mirror of
https://github.com/git/git
synced 2024-08-24 18:26:02 +00:00
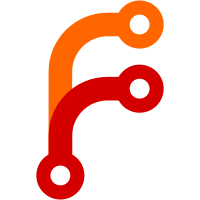
Originally, test_expect_failure was designed to be the opposite of test_expect_success, but this was a bad decision. Most tests run a series of commands that leads to the single command that needs to be tested, like this: test_expect_{success,failure} 'test title' ' setup1 && setup2 && setup3 && what is to be tested ' And expecting a failure exit from the whole sequence misses the point of writing tests. Your setup$N that are supposed to succeed may have failed without even reaching what you are trying to test. The only valid use of test_expect_failure is to check a trivial single command that is expected to fail, which is a minority in tests of Porcelain-ish commands. This large-ish patch rewrites all uses of test_expect_failure to use test_expect_success and rewrites the condition of what is tested, like this: test_expect_success 'test title' ' setup1 && setup2 && setup3 && ! this command should fail ' test_expect_failure is redefined to serve as a reminder that that test *should* succeed but due to a known breakage in git it currently does not pass. So if git-foo command should create a file 'bar' but you discovered a bug that it doesn't, you can write a test like this: test_expect_failure 'git-foo should create bar' ' rm -f bar && git foo && test -f bar ' This construct acts similar to test_expect_success, but instead of reporting "ok/FAIL" like test_expect_success does, the outcome is reported as "FIXED/still broken". Signed-off-by: Junio C Hamano <gitster@pobox.com>
64 lines
1.7 KiB
Bash
Executable file
64 lines
1.7 KiB
Bash
Executable file
#!/bin/sh
|
|
#
|
|
# Copyright (c) 2007 Eric Wong
|
|
test_description='git-svn dcommit clobber series'
|
|
. ./lib-git-svn.sh
|
|
|
|
test_expect_success 'initialize repo' "
|
|
mkdir import &&
|
|
cd import &&
|
|
awk 'BEGIN { for (i = 1; i < 64; i++) { print i } }' > file
|
|
svn import -m 'initial' . $svnrepo &&
|
|
cd .. &&
|
|
git svn init $svnrepo &&
|
|
git svn fetch &&
|
|
test -e file
|
|
"
|
|
|
|
test_expect_success '(supposedly) non-conflicting change from SVN' "
|
|
test x\"\`sed -n -e 58p < file\`\" = x58 &&
|
|
test x\"\`sed -n -e 61p < file\`\" = x61 &&
|
|
svn co $svnrepo tmp &&
|
|
cd tmp &&
|
|
perl -i -p -e 's/^58\$/5588/' file &&
|
|
perl -i -p -e 's/^61\$/6611/' file &&
|
|
poke file &&
|
|
test x\"\`sed -n -e 58p < file\`\" = x5588 &&
|
|
test x\"\`sed -n -e 61p < file\`\" = x6611 &&
|
|
svn commit -m '58 => 5588, 61 => 6611' &&
|
|
cd ..
|
|
"
|
|
|
|
test_expect_success 'some unrelated changes to git' "
|
|
echo hi > life &&
|
|
git update-index --add life &&
|
|
git commit -m hi-life &&
|
|
echo bye >> life &&
|
|
git commit -m bye-life life
|
|
"
|
|
|
|
test_expect_success 'change file but in unrelated area' "
|
|
test x\"\`sed -n -e 4p < file\`\" = x4 &&
|
|
test x\"\`sed -n -e 7p < file\`\" = x7 &&
|
|
perl -i -p -e 's/^4\$/4444/' file &&
|
|
perl -i -p -e 's/^7\$/7777/' file &&
|
|
test x\"\`sed -n -e 4p < file\`\" = x4444 &&
|
|
test x\"\`sed -n -e 7p < file\`\" = x7777 &&
|
|
git commit -m '4 => 4444, 7 => 7777' file &&
|
|
git svn dcommit &&
|
|
svn up tmp &&
|
|
cd tmp &&
|
|
test x\"\`sed -n -e 4p < file\`\" = x4444 &&
|
|
test x\"\`sed -n -e 7p < file\`\" = x7777 &&
|
|
test x\"\`sed -n -e 58p < file\`\" = x5588 &&
|
|
test x\"\`sed -n -e 61p < file\`\" = x6611
|
|
"
|
|
|
|
test_expect_success 'attempt to dcommit with a dirty index' '
|
|
echo foo >>file &&
|
|
git add file &&
|
|
! git svn dcommit
|
|
'
|
|
|
|
test_done
|