mirror of
https://github.com/git/git
synced 2024-10-12 19:42:35 +00:00
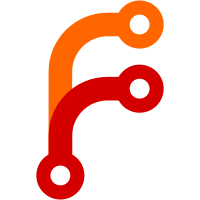
The "trivial merge" codepath wants to optimize itself by making an internal call to the read-tree machinery, but it does not read the index before doing so, and the codepath is never exercised. Incidentally, this failure to read the index upfront means that the safety to refuse doing anything when the index is unmerged does not kick in, either. These two problem are fixed by using read_cache_unmerged() that does read the index before checking if it is unmerged at the beginning of cmd_merge(). The primary logic of the merge, however, assumes that the process never reads the index in-core, and the call to write_cache_as_tree() it makes from write_tree_trivial() will always read from the on-disk index that is prepared the strategy back-ends. This assumption is now broken by the above fix. To fix this issue, we now call discard_cache() before calling write_tree_trivial() when it wants to write the on-disk index as a tree. When multiple strategies are tried, their results are evaluated by reading the resulting index and inspecting it. The codepath needs to make a call to read_cache() for each successful strategy, and for that to work, they need to discard_cache() the one read by the previous round. Also the "trivial merge" forgot that the current commit is one of the parents of the resulting commit. Signed-off-by: Junio C Hamano <gitster@pobox.com>
511 lines
9.6 KiB
Bash
Executable file
511 lines
9.6 KiB
Bash
Executable file
#!/bin/sh
|
|
#
|
|
# Copyright (c) 2007 Lars Hjemli
|
|
#
|
|
|
|
test_description='git-merge
|
|
|
|
Testing basic merge operations/option parsing.'
|
|
|
|
. ./test-lib.sh
|
|
|
|
cat >file <<EOF
|
|
1
|
|
2
|
|
3
|
|
4
|
|
5
|
|
6
|
|
7
|
|
8
|
|
9
|
|
EOF
|
|
|
|
cat >file.1 <<EOF
|
|
1 X
|
|
2
|
|
3
|
|
4
|
|
5
|
|
6
|
|
7
|
|
8
|
|
9
|
|
EOF
|
|
|
|
cat >file.5 <<EOF
|
|
1
|
|
2
|
|
3
|
|
4
|
|
5 X
|
|
6
|
|
7
|
|
8
|
|
9
|
|
EOF
|
|
|
|
cat >file.9 <<EOF
|
|
1
|
|
2
|
|
3
|
|
4
|
|
5
|
|
6
|
|
7
|
|
8
|
|
9 X
|
|
EOF
|
|
|
|
cat >result.1 <<EOF
|
|
1 X
|
|
2
|
|
3
|
|
4
|
|
5
|
|
6
|
|
7
|
|
8
|
|
9
|
|
EOF
|
|
|
|
cat >result.1-5 <<EOF
|
|
1 X
|
|
2
|
|
3
|
|
4
|
|
5 X
|
|
6
|
|
7
|
|
8
|
|
9
|
|
EOF
|
|
|
|
cat >result.1-5-9 <<EOF
|
|
1 X
|
|
2
|
|
3
|
|
4
|
|
5 X
|
|
6
|
|
7
|
|
8
|
|
9 X
|
|
EOF
|
|
|
|
create_merge_msgs() {
|
|
echo "Merge commit 'c2'" >msg.1-5 &&
|
|
echo "Merge commit 'c2'; commit 'c3'" >msg.1-5-9 &&
|
|
echo "Squashed commit of the following:" >squash.1 &&
|
|
echo >>squash.1 &&
|
|
git log --no-merges ^HEAD c1 >>squash.1 &&
|
|
echo "Squashed commit of the following:" >squash.1-5 &&
|
|
echo >>squash.1-5 &&
|
|
git log --no-merges ^HEAD c2 >>squash.1-5 &&
|
|
echo "Squashed commit of the following:" >squash.1-5-9 &&
|
|
echo >>squash.1-5-9 &&
|
|
git log --no-merges ^HEAD c2 c3 >>squash.1-5-9 &&
|
|
echo > msg.nolog &&
|
|
echo "* commit 'c3':" >msg.log &&
|
|
echo " commit 3" >>msg.log &&
|
|
echo >>msg.log
|
|
}
|
|
|
|
verify_diff() {
|
|
if ! test_cmp "$1" "$2"
|
|
then
|
|
echo "$3"
|
|
false
