mirror of
https://github.com/git/git
synced 2024-10-01 06:05:20 +00:00
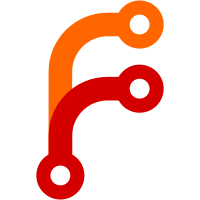
Add initialization and discard functions to mem_pool type. As the memory allocated by mem_pool can now be freed, we also track the large allocations. If the there are existing mp_blocks in the mem_poo's linked list of mp_blocksl, then the mp_block for a large allocation is inserted behind the head block. This is because only the head mp_block is considered when searching for availble space. This results in the following desirable properties: 1) The mp_block allocated for the large request will not be included not included in the search for available in future requests, the large mp_block is sized for the specific request and does not contain any spare space. 2) The head mp_block will not bumped from considation for future memory requests just because a request for a large chunk of memory came in. These changes are in preparation for a future commit that will utilize creating and discarding memory pool. Signed-off-by: Jameson Miller <jamill@microsoft.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
45 lines
936 B
C
45 lines
936 B
C
#ifndef MEM_POOL_H
|
|
#define MEM_POOL_H
|
|
|
|
struct mp_block {
|
|
struct mp_block *next_block;
|
|
char *next_free;
|
|
char *end;
|
|
uintmax_t space[FLEX_ARRAY]; /* more */
|
|
};
|
|
|
|
struct mem_pool {
|
|
struct mp_block *mp_block;
|
|
|
|
/*
|
|
* The amount of available memory to grow the pool by.
|
|
* This size does not include the overhead for the mp_block.
|
|
*/
|
|
size_t block_alloc;
|
|
|
|
/* The total amount of memory allocated by the pool. */
|
|
size_t pool_alloc;
|
|
};
|
|
|
|
/*
|
|
* Initialize mem_pool with specified initial size.
|
|
*/
|
|
void mem_pool_init(struct mem_pool **mem_pool, size_t initial_size);
|
|
|
|
/*
|
|
* Discard a memory pool and free all the memory it is responsible for.
|
|
*/
|
|
void mem_pool_discard(struct mem_pool *mem_pool);
|
|
|
|
/*
|
|
* Alloc memory from the mem_pool.
|
|
*/
|
|
void *mem_pool_alloc(struct mem_pool *pool, size_t len);
|
|
|
|
/*
|
|
* Allocate and zero memory from the memory pool.
|
|
*/
|
|
void *mem_pool_calloc(struct mem_pool *pool, size_t count, size_t size);
|
|
|
|
#endif
|