mirror of
https://github.com/git/git
synced 2024-09-13 21:34:42 +00:00
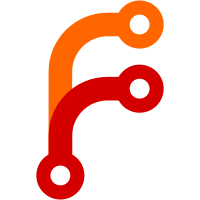
When defined, this allows plumbing commands that update the index (add, apply, checkout-index, merge-recursive, mv, read-tree, rm, update-index, and write-tree) to write their resulting index to an alternative index file while holding a lock to the original index file. With this, git-commit that jumps the index does not have to make an extra copy of the index file, and more importantly, it can do the update while holding the lock on the index. However, I think the interface to let an environment variable specify the output is a mistake, as shown in the documentation. If a curious user has the environment variable set to something other than the file GIT_INDEX_FILE points at, almost everything will break. This should instead be a command line parameter to tell these plumbing commands to write the result in the named file, to prevent stupid mistakes. Signed-off-by: Junio C Hamano <junkio@cox.net>
88 lines
2.1 KiB
C
88 lines
2.1 KiB
C
/*
|
|
* GIT - The information manager from hell
|
|
*
|
|
* Copyright (C) Linus Torvalds, 2005
|
|
*/
|
|
#include "builtin.h"
|
|
#include "cache.h"
|
|
#include "tree.h"
|
|
#include "cache-tree.h"
|
|
|
|
static const char write_tree_usage[] =
|
|
"git-write-tree [--missing-ok] [--prefix=<prefix>/]";
|
|
|
|
int write_tree(unsigned char *sha1, int missing_ok, const char *prefix)
|
|
{
|
|
int entries, was_valid, newfd;
|
|
|
|
/* We can't free this memory, it becomes part of a linked list parsed atexit() */
|
|
struct lock_file *lock_file = xcalloc(1, sizeof(struct lock_file));
|
|
|
|
newfd = hold_locked_index(lock_file, 1);
|
|
|
|
entries = read_cache();
|
|
if (entries < 0)
|
|
die("git-write-tree: error reading cache");
|
|
|
|
if (!active_cache_tree)
|
|
active_cache_tree = cache_tree();
|
|
|
|
was_valid = cache_tree_fully_valid(active_cache_tree);
|
|
|
|
if (!was_valid) {
|
|
if (cache_tree_update(active_cache_tree,
|
|
active_cache, active_nr,
|
|
missing_ok, 0) < 0)
|
|
die("git-write-tree: error building trees");
|
|
if (0 <= newfd) {
|
|
if (!write_cache(newfd, active_cache, active_nr)
|
|
&& !close(newfd))
|
|
commit_lock_file(lock_file);
|
|
}
|
|
/* Not being able to write is fine -- we are only interested
|
|
* in updating the cache-tree part, and if the next caller
|
|
* ends up using the old index with unupdated cache-tree part
|
|
* it misses the work we did here, but that is just a
|
|
* performance penalty and not a big deal.
|
|
*/
|
|
}
|
|
|
|
if (prefix) {
|
|
struct cache_tree *subtree =
|
|
cache_tree_find(active_cache_tree, prefix);
|
|
hashcpy(sha1, subtree->sha1);
|
|
}
|
|
else
|
|
hashcpy(sha1, active_cache_tree->sha1);
|
|
|
|
rollback_lock_file(lock_file);
|
|
|
|
return 0;
|
|
}
|
|
|
|
int cmd_write_tree(int argc, const char **argv, const char *unused_prefix)
|
|
{
|
|
int missing_ok = 0, ret;
|
|
const char *prefix = NULL;
|
|
unsigned char sha1[20];
|
|
|
|
while (1 < argc) {
|
|
const char *arg = argv[1];
|
|
if (!strcmp(arg, "--missing-ok"))
|
|
missing_ok = 1;
|
|
else if (!prefixcmp(arg, "--prefix="))
|
|
prefix = arg + 9;
|
|
else
|
|
usage(write_tree_usage);
|
|
argc--; argv++;
|
|
}
|
|
|
|
if (argc > 2)
|
|
die("too many options");
|
|
|
|
ret = write_tree(sha1, missing_ok, prefix);
|
|
printf("%s\n", sha1_to_hex(sha1));
|
|
|
|
return ret;
|
|
}
|