|
|
fi
|
|
}
|
|
|
|
verify_merge() {
|
|
verify_diff "$2" "$1" "[OOPS] bad merge result" &&
|
|
if test $(git ls-files -u | wc -l) -gt 0
|
|
then
|
|
echo "[OOPS] unmerged files"
|
|
false
|
|
fi &&
|
|
if test_must_fail git diff --exit-code
|
|
then
|
|
echo "[OOPS] working tree != index"
|
|
false
|
|
fi &&
|
|
if test -n "$3"
|
|
then
|
|
git show -s --pretty=format:%s HEAD >msg.act &&
|
|
verify_diff "$3" msg.act "[OOPS] bad merge message"
|
|
fi
|
|
}
|
|
|
|
verify_head() {
|
|
if test "$1" != "$(git rev-parse HEAD)"
|
|
then
|
|
echo "[OOPS] HEAD != $1"
|
|
false
|
|
fi
|
|
}
|
|
|
|
verify_parents() {
|
|
i=1
|
|
while test $# -gt 0
|
|
do
|
|
if test "$1" != "$(git rev-parse HEAD^$i)"
|
|
then
|
|
echo "[OOPS] HEAD^$i != $1"
|
|
return 1
|
|
fi
|
|
i=$(expr $i + 1)
|
|
shift
|
|
done
|
|
}
|
|
|
|
verify_mergeheads() {
|
|
i=1
|
|
if ! test -f .git/MERGE_HEAD
|
|
then
|
|
echo "[OOPS] MERGE_HEAD is missing"
|
|
false
|
|
fi &&
|
|
while test $# -gt 0
|
|
do
|
|
head=$(head -n $i .git/MERGE_HEAD | sed -ne \$p)
|
|
if test "$1" != "$head"
|
|
then
|
|
echo "[OOPS] MERGE_HEAD $i != $1"
|
|
return 1
|
|
fi
|
|
i=$(expr $i + 1)
|
|
shift
|
|
done
|
|
}
|
|
|
|
verify_no_mergehead() {
|
|
if test -f .git/MERGE_HEAD
|
|
then
|
|
echo "[OOPS] MERGE_HEAD exists"
|
|
false
|
|
fi
|
|
}
|
|
|
|
|
|
test_expect_success 'setup' '
|
|
git add file &&
|
|
test_tick &&
|
|
git commit -m "commit 0" &&
|
|
git tag c0 &&
|
|
c0=$(git rev-parse HEAD) &&
|
|
cp file.1 file &&
|
|
git add file &&
|
|
test_tick &&
|
|
git commit -m "commit 1" &&
|
|
git tag c1 &&
|
|
c1=$(git rev-parse HEAD) &&
|
|
git reset --hard "$c0" &&
|
|
cp file.5 file &&
|
|
git add file &&
|
|
test_tick &&
|
|
git commit -m "commit 2" &&
|
|
git tag c2 &&
|
|
c2=$(git rev-parse HEAD) &&
|
|
git reset --hard "$c0" &&
|
|
cp file.9 file &&
|
|
git add file &&
|
|
test_tick &&
|
|
git commit -m "commit 3" &&
|
|
git tag c3 &&
|
|
c3=$(git rev-parse HEAD)
|
|
git reset --hard "$c0" &&
|
|
create_merge_msgs
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'test option parsing' '
|
|
test_must_fail git merge -$ c1 &&
|
|
test_must_fail git merge --no-such c1 &&
|
|
test_must_fail git merge -s foobar c1 &&
|
|
test_must_fail git merge -s=foobar c1 &&
|
|
test_must_fail git merge -m &&
|
|
test_must_fail git merge
|
|
'
|
|
|
|
test_expect_success 'merge c0 with c1' '
|
|
git reset --hard c0 &&
|
|
git merge c1 &&
|
|
verify_merge file result.1 &&
|
|
verify_head "$c1"
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c2' '
|
|
git reset --hard c1 &&
|
|
test_tick &&
|
|
git merge c2 &&
|
|
verify_merge file result.1-5 msg.1-5 &&
|
|
verify_parents $c1 $c2
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c2 and c3' '
|
|
git reset --hard c1 &&
|
|
test_tick &&
|
|
git merge c2 c3 &&
|
|
verify_merge file result.1-5-9 msg.1-5-9 &&
|
|
verify_parents $c1 $c2 $c3
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c0 with c1 (no-commit)' '
|
|
git reset --hard c0 &&
|
|
git merge --no-commit c1 &&
|
|
verify_merge file result.1 &&
|
|
verify_head $c1
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c2 (no-commit)' '
