merge conflict
|
|
git show ${expected_tree}:greeting >tmp &&
|
|
sed -e s/HEAD/side1/ tmp >expect &&
|
|
git show ${actual_tree}:greeting >actual &&
|
|
test_cmp expect actual
|
|
'
|
|
|
|
test_expect_success 'Barf on misspelled option, with exit code other than 0 or 1' '
|
|
# Mis-spell with single "s" instead of double "s"
|
|
test_expect_code 129 git merge-tree --write-tree --mesages FOOBAR side1 side2 2>expect &&
|
|
|
|
grep "error: unknown option.*mesages" expect
|
|
'
|
|
|
|
test_expect_success 'Barf on too many arguments' '
|
|
test_expect_code 129 git merge-tree --write-tree side1 side2 invalid 2>expect &&
|
|
|
|
grep "^usage: git merge-tree" expect
|
|
'
|
|
|
|
test_done
|