mirror of
https://github.com/git/git
synced 2024-08-27 11:39:22 +00:00
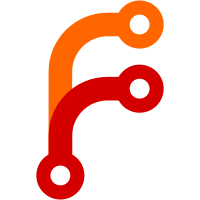
Rather than making the C library search for git every time we want to execute it we now search for the main git wrapper at startup, do symlink resolution, and then always use the absolute path that we found to execute the binary later on. This should save us some cycles, especially on stat challenged systems like Cygwin/Win32. While I was working on this change I also converted all of our existing pipes ([open "| git ..."]) to use two new pipe wrapper functions. These functions take additional options like --nice and --stderr which instructs Tcl to take special action, like running the underlying git program through `nice` (if available) or redirect stderr to stdout for capture in Tcl. Signed-off-by: Shawn O. Pearce <spearce@spearce.org>
39 lines
839 B
Tcl
39 lines
839 B
Tcl
# git-gui branch (create/delete) support
|
|
# Copyright (C) 2006, 2007 Shawn Pearce
|
|
|
|
proc load_all_heads {} {
|
|
global some_heads_tracking
|
|
|
|
set rh refs/heads
|
|
set rh_len [expr {[string length $rh] + 1}]
|
|
set all_heads [list]
|
|
set fd [git_read for-each-ref --format=%(refname) $rh]
|
|
while {[gets $fd line] > 0} {
|
|
if {!$some_heads_tracking || ![is_tracking_branch $line]} {
|
|
lappend all_heads [string range $line $rh_len end]
|
|
}
|
|
}
|
|
close $fd
|
|
|
|
return [lsort $all_heads]
|
|
}
|
|
|
|
proc load_all_tags {} {
|
|
set all_tags [list]
|
|
set fd [git_read for-each-ref \
|
|
--sort=-taggerdate \
|
|
--format=%(refname) \
|
|
refs/tags]
|
|
while {[gets $fd line] > 0} {
|
|
if {![regsub ^refs/tags/ $line {} name]} continue
|
|
lappend all_tags $name
|
|
}
|
|
close $fd
|
|
return $all_tags
|
|
}
|
|
|
|
proc radio_selector {varname value args} {
|
|
upvar #0 $varname var
|
|
set var $value
|
|
}
|