|
|
git reset --hard c1 &&
|
|
git merge --no-commit c2 &&
|
|
verify_merge file result.1-5 &&
|
|
verify_head $c1 &&
|
|
verify_mergeheads $c2
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c2 and c3 (no-commit)' '
|
|
git reset --hard c1 &&
|
|
git merge --no-commit c2 c3 &&
|
|
verify_merge file result.1-5-9 &&
|
|
verify_head $c1 &&
|
|
verify_mergeheads $c2 $c3
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c0 with c1 (squash)' '
|
|
git reset --hard c0 &&
|
|
git merge --squash c1 &&
|
|
verify_merge file result.1 &&
|
|
verify_head $c0 &&
|
|
verify_no_mergehead &&
|
|
verify_diff squash.1 .git/SQUASH_MSG "[OOPS] bad squash message"
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c2 (squash)' '
|
|
git reset --hard c1 &&
|
|
git merge --squash c2 &&
|
|
verify_merge file result.1-5 &&
|
|
verify_head $c1 &&
|
|
verify_no_mergehead &&
|
|
verify_diff squash.1-5 .git/SQUASH_MSG "[OOPS] bad squash message"
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c2 and c3 (squash)' '
|
|
git reset --hard c1 &&
|
|
git merge --squash c2 c3 &&
|
|
verify_merge file result.1-5-9 &&
|
|
verify_head $c1 &&
|
|
verify_no_mergehead &&
|
|
verify_diff squash.1-5-9 .git/SQUASH_MSG "[OOPS] bad squash message"
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c2 (no-commit in config)' '
|
|
git reset --hard c1 &&
|
|
git config branch.master.mergeoptions "--no-commit" &&
|
|
git merge c2 &&
|
|
verify_merge file result.1-5 &&
|
|
verify_head $c1 &&
|
|
verify_mergeheads $c2
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c2 (squash in config)' '
|
|
git reset --hard c1 &&
|
|
git config branch.master.mergeoptions "--squash" &&
|
|
git merge c2 &&
|
|
verify_merge file result.1-5 &&
|
|
verify_head $c1 &&
|
|
verify_no_mergehead &&
|
|
verify_diff squash.1-5 .git/SQUASH_MSG "[OOPS] bad squash message"
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'override config option -n with --summary' '
|
|
git reset --hard c1 &&
|
|
git config branch.master.mergeoptions "-n" &&
|
|
test_tick &&
|
|
git merge --summary c2 >diffstat.txt &&
|
|
verify_merge file result.1-5 msg.1-5 &&
|
|
verify_parents $c1 $c2 &&
|
|
if ! grep "^ file | *2 +-$" diffstat.txt
|
|
then
|
|
echo "[OOPS] diffstat was not generated with --summary"
|
|
false
|
|
fi
|
|
'
|
|
|
|
test_expect_success 'override config option -n with --stat' '
|
|
git reset --hard c1 &&
|
|
git config branch.master.mergeoptions "-n" &&
|
|
test_tick &&
|
|
git merge --stat c2 >diffstat.txt &&
|
|
verify_merge file result.1-5 msg.1-5 &&
|
|
verify_parents $c1 $c2 &&
|
|
if ! grep "^ file | *2 +-$" diffstat.txt
|
|
then
|
|
echo "[OOPS] diffstat was not generated with --stat"
|
|
false
|
|
fi
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'override config option --stat' '
|
|
git reset --hard c1 &&
|
|
git config branch.master.mergeoptions "--stat" &&
|
|
test_tick &&
|
|
git merge -n c2 >diffstat.txt &&
|
|
verify_merge file result.1-5 msg.1-5 &&
|
|
verify_parents $c1 $c2 &&
|
|
if grep "^ file | *2 +-$" diffstat.txt
|
|
then
|
|
echo "[OOPS] diffstat was generated"
|
|
false
|
|
fi
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c2 (override --no-commit)' '
|
|
git reset --hard c1 &&
|
|
git config branch.master.mergeoptions "--no-commit" &&
|
|
test_tick &&
|
|
git merge --commit c2 &&
|
|
verify_merge file result.1-5 msg.1-5 &&
|
|
verify_parents $c1 $c2
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c2 (override --squash)' '
|
|
git reset --hard c1 &&
|
|
git config branch.master.mergeoptions "--squash" &&
|
|
test_tick &&
|
|
git merge --no-squash c2 &&
|
|
verify_merge file result.1-5 msg.1-5 &&
|
|
verify_parents $c1 $c2
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c0 with c1 (no-ff)' '
|
|
git reset --hard c0 &&
|
|
git config branch.master.mergeoptions "" &&
|
|
test_tick &&
|
|
git merge --no-ff c1 &&
|
|
verify_merge file result.1 &&
|
|
verify_parents $c0 $c1
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'combining --squash and --no-ff is refused' '
|
|
test_must_fail git merge --squash --no-ff c1 &&
|
|
test_must_fail git merge --no-ff --squash c1
|
|
'
|
|
|
|
test_expect_success 'merge c0 with c1 (ff overrides no-ff)' '
|
|
git reset --hard c0 &&
|
|
git config branch.master.mergeoptions "--no-ff" &&
|
|
git merge --ff c1 &&
|
|
verify_merge file result.1 &&
|
|
verify_head $c1
|
|
'
|
|
|
|
test_expect_success 'merge log message' '
|
|
git reset --hard c0 &&
|
|
git merge --no-log c2 &&
|
|
git show -s --pretty=format:%b HEAD >msg.act &&
|
|
verify_diff msg.nolog msg.act "[OOPS] bad merge log message" &&
|
|
|
|
git merge --log c3 &&
|
|
git show -s --pretty=format:%b HEAD >msg.act &&
|
|
verify_diff msg.log msg.act "[OOPS] bad merge log message" &&
|
|
|
|
git reset --hard HEAD^ &&
|
|
git config merge.log yes &&
|
|
git merge c3 &&
|
|
git show -s --pretty=format:%b HEAD >msg.act &&
|
|
verify_diff msg.log msg.act "[OOPS] bad merge log message"
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c0, c2, c0, and c1' '
|
|
git reset --hard c1 &&
|
|
git config branch.master.mergeoptions "" &&
|
|
test_tick &&
|
|
git merge c0 c2 c0 c1 &&
|
|
verify_merge file result.1-5 &&
|
|
verify_parents $c1 $c2
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c0, c2, c0, and c1' '
|
|
git reset --hard c1 &&
|
|
git config branch.master.mergeoptions "" &&
|
|
test_tick &&
|
|
git merge c0 c2 c0 c1 &&
|
|
verify_merge file result.1-5 &&
|
|
verify_parents $c1 $c2
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge c1 with c1 and c2' '
|
|
git reset --hard c1 &&
|
|
git config branch.master.mergeoptions "" &&
|
|
test_tick &&
|
|
git merge c1 c2 &&
|
|
verify_merge file result.1-5 &&
|
|
verify_parents $c1 $c2
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'merge fast-forward in a dirty tree' '
|
|
git reset --hard c0 &&
|
|
mv file file1 &&
|
|
cat file1 >file &&
|
|
rm -f file1 &&
|
|
git merge c2
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_expect_success 'in-index merge' '
|
|
git reset --hard c0 &&
|
|
git merge --no-ff -s resolve c1 > out &&
|
|
grep "Wonderful." out &&
|
|
verify_parents $c0 $c1
|
|
'
|
|
|
|
test_debug 'gitk --all'
|
|
|
|
test_done